cors issue when using axios interceptors.
I am using axios for my api request and whenever I try to send the login request to API without using axios interceptors it work fine but when I add the interceptors it will have an cors error.
Here is my code with axios interceptor:
And I am trying to use this axios instance in login as below
Whenever I remove the interceptors code block it works without any issue but whenever I add it back it doesn't work. I have also tried to user login request as below:
But results in the same issue.
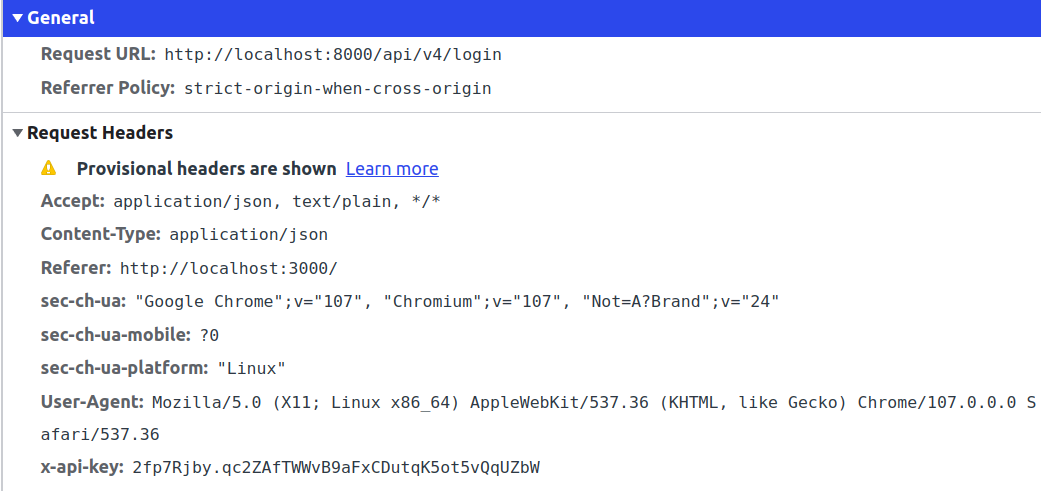
5 Replies
inland-turquoise•3y ago
When you remove the interceptors do you also remove
x-api-key
from the headers or do you append it as default or something? CORS might be triggered because Access-Control-Allow-Headers
on the server does not include x-api-key
🤔like-goldOP•3y ago
I am showing the alternative methods that I used without using the interceptors. Either I am using interceptors or the headers while request the axios call. Not both at the same time.
But both method doesn't work. And BTW
x-api-key
is included in the server headers because the backend expects the api key in headers.inland-turquoise•3y ago
Can you share the error if its logged in to the console?
like-goldOP•3y ago
You were right @aliemirs my server was not accepting
x-api-key
as a preflight headers. Thank you for you help.inland-turquoise•3y ago
Glad to hear issue is identified (and hopefully resolved) 🙏 Have a nice day! 👋