404 after logging in
Why does refine application show a 404 after signing in? I have a custom auth provider(keycloak.js) and this started happening after I injected it into refine application. As a framework I thought refine should not care what that auth provider does, whenever you hit a route like on screenshot refine should take control and redirect you if you're logged in. And it actually happened before when there was no keycloak auth provider. But now this happens. Also, as a matter of fact, I have a custom catchAll page(basically the one you see on screenshot) and it's code will be below. Should this behaviour somehow be moderated in catchAll component?
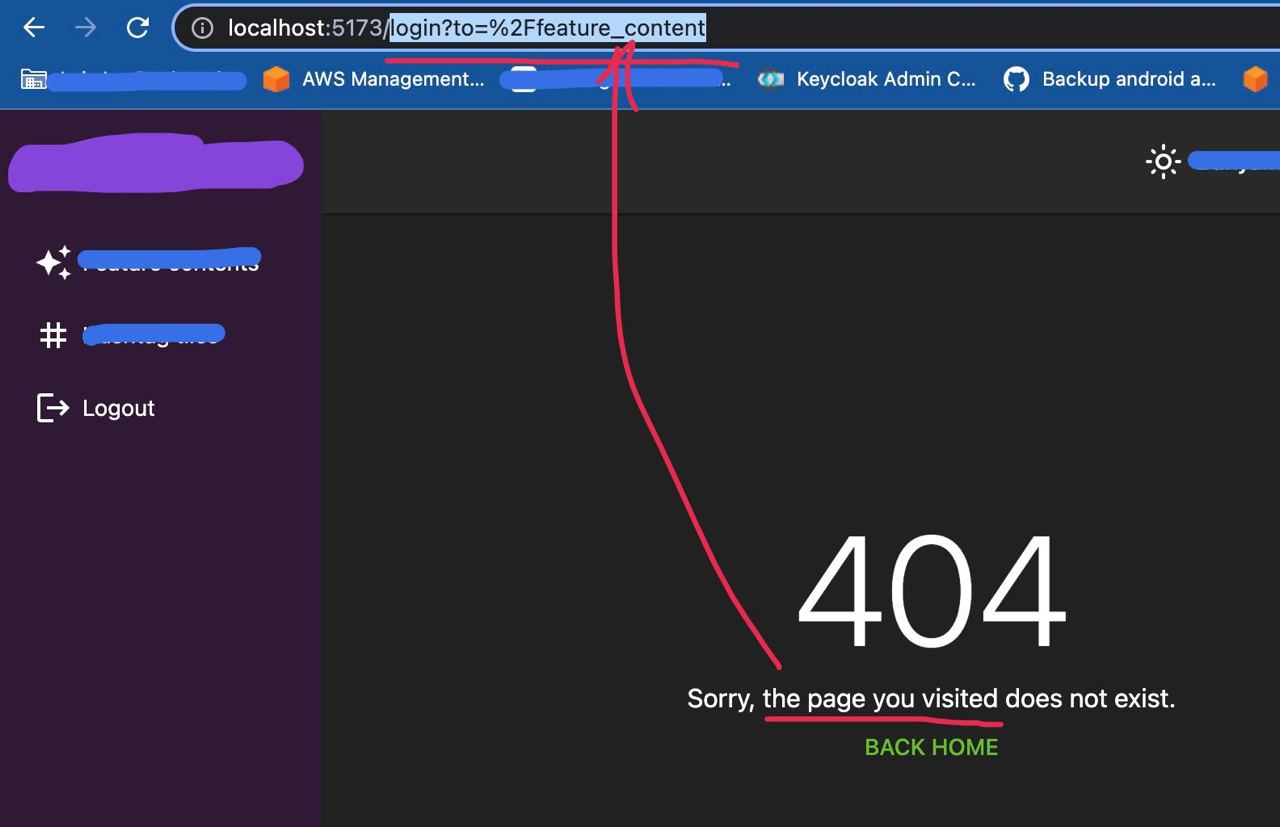
62 Replies
magic-amberOPβ’2y ago
The code for catchall component:
Hey @metammodern ,
This is not expected behavior. Can you share your AuthProvider?
magic-amberOPβ’2y ago
Sure, here is authProvider:
(if you know how to fold code blocks on discord let me know)
And here is UserService.ts
My guess is that Keycloak is running as async so when run checkAuth it returns Promise.reject for first load.
To avoid a similar situation in our Auth0 example, we wait for Auth0 to be init, https://github.com/refinedev/refine/blob/next/examples/auth-auth0/src/App.tsx#L23
Hey @_rassie ,
I hope you are very well. Maybe you can help us with this π
magic-amberOPβ’2y ago
@Omer I can log and check)
magic-amberOPβ’2y ago
@Omer I did it like this. The logs are the ones that ran only after redirect back from keycloak
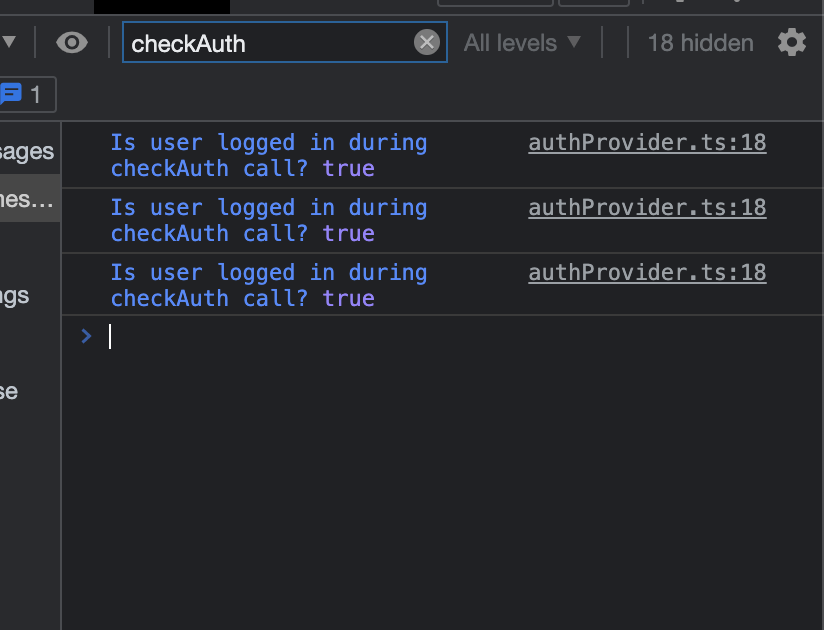
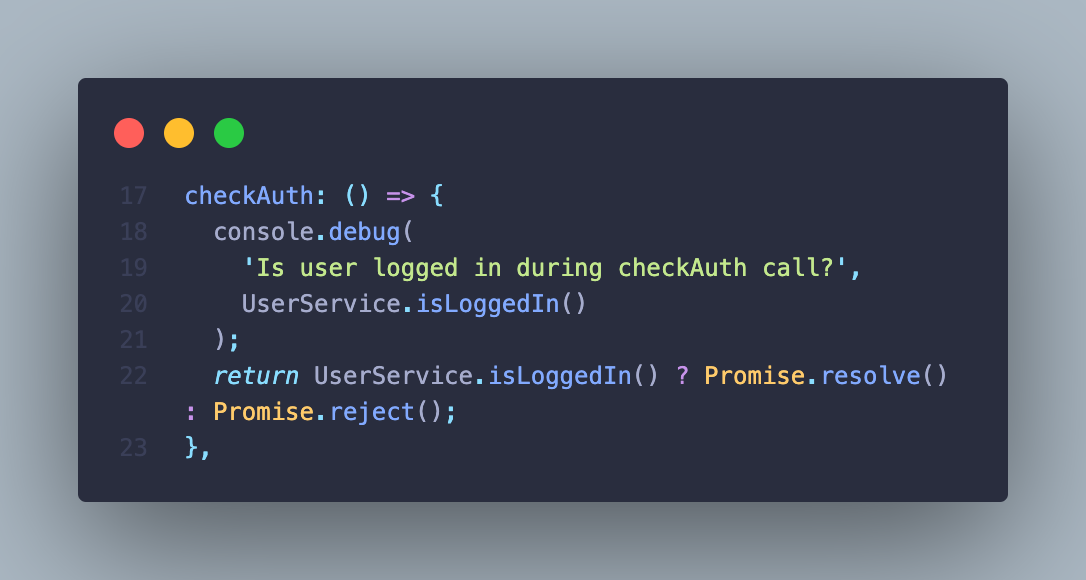
How can we reproduce this issue?
Also you can review Auth0 and Google Auth examples
https://refine.dev/docs/advanced-tutorials/auth/auth0/
https://refine.dev/docs/examples/auth-provider/google-auth/
Auth0 Login | refine
Auth0 is a flexible, drop-in solution for adding authentication and authorization services to your applications. Your team and organization can avoid the cost, time, and risk that comes with building your own solution to authenticate and authorize users. You can check the Auth0 document for details.
Google Auth | refine
You can use Google Login to control access and provide identity for your app. This example will guide you through how to connect Google Login into your project using refine.
magic-amberOPβ’2y ago
Hm, I guess I'll have to create a stackblitz for that, that should not be anyhow related to keycloak...
Hey @dontpanicaim ,
I hope you are very well. Maybe you can help us with this π
extended-salmonβ’2y ago
does the 404 go away with a manual page reload?
magic-amberOPβ’2y ago
@dontpanicaim hi, no it doesn't
extended-salmonβ’2y ago
@Omer thanks! im doing well! hope you are being awesome as normally too π
Glad to see you! Are you planning to join the hackathon? β‘οΈ
extended-salmonβ’2y ago
ooh is there a hackathon?
@metammodern hmm everything looks pretty good, do you know if
getUserIdentity
is being called?
I've been thinking of replace supabase with Hasura in the project I was working onThe refine Open Source Hackathon | refine
refine Hackathon is an excellent opportunity for developers to showcase their skills, learn refine and win prizes!
magic-amberOPβ’2y ago
let me check
extended-salmonβ’2y ago
and want to play with the new Inferencer
magic-amberOPβ’2y ago
@dontpanicaim yes it's called. And I see my user details in top right corner
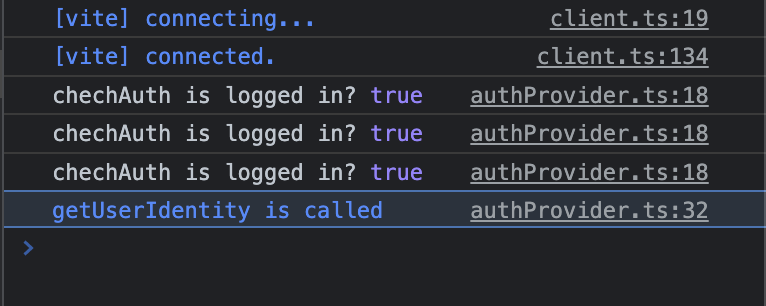
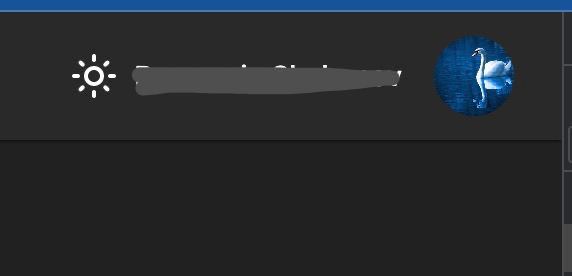
magic-amberOPβ’2y ago
@Omer thanks for the links, I used them as a reference when I was implementing this authProvider
congrats on transfering examples to codesandbox btw)
extended-salmonβ’2y ago
it feels like an async issue, like @Omer suggested
I started to see a lot of people using Hasura. They seem to be doing well π inferencer doesn't support GraphQL for now but @aliemirs will take care
extended-salmonβ’2y ago
awesome, looking forwarding in trying it out once it supported
I wish we had a keycloak example. Unfortunately, I never used it
extended-salmonβ’2y ago
@metammodern you can try making checkAuth async
I work a lot with keycloak, but didnt needed to make an auth provider since it comes with supabase
@metammodern ideally all methods in the auth provider are async
magic-amberOPβ’2y ago
mhm, I'll try, but for @Omer here's a tip/hint: you told that in Auth0 you had to add early loading return if auth0 was loading. In my case I don't need it. Check the UserService initKeycloak method, it accepts a callback. And that callback is app rendering in index.tsx file:
So I don't render anything until keycloak is initialized and ready to provide any reasonable info
Yes, you are right
magic-amberOPβ’2y ago
@dontpanicaim it didn't help. I am suspicios that something is in catchAll part
I see in example a prewritten antD ErrorComponent is used. Can you give me link to it?
I'll check how it's written
magic-amberOPβ’2y ago
found it https://github.com/refinedev/refine/blob/next/packages/antd/src/components/pages/error/index.tsx
GitHub
refine/index.tsx at next Β· refinedev/refine
Build your React-based CRUD applications, without constraints. - refine/index.tsx at next Β· refinedev/refine
It's here https://github.com/refinedev/refine/blob/next/packages/antd/src/components/pages/error/index.tsx
Also you can use swizzle command
GitHub
refine/index.tsx at next Β· refinedev/refine
Build your React-based CRUD applications, without constraints. - refine/index.tsx at next Β· refinedev/refine
magic-amberOPβ’2y ago
yes swizzle, I was thinking about "swoosh"π
wait what am I doing. Let me just remove catchAll and see what happens. Dumb...
nah, not the rootcause(
The problem is not with the error page. The problem is somehow checkAuth returns promise.reject after login
I guess the easiest way to solve the problem is to look at Auth0 and Google Auth examples
magic-amberOPβ’2y ago
So if I hardcode to return Promise.resolve() it will work you say?)
Yes
magic-amberOPβ’2y ago
Just checked, nope) didn't work)
Is it possible for you to share a video where we can see the issue?
magic-amberOPβ’2y ago
Of course!
magic-amberOPβ’2y ago
The only thing that I'm also suspicious is that hash in the end that's being added by keycloak. But it's being removed immideately(I gues by refine or by react-router)
Aha okay
If you always return promise.resolve() on the checkAuth the login screen will not appear. You can only see it in the unauthenticated state
Auth Provider | refine
refine let's you set authentication logic by providing the authProvider property to the `` component.
magic-amberOPβ’2y ago
I did a trick: I logged out, then went to sign in page of keycloak and during that I changed the code. When keycloak redirected back to app it already had "checkAuth" always returning Promise.resolve(). Does this count?)
I'll get back to this tomorrow
I will create a Keycloak example for you tomorrow β‘οΈ
magic-amberβ’2y ago
Oh, seems I'm late to the party π I'm afraid I can't contribute much because it's been some time since I last used keycloak.js (I'm firmly in the oauth2-proxy camp) and I also don't see an obvious error in the code. I'll follow the discussion though, maybe something will catch my eye.
magic-amberOPβ’2y ago
I wanted to check it but it's not in sandbox yet. I'll try locally though
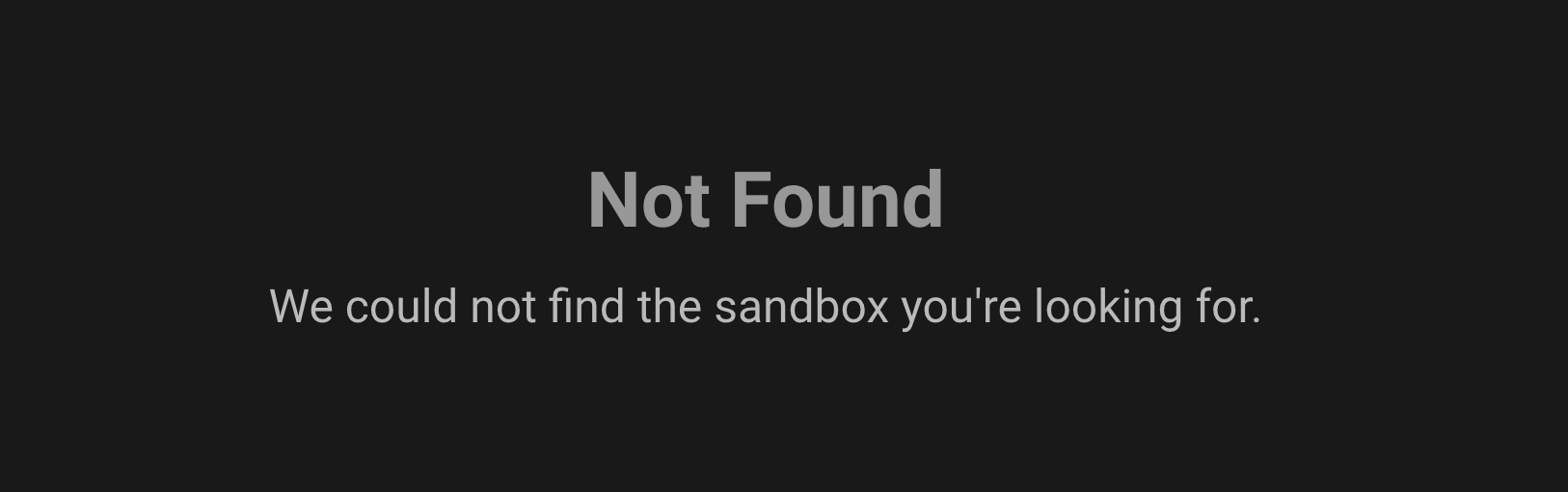
magic-amberOPβ’2y ago
@Omer aha! Got you!)
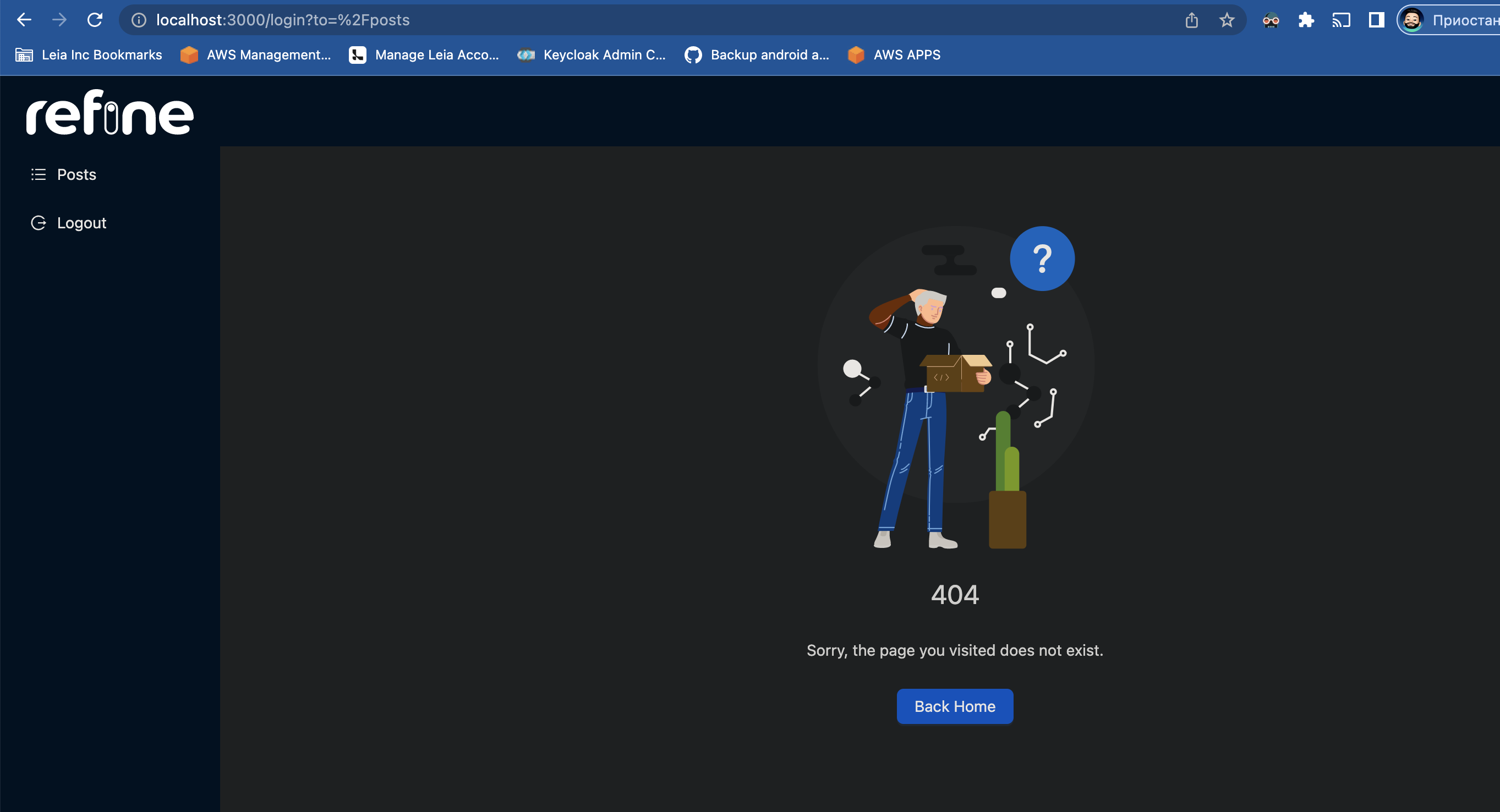
magic-amberOPβ’2y ago
So what I did: I logged out completely. Then Went to "localhost:3000/posts", it changed to "http://localhost:3000/login?to=%2Fposts" and opened a login Page. So far so good. Then I logged in, it redirected me back and showed me 404(screenshot above)
Could you check this line?
https://github.com/refinedev/refine/blob/master/examples/auth-keycloak/src/App.tsx#L34
GitHub
refine/App.tsx at master Β· refinedev/refine
Build your React-based CRUD applications, without constraints. - refine/App.tsx at master Β· refinedev/refine
magic-amberOPβ’2y ago
yep, i see. but it's logout, not login
and even if it was login--it's the default
How i can reproduce?
magic-amberOPβ’2y ago
I wrote instruction above but let me record a video will be clearer
magic-amberOPβ’2y ago
Aha I got it π
magic-amberOPβ’2y ago
awesome)
And thank you for keycloak example, I was thinking about adding it myself since you have keycloak in providers diagram on npm) Now we have source to refer to in our internal docs)
I have a temporary solution to this,
magic-amberOPβ’2y ago
Looks good enough, I'll apply it) Should I maybe create an issue so that core team digs into rootcauses of this?
It would be great π₯
magic-amberOPβ’2y ago
Ok, I'll get back to this in a while when I apply this fix locally. Thank you so much @Omer ! Honestly did not expect much of involvment from a CTO/CEO but seems you really like being activeπ π
Hi, @Omer ! The issue is now resolved with your fix. When logging in I don't have a 404 anymore.
However, if I implicitely go to "http://localhost:5173/login?to=%2Ffeature_content" the issue still remains, I still see 404. Probably an issue with router at this point and not with keycloak or smth.
What do you think?
π₯Ί
extended-salmonβ’2y ago
Hey @metammodern can you share the code for the related routes, i think we can resolve this with some quick tweaks
magic-amberOPβ’2y ago
@aliemirs hello:) I use the default router provider:
import routerProvider from '@pankod/refine-react-router-v6';
in App.tsx
And then I created resources according to docs. and also I have auth provider matching the docs examplesextended-salmonβ’2y ago
Can you share the /login route and its wrapper route?
magic-amberOPβ’2y ago
And after that I open then link provided above. First it requests authorization, I authorize(sign in), it comes back and resource list page opens(Again, this started working after Omer's fix applied).
But if I open the same link (login?to=/resource_name) it gives 404. So the issue is not in authProvider
Umm, let me see if I even have such thing
I have a pages/login.tsx file. Is that what you want?
Other than that, I don't have any explicit "/login" route defined anywhere
If you want(and if you can) we may jump on a call I can screenshare then and show exact issue. If we resovle it this way I will write the solution here so it won't be lost