How to retrieve a list of elements
Hello, I don't quite understand how refine works. I would like to retrieve the list of all my exercises (available on my api via GET: /exercises), the problem is that refine always adds end and start parameters to the query, I don't want that.
What's more, I'd like to know, on a page that's neither List Show Edit or Create, a way of retrieving all this, what should I use? useOne? useMany?
I don't know, I'm just starting out.
Merci !
14 Replies
Hi @realdragonma. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
Hello! To fetch the list of all your exercises without the
end
and start
parameters, you can create a custom data provider method. In this custom method, you can make a request to your API without those parameters.
To fetch the data on a page that's neither List, Show, Edit, or Create, you can use the useList
data hook. This hook is suitable for fetching multiple records from a resource.
Here's an example of how to use the useList
hook:
This code snippet will fetch all the exercises from your API. You can find more information about the useList
hook in the useList documentation (https://refine.dev/docs/api-reference/core/hooks/data/useList/).
I hope this helps you get started with refine! If you have any more questions, feel free to ask.
----------------------
Note: you can ask me a follow up question by @ mentioning me again
:speech_balloon:
----------------------<@214840332943097856> kapa.ai is still learning and improving, please let us how it did by reacting below
foreign-sapphireOP•2y ago
It works fine, but why does the request always add "?_end=10&_start=0&" at the end? I don't want that.
manual-pink•2y ago
Because your requests send via the
dataProvider
you defined. If you want to change the default behavior of the dataProvider
, you can create a custom dataProvider
and use it in your project or override the default dataProvider
methods.
You can find more information here: https://refine.dev/docs/tutorial/understanding-dataprovider/index/foreign-sapphireOP•2y ago
Thank you, it works! The only problem is with the types now: here's what I'm doing:
but I get an error on the data.data: "TS2339: Property 'sessions' does not exist on type 'BaseRecord[]'."
Is it possible not to return this BaseRecord[], but an any? Here's my dataProvider :
manual-pink•2y ago
You can use generics of the
useList
like below:
foreign-sapphireOP•2y ago
Thank you for your response, but I'm sorry, the error persists: TS2339: Property 'sessions' does not exist on type 'ISession[]'.
data?.data is equal:
So this is why I want that .sessions
My sessions is accessible via "data.data.sessions"
manual-pink•2y ago
Could you share your usage of
useList
hook?foreign-sapphireOP•2y ago
I have no usage yet, I just want to see how it works, but the idea is to selection a sessions :
I just need to correct this type error
Here is the value of data :
foreign-sapphireOP•2y ago
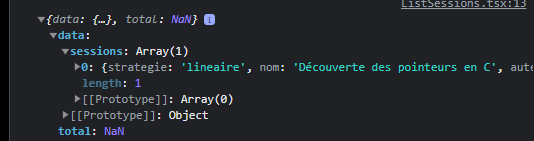
manual-pink•2y ago
When you give a generic type, you don't need to define type again like below:
Because,
data?.data.sessions
already will have ISession[]
typeforeign-sapphireOP•2y ago
So is there any way to access directly data.data.sessions ? and not data ?
manual-pink•2y ago
You should customize your
dataProvider
according to your API response:
What you return here with data
should be an array.
Currently, you are returning an object. So, you can refactor it like below:
foreign-sapphireOP•2y ago
Thanks !