Display list object in Select
Hi ! How can i show my clients key in the Select component (which is a list of object of type {id: string, config: {}), and i want to show the name by default in the Select component:
here is a exemple of result of the resource "session" with a given id :
As you can see, i want to show in the select all clients id
12 Replies
Hi @realdragonma. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
To show the clients' ids in the Select component, you can use the
useSelect
hook from Refine. First, you need to import the useSelect
hook and modify your SessionsEdit
component to utilize it. Here's an example of how to do that:
In this example, we're using the useSelect
hook to fetch the clients' data and set the optionLabel
to "id"
to display the clients' ids in the Select component. The options
returned by the useSelect
hook are then passed to the Select
component.
Please note that the provided code snippets are based on the information extracts and may require some adjustments to fit your specific use case.
----------------------
Note: you can ask me a follow up question by @ mentioning me again
:speech_balloon:
----------------------<@214840332943097856> kapa.ai is still learning and improving, please let us how it did by reacting below
deep-jadeOP•2y ago
@kapa.ai You seem to not understand that I want the Select prefilled by the "clients" present in the queryResults of the useForm.
Here is what i want to edit :
as you may see, "clients" is a list objet of type {id: string, config: any}[]
So in this example, i would like to have "laweb" and "cas" prefilled in the Select
Let me check the knowledge base for you. One moment! :books:
----------------------
I apologize for the misunderstanding. To prefill the Select component with the clients' ids from the
queryResult
, you can use the queryResult
data and map it to the Select component's value. Here's how you can do that:
In this example, we're using the useState
and useEffect
hooks from React. We initialize a state called selectedClients
to store the selected clients' ids. When the queryResult
data is available, we update the selectedClients
state with the clients' ids from the data. Then, we set the value
prop of the Select component to selectedClients
and handle the onChange
event to update the selectedClients
state when the user makes changes to the selection.
Please note that the provided code snippets are based on the information extracts and may require some adjustments to fit your specific use case.
----------------------
Note: you can ask me a follow up question by @ mentioning me again
:speech_balloon:
----------------------<@214840332943097856> kapa.ai is still learning and improving, please let us how it did by reacting below
yappiest-sapphire•2y ago
You can use Form.Item component from antd package. The ‘name’ prop of the component may help you.
deep-jadeOP•2y ago
I already tried a lot of solution, even with "name", I never find a issue for my case
yappiest-sapphire•2y ago
In the example code, you need to remove options because the Form.Item pass the
value
and onChange
to its children.
I don't see any other errordeep-jadeOP•2y ago
As you may see here, my clients (I put you a input because we can see the error in details) are show as [objet Object]. And i dont want that.
Here is an example of the data i want to show :
My "clients" key is a list object (type:
{id: string, config: {}}[]
So in my Select, with this example, I just want "laweb" (in this example) and not [object Object]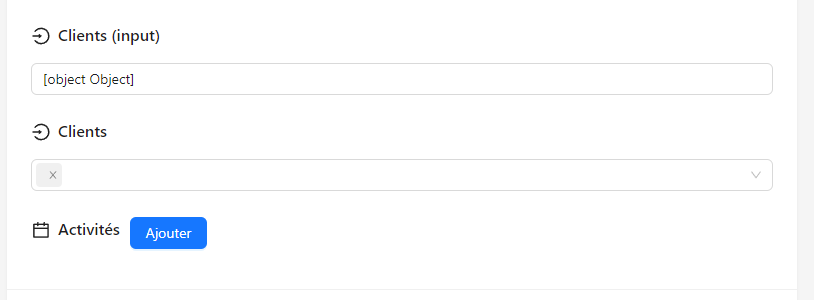
deep-jadeOP•2y ago
Here is my code :
Sorry if I'm not making myself clear, i'm french, my english may not be good and clear
@kapa.ai Do you have any suggestion with taking in note what we said ?
Let me check the knowledge base for you. One moment! :books:
----------------------
Based on our previous discussion, you can prefill the Select component with the clients' ids from the
queryResult
by using the queryResult
data and mapping it to the Select component's value. Here's how you can do that:
In this example, we're using the useState
and useEffect
hooks from React. We initialize a state called selectedClients
to store the selected clients' ids. When the queryResult
data is available, we update the selectedClients
state with the clients' ids from the data. Then, we set the value
prop of the Select component to selectedClients
and handle the onChange
event to update the selectedClients
state when the user makes changes to the selection.
Please note that the provided code snippets are based on the information extracts and may require some adjustments to fit your specific use case.
----------------------
Note: you can ask me a follow up question by @ mentioning me again
:speech_balloon:
----------------------<@214840332943097856> kapa.ai is still learning and improving, please let us how it did by reacting below