authProvider onError Only Called on Final Error
Hi!
I have an authentication token for my API that expires after a certain amount of time.
Once it does, the application receives an error when it tries to fetch data (as expected).
I have authProvider's onError set up so that when it receives a 401, it logs the user out and redirects them to the login page.
However, onError is only called after multiple failures.
I'm wondering if I can log the user out via an Axios interceptor rather than authProvider's onError?
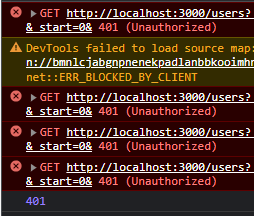
20 Replies
Hi @avangeous. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
Based on the information provided in the extracts, it's clear that the
onError
method in the authProvider
is designed to handle error responses from the API, including logging out the user and redirecting them to a specific page. However, the extracts do not provide any information about using an Axios interceptor for this purpose.
The onError
method is called when an error response is received from the API. If the error status code is 401
(indicating an expired token), the user is logged out and redirected to the login page. This is achieved by returning an object with logout: true
and redirectTo: "/login"
from the onError
method.
(Source: refine.dev (https://refine.dev/docs/tutorial/understanding-authprovider/create-authprovider))
However, the extracts do not provide any information on how to implement this functionality using an Axios interceptor. Therefore, based on the information available, it's not possible to provide a confident answer to your question.
For more information on how to handle errors in refine, you can refer to the useOnError documentation (https://refine.dev/docs/api-reference/core/hooks/authentication/useOnError/).
----------------------
Note: you can ask me a follow up question by @ mentioning me again
:speech_balloon:
----------------------<@1122927158348763206> kapa.ai is still learning and improving, please let us how it did by reacting below
exotic-emerald•14mo ago
@kapa.ai How can I log a user out?
Let me check the knowledge base for you. One moment! :books:
----------------------
In Refine, you can log out a user by using the
logout
method provided by the authProvider
. This method can be called with the useLogout
hook.
Here's an example of how you can create a custom logout button using the useLogout
hook:
In this example, mutate
acquired from the useLogout
can accept any kind of object for values since the logout
method from the authProvider
doesn't have a restriction on its parameters.
For more information, you can refer to the useLogout documentation (https://refine.dev/docs/api-reference/core/hooks/authentication/useLogout) and authProvider documentation (https://refine.dev/docs/api-reference/core/providers/auth-provider).
----------------------
Note: you can ask me a follow up question by @ mentioning me again
:speech_balloon:
----------------------<@1122927158348763206> kapa.ai is still learning and improving, please let us how it did by reacting below
exotic-emerald•14mo ago
@kapa.ai How can I log a user out outside of a React component?
Let me check the knowledge base for you. One moment! :books:
----------------------
Logging out a user outside of a React component in Refine is not directly covered in the provided extracts. However, the
useLogout
hook, which is used to log out a user, is a React hook and is designed to be used inside a React component.
Here's an example of how you can use the useLogout
hook inside a React component:
(Source: refine.dev (https://refine.dev/docs/api-reference/core/hooks/authentication/useLogout))
If you need to log out a user outside of a React component, you might need to directly call the logout
method from your authProvider
. However, the specifics of how to do this are not covered in the provided extracts.
For more information, you can refer to the useLogout documentation (https://refine.dev/docs/api-reference/core/hooks/authentication/useLogout) and authProvider documentation (https://refine.dev/docs/api-reference/core/providers/auth-provider).
----------------------
Note: you can ask me a follow up question by @ mentioning me again
:speech_balloon:
----------------------<@1122927158348763206> kapa.ai is still learning and improving, please let us how it did by reacting below
exotic-emerald•14mo ago
Handling a logout-on-error on the axiosInstance side theoretically seems to work, but given its outside of a React component I'm stumped as to how I can log the user out from here.
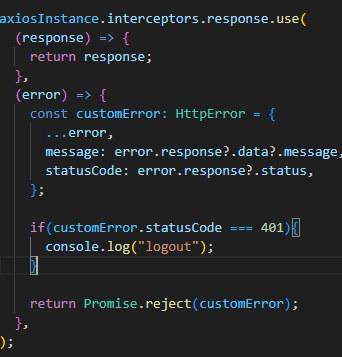
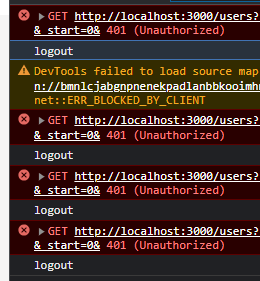
foreign-sapphire•14mo ago
Hi! I'm having the same problem, did you manage to resolve it?
In my case onError is not called never
exotic-emerald•14mo ago
I do have it called, are you still having that issue?
foreign-sapphire•14mo ago
Hey! I solved it, thanks. It works like in your case, the onError is calles after like 3 or 4 sequencing error responses
exotic-emerald•14mo ago
Lol weird. I haven't had the chance to look into why, feels like it shouldn't happen.
harsh-harlequin•14mo ago
hello, because by default TanStack query retries 3 times when request is failed
exotic-emerald•13mo ago
Is there any workaround to prevent the retry?