How can I handle a 401 unauthorized response to logout the user from the app?
I'm asking if refine has a way to handle when you have a 401 response in your dataProvider, and make the app run the logout method of the AuthProvider or if I have to handle it in the axios instance.
I'm asking this because I dont want the check function of the AuthProvider validating the jwt token, because that would imply that I have to save the JWT secret in a env variable in the client, and that is not secure.
Thanks!
31 Replies
Hi @jaoc1811. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
Yes, Refine provides a way to handle 401 responses in your dataProvider and trigger the logout method of the AuthProvider. You can achieve this by implementing the
onError
method in your AuthProvider. This method is called when you get an error response from the API.
Here is an example of how you can implement the onError
method to log out the user if the API returns a 401 error:
In this example, if the API returns a 401 or 403 error, the onError
method will return an object with logout: true
and redirectTo: "/login"
. This will cause the useOnError
hook to call the logout
method and redirect the user to the login page.
You can also use the useOnError
hook's mutation to handle the error of a fetch request:
In this example, if the fetch request fails, the mutate
method of the useOnError
hook is called with the error as an argument. This will trigger the onError
method of the AuthProvider.
For more information, refer to the useOnError
documentation→ (https://refine.dev/docs/api-reference/core/hooks/authentication/useOnError/)
Regarding your concern about storing the JWT secret in an environment variable on the client side, you're correct that this is not secure. The JWT secret should be kept on the server side and never exposed to the client. The client should only store the JWT token, not the secret.
----------------------
Note: you can ask me a follow up question by @ mentioning me again
:speech_balloon:
----------------------<@110864105035808768> kapa.ai is still learning and improving, please let us know how it did by reacting below
sunny-greenOP•2y ago
@kapa.ai I'm trying with the onError function, but i'm getting 401 responses and the function is not running
@kapa.ai
Let me check the knowledge base for you. One moment! :books:
Sorry @jaoc1811, I seem to be having a bit of trouble. Don't worry though, I've pinged my supprt and they will try to fix me up! :technologist:
Perhaps try again in 15mins?
sunny-greenOP•2y ago
@kapa.ai I'm trying with the onError function, but i'm getting 401 responses and the function is not running
Let me check the knowledge base for you. One moment! :books:
----------------------
The
onError
function should be triggered when a 401 response is received. If it's not working as expected, there might be an issue with how it's implemented or how the error is being handled in your dataProvider.
Here's a basic example of how the onError
function should be implemented in your authProvider
:
In this example, if the API returns a 401 or 403 error, the onError
method will return an object with logout: true
and redirectTo: "/login"
. This will cause the useOnError
hook to call the logout
method and redirect the user to the login page.
If you're still having issues, you might want to check how you're handling errors in your dataProvider. The error object that's passed to the onError
method should contain a status
property with the HTTP status code of the error response.
For more information, refer to the onError
documentation→ (https://refine.dev/docs/api-reference/core/providers/auth-provider) and the useOnError
documentation→ (https://refine.dev/docs/api-reference/core/hooks/authentication/useOnError/).
----------------------
Note: you can ask me a follow up question by @ mentioning me again
:speech_balloon:
----------------------<@110864105035808768> kapa.ai is still learning and improving, please let us know how it did by reacting below
sunny-greenOP•2y ago
@kapa.ai do i have to use the useOnError? o the onError should be executed automatically?
Let me check the knowledge base for you. One moment! :books:
----------------------
The
onError
method in your authProvider
should be executed automatically when an error response is received from the API. You don't necessarily need to use the useOnError
hook for this to happen.
The useOnError
hook is used to manually trigger the onError
method from your authProvider
. It's useful when you want to handle errors in a specific way in certain parts of your application. For example, you might want to use the useOnError
hook to handle errors from a fetch request:
In this example, if the fetch request fails, the mutate
method of the useOnError
hook is called with the error as an argument. This will trigger the onError
method of the AuthProvider.
However, for general error handling (like handling 401 responses), you should be able to rely on the onError
method in your authProvider
.
For more information, refer to the onError
documentation→ (https://refine.dev/docs/api-reference/core/providers/auth-provider) and the useOnError
documentation→ (https://refine.dev/docs/api-reference/core/hooks/authentication/useOnError/).
----------------------
Note: you can ask me a follow up question by @ mentioning me again
:speech_balloon:
----------------------<@110864105035808768> kapa.ai is still learning and improving, please let us know how it did by reacting below
sunny-greenOP•2y ago
@kapa.ai can you give me a error response example? because the onError function is not running
Let me check the knowledge base for you. One moment! :books:
----------------------
The
onError
function in your authProvider
should be triggered when an error response is received from the API. The error object that's passed to the onError
method should contain a status
property with the HTTP status code of the error response.
Here's an example of how an error response might look:
In this case, if your onError
method is implemented like this:
Then the onError
method should be triggered when a 401 or 403 error response is received, and it should return an object with logout: true
and redirectTo: "/login"
.
If the onError
method is not being triggered as expected, there might be an issue with how the error is being handled in your dataProvider. Make sure that the error object that's being passed to the onError
method contains a status
property with the HTTP status code of the error response.
For more information, refer to the onError
documentation→ (https://refine.dev/docs/api-reference/core/providers/auth-provider) and the useOnError
documentation→ (https://refine.dev/docs/api-reference/core/hooks/authentication/useOnError/).
----------------------
Note: you can ask me a follow up question by @ mentioning me again
:speech_balloon:
----------------------<@110864105035808768> kapa.ai is still learning and improving, please let us know how it did by reacting below
sunny-greenOP•2y ago
nope, onError is not been called
flat-fuchsia•2y ago
I am having the same issue, were you able to solve it?
I am storing my access token on local storage and then validating the JWT using a library that connects to my user pool / client id (using AWS cognito), so it should be secure, but I cannot get my logout function to run after the onError returns the
logout: true
sunny-greenOP•2y ago
Hi!
the onError function is not running?
flat-fuchsia•2y ago
my onError function is running fine, and it determines that the JWT token is expired, and then it returns this:
But my logout function, like this:
Never gets ran
sunny-greenOP•2y ago
oh, I see
What was happening to me was that the onError function was never running, and I found out that it wasn't running because the component was autogenerated by infencerer, after I put my own code in the component the onError function was working fine.
It is really strange that your logout function is not running, that is the exactly purpose of the logout field in the return of the onError
flat-fuchsia•2y ago
oh yea that makes sense, I am not using the inferencer in my case, just built a simple page to list items
yea im not sure if I am supposed to use that
useOnError
hook or something, that would be weird considering this is supposed to simplify all that. Also, then I would need to use that hook for every possible component, which doesn't seem rightsunny-greenOP•2y ago
I should not be necessary to use the hook. Actually I just tried and my onError function runs the logout function fine
sunny-greenOP•2y ago
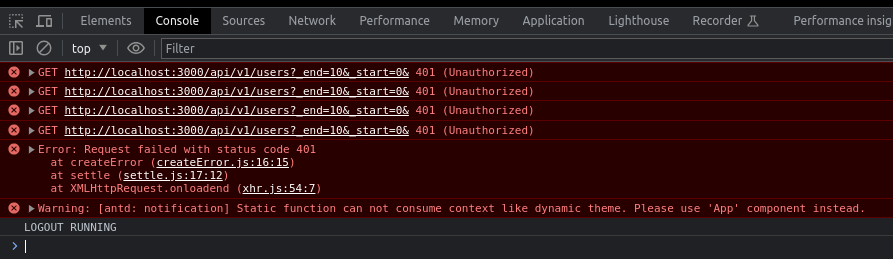
sunny-greenOP•2y ago
are you sure that the onError function is running?
oh wait, I didn't check it right. Let me check again
Yes, I checked it again, and it works
flat-fuchsia•2y ago
Yes this is my console log, it indentifies the token is expired, and then returns the logout, but never actually logs out
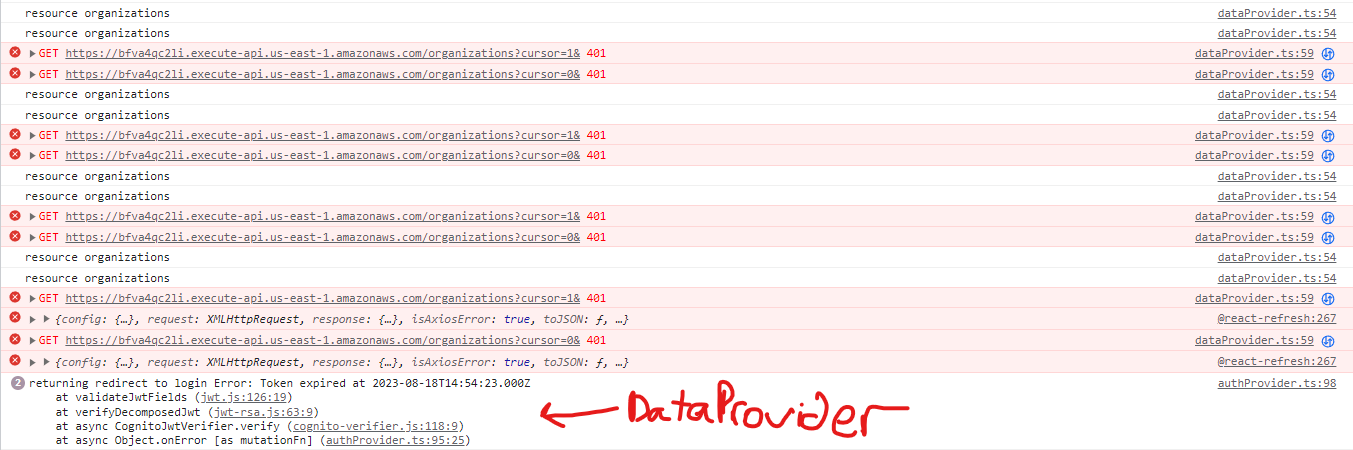
sunny-greenOP•2y ago
these are my onError and logout functions
flat-fuchsia•2y ago
hmm, i suppose the only difference I have is I have my logic in a try/catch block, is that stopping the return too early?
nvm it should return from the function
sunny-greenOP•2y ago
maybe those return { error } are the problem?
because that "error" hasn't the onErrorResponse structure
flat-fuchsia•2y ago
well all of those attributes for the return error are optional,. For all other errors I just pass the error because I don't know how to handle them yet, but for an expired token it's returning :
In my case where the token is expired
sunny-greenOP•2y ago
wow, I'm trying to see what could be wrong but I can't find anything
ok, try this
take out the finally statement from the try catch
and try
I understand that when you have a finally statement in a try catch, the return in the try or catch is saved temporally but the finally's overrides them
so maybe you're returning always { error } instead of the complete json
flat-fuchsia•2y ago
Brilliant! That fixed it! I totally forgot about that lol, thank you!
sunny-greenOP•2y ago
Nice!
flat-fuchsia•2y ago
i was treating that as a default similar to a switch/case