Why does my List page generate so many queries?
This is my code for my list page (listing organizations):
And my
getList
is defined in my dataProvider
by:
Yea, as shown in the screenshot, I am pushing out like 10 GET requests when the organizations page loads, I only have the list page for now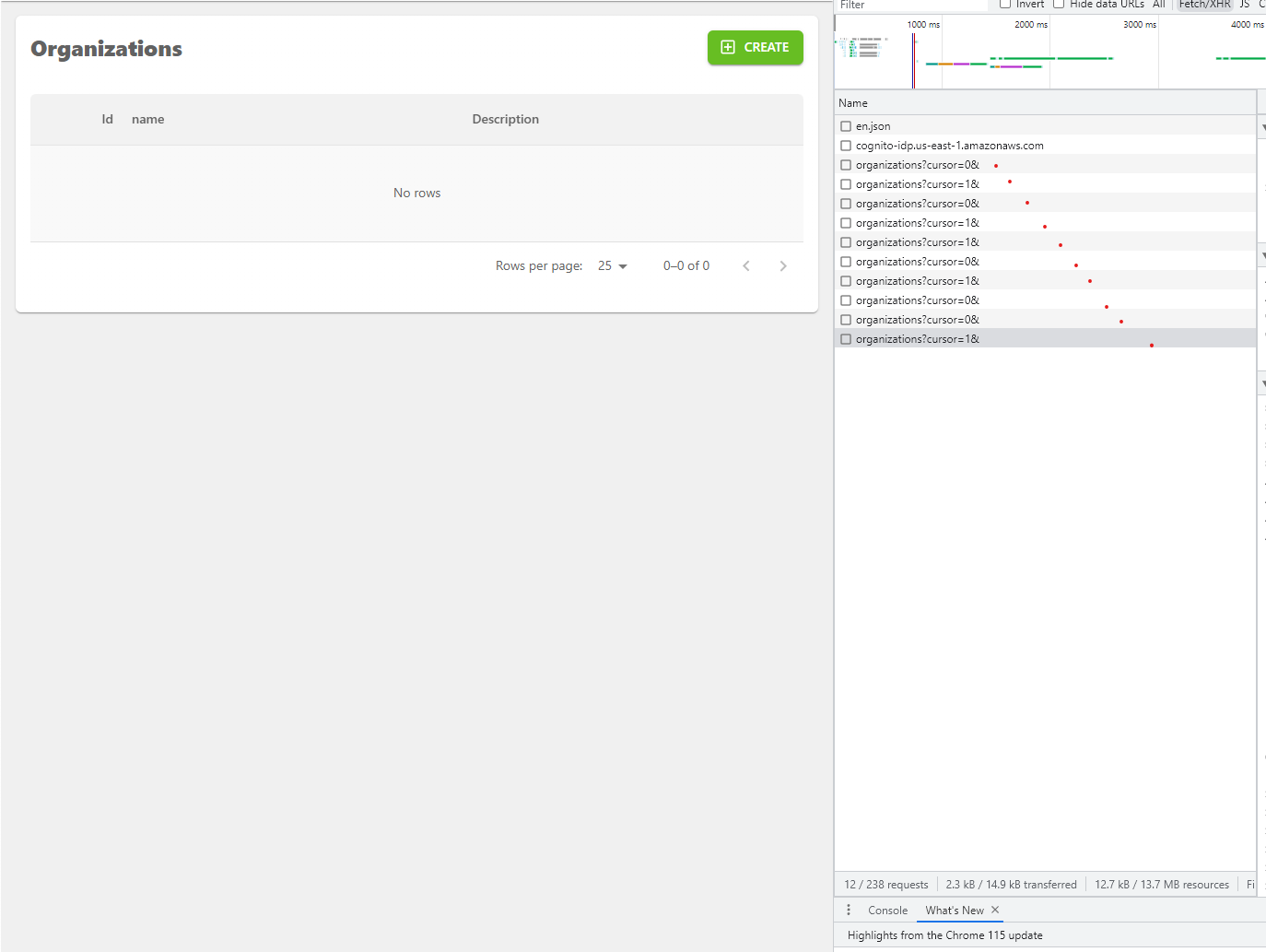
13 Replies
Hi @nick_montoya. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
It seems like you're experiencing an issue with multiple GET requests being sent when your organizations page loads. This could be due to the way your
useInfiniteList
hook is set up.
In your useInfiniteList
hook, you have queryOptions: { enabled: !!dataGridProps?.rows }
. This means that the query will be enabled if dataGridProps?.rows
is truthy. If dataGridProps?.rows
changes frequently, it could trigger multiple requests.
One way to mitigate this is to ensure that dataGridProps?.rows
does not change frequently or unnecessarily. You could also consider using a different condition to enable the query.
If you're still experiencing issues, you might want to check the refine documentation (https://refine.dev/docs) or the refine blog (https://refine.dev/blog) for more information and examples on how to use the useInfiniteList
and useDataGrid
hooks.
----------------------
Note: you can ask me a follow up question by @ mentioning me again
:speech_balloon:
----------------------<@263527875506995202> kapa.ai is still learning and improving, please let us know how it did by reacting below
grumpy-cyan•13mo ago
@kapa.ai does refine implement a retry function? because my data table is currently empty, so is it trying to keep querying a certain amount of times before it says there are none?
Let me check the knowledge base for you. One moment! :books:
----------------------
Yes, refine does implement a retry function. This is done through the
queryOptions
and mutationOptions
properties in various hooks.
For instance, in the useTable
hook, you can pass queryOptions
to control the behavior of the query. Here's an example:
In this example, if the query fails, it will be retried 3 times before finally failing. This is useful in scenarios where temporary network issues might cause a query to fail.
Similarly, in the useForm
hook, you can pass updateMutationOptions
or createMutationOptions
to control the behavior of the mutations. Here's an example:
In this example, if the update mutation fails, it will be retried 3 times before finally failing.
These options are powered by the underlying data fetching library that refine uses, which is react-query. You can learn more about these options in the react-query documentation (https://tanstack.com/query/v4/docs/react/reference/useQuery).
Sources:
- useTable documentation (https://refine.dev/docs/api-reference/core/hooks/useTable)
- useForm documentation (https://refine.dev/docs/api-reference/core/hooks/useForm)
----------------------
Note: you can ask me a follow up question by @ mentioning me again
:speech_balloon:
----------------------<@263527875506995202> kapa.ai is still learning and improving, please let us know how it did by reacting below
inland-turquoise•13mo ago
Hey @nick_montoya, this is interesting, sorry for the issue! 🙏 I'll check the code you've provided and try to reproduce and identify the issue.
Apart from the provided code, do you have any calls for the
fetchNextPage
?grumpy-cyan•13mo ago
Hi Aliemir,
Thanks!
I don't use
fetchNextPage
anywhere, in fact I removed it completely, but it sill does it.inland-turquoise•13mo ago
Thank you for the answer, btw about the retries, the default retry count is 3 but it only retries after an error, in your case there's no retries
grumpy-cyan•13mo ago
oh i see right, so in that case it shouldn't rety at all
inland-turquoise•13mo ago
Judging by the network calls you've shared, it looks like there's an issue with the pagination of the
useInfiniteList
. Have you tried useDataGrid
? Does it have the same issue or just when using the infinite list hook?grumpy-cyan•13mo ago
Sorry I am new to refine and MUI, does
useDataGrid
extend the same functionality of useInifinteList
?
I was trying to go off the examples online, and overall I thought i had to use useInfitineList
and then I would pass that data to my DataGrid
, but is there a better way to do this?
This is my code:
inland-turquoise•13mo ago
Hey @nick_montoya, sorry for the late response in this issue.
Just checked out the code you've shared above. It looks like you've mixed up the
useDataGrid
and useInfiniteList
. This might be the one messing up with the pagination and triggering multiple calls to the getList
.
You don't need to use both of them. We provided useDataGrid
to make it easier to integrate with the DataGrid
component.
It uses the useTable
hook from the @refinedev/core
under the hood but provides some extra props for the <DataGrid>
.
If you use useInfiniteList
which is an extended version of the useList
hook but with infinite query support, you don't need the useDataGrid
hook, you can use the data from useInfiniteList
and configure the pagination stuff manually with <DataGrid>
.grumpy-cyan•13mo ago
Ooooo that makes sense, I changed to just using dataGridProps, and now it works fine!