using the react-i18next example I guess a type mismatch
Hey everyone, I just started integrating translations into our application and noticed that the documentation is a little bit off. Here (https://refine.dev/docs/api-reference/core/providers/i18n-provider/#creating-the-i18n-provider) in the docs it says that we should do the following:
But this leads to a type mismatch between the I18nProdiver (from @refinedev/core) and the
t
function from react-i18next:
I guess I'm not the only one getting that error. Maybe there is a common work-around already?7 Replies
Hi @bastianwegge. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
The error you're encountering is due to TypeScript's strict type checking. The
t
function from react-i18next
can return a string
or a TFunctionDetailedResult<string>
, but the translate
method in the I18nProvider
type from @refinedev/core
expects a function that returns only a string
.
To resolve this, you can add a type assertion to ensure that the t
function always returns a string
. Here's how you can modify your i18nProvider
:
This tells TypeScript to treat the result of t(key, options)
as a string
, which should resolve the type mismatch error.
Please note that this workaround assumes that your translations will always return a string
. If your translations can return complex objects (which would be the case if you're using react-i18next
's TFunctionDetailedResult
), you might need to adjust your translate
function accordingly.
For more information, you can refer to the Refine i18nProvider documentation (https://refine.dev/docs/api-reference/core/providers/i18n-provider) and the react-i18next documentation (https://react.i18next.com).
----------------------
Note: you can ask me a follow up question by @ mentioning me again
:speech_balloon:
----------------------<@317433576721219584> kapa.ai is still learning and improving, please let us know how it did by reacting below
correct-apricot•13mo ago
Hey @bastianwegge,
I checked the our i18n-react(https://refine.dev/docs/examples/i18n/i18n-react/) example and it works fine without any missing type errors. i18n-react' translate function has overloads for both
(key, options, defaultMsg)
and (key, defaultMsg)
.React | refine
refine i18n Provider allows you to add mutiple language choices to your web application. The default language of refine is English. You can include and use your own translation in the project for different language preferences. This example shows how to use different language options and translations in practice.
other-emerald•13mo ago
Hey @salihozdemir ! Thanks for taking time for this.
I was refering to your documentation on react-i18next, not i18n-react. Yes sure, a different library has different implementations that might fit to the requested type, but the one from react-i18next - as mentioned in your docs - isn‘t.
Bot was right, adjusting the return „as string“ does what I wanted but is a workaround nevertheless.
correct-apricot•13mo ago
Hey @bastianwegge,
Sorry for the misunderstanding. By default, I thought that the t function of the useTranslation hook returns string. When I look at the react-i18next documentation again, I see that it returns string (you can look here https://react.i18next.com/latest/usetranslation-hook). I wonder when it returns an array? Likewise, when I look at our examples, I don't see a type error 🤔
other-emerald•13mo ago
I just noticed that i18next and the react adapter is written in JavaScript with TypeScript types being added afterwords.
I also tracked down the types to the following two type definitions. It seems that based on the existence of the key inside of the translations, the t function might return another type. In any case, the workaround is fine for us!
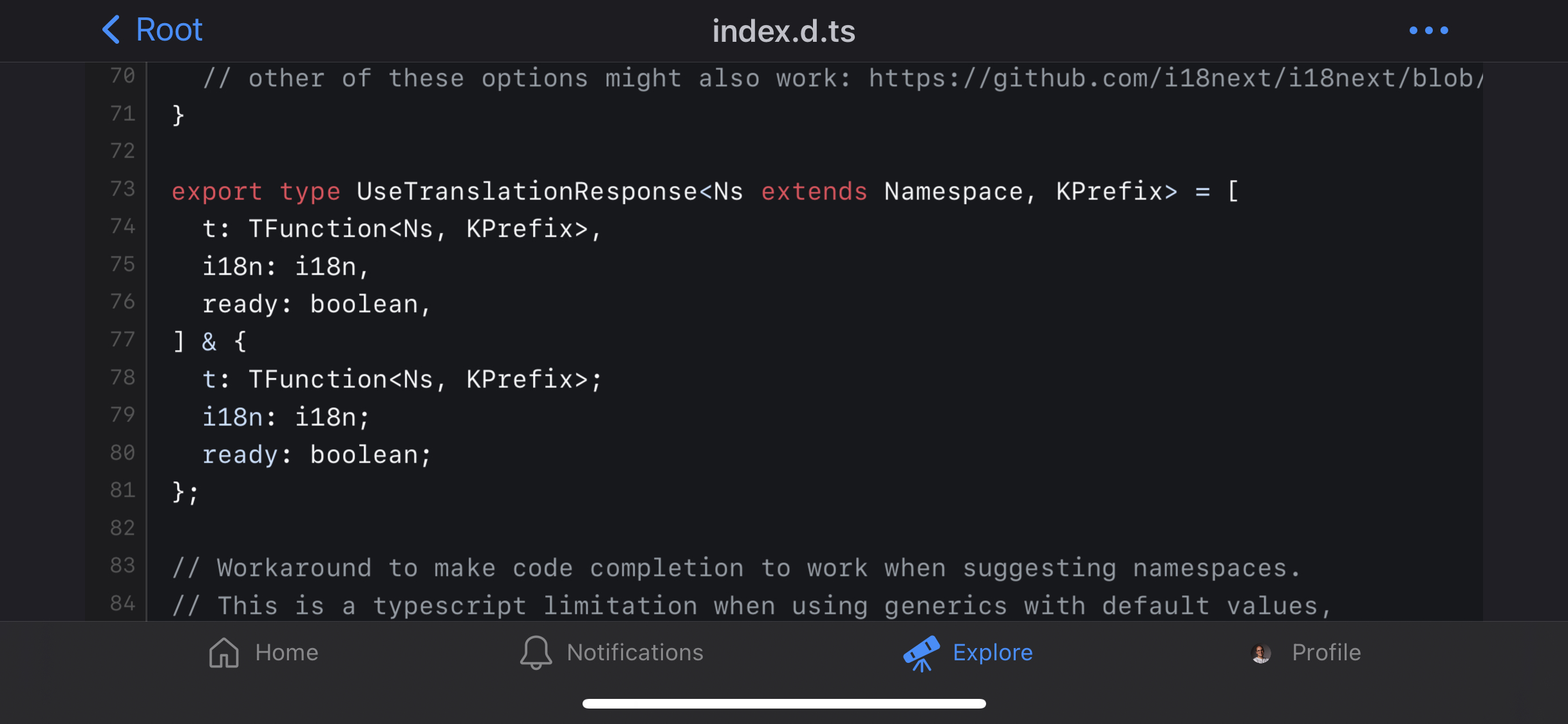
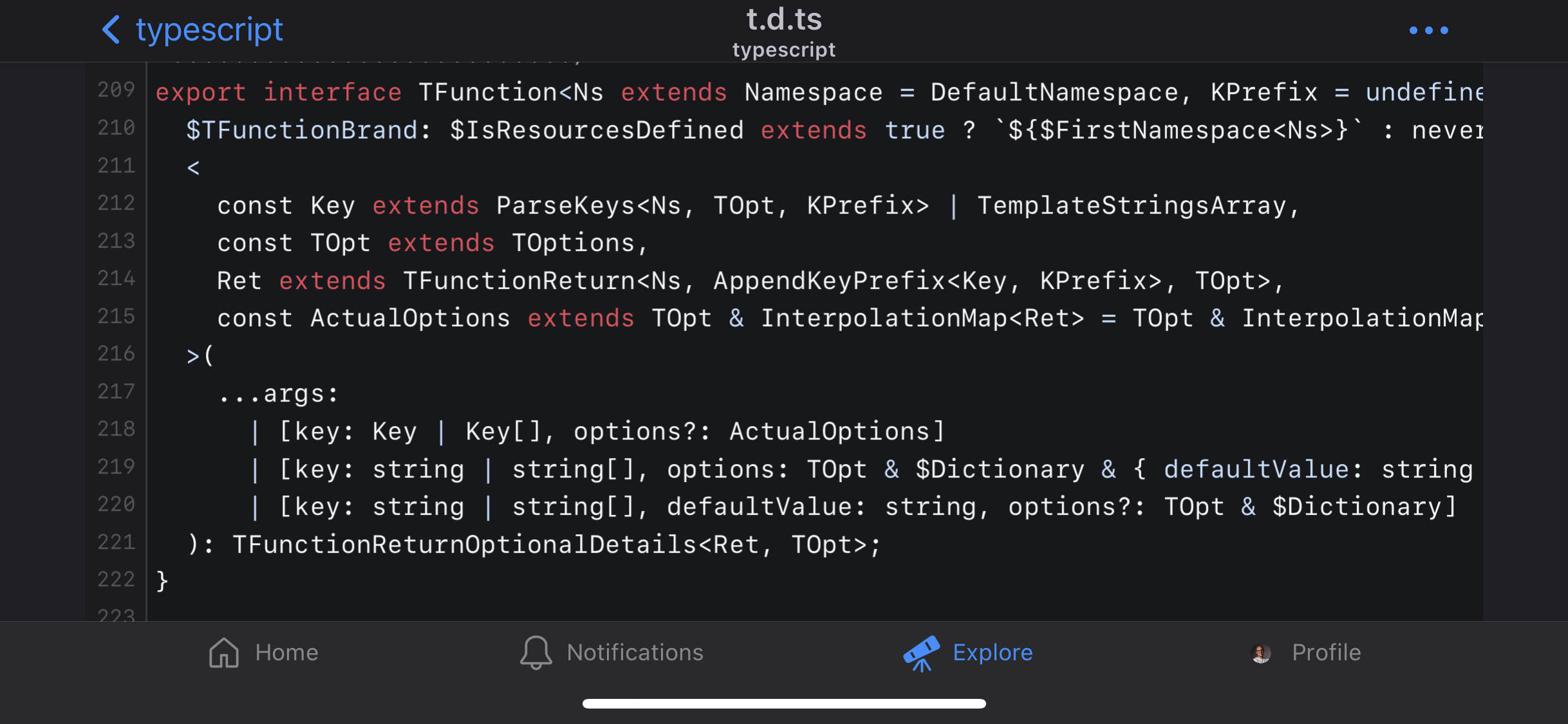
correct-apricot•13mo ago
Good to hear that the workaround is fine for you. Thanks for your feedback! 🙏