handle axios console error coming from useCreate
``import { HttpError } from "@refinedev/core";
import axios, { AxiosRequestConfig } from "axios";
export const TOKEN_KEY = "***";
const axiosInstance = axios.create({
baseURL: "http://127.0.0.1:5000/api",
});
axiosInstance.interceptors.request.use(
(request: AxiosRequestConfig) => {
// Retrieve the token from local storage
const token = localStorage.getItem(TOKEN_KEY);
// Check if the header property exists
if (request.headers) {
// Set the Authorization header if it exists
request.headers["Authorization"] = `Bearer ${token}`;
} else {
// Create the headers property if it does not exist
request.headers = {
Authorization: `Bearer ${token}`,
};
}
return request;
},
(error) => {
// Do something with request error
return Promise.reject(error);
}
);
axiosInstance.interceptors.response.use(
(response) => {
return response;
},
(error) => {
const customError: HttpError = {
...error,
message: error.response?.data?.message,
statusCode: error.response?.status,
};
return Promise.reject(customError);
}
);
export { axiosInstance };
how do i handle the console error thrown probably useCreate or axios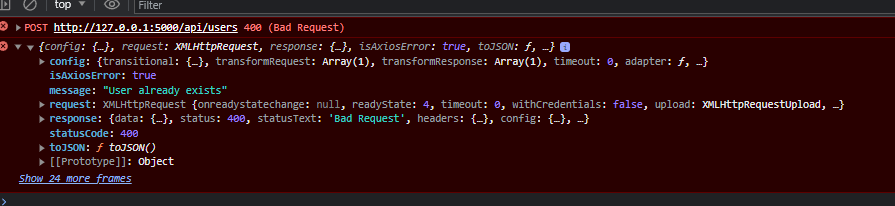
9 Replies
Hi @tarang._. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
In refine, you can handle errors thrown by
useCreate
or axios
by extending the error from HttpError
and using axios
interceptor to transform the error from the response before axios
returns the response to your code.
Here is an example of how to handle errors in your data provider:
(Source: refine.dev docs (https://refine.dev/docs/api-reference/core/providers/data-provider/))
Also, refine uses useOnError
internally in the data hooks to handle errors in a unified way. When an error is thrown by any data hook, the useOnError
function is triggered with the error object. Afterward, the error object is passed to the onError
method of the authProvider
, which can be utilized to redirect the user or to log them out.
(Source: refine.dev docs (https://refine.dev/docs/api-reference/core/hooks/authentication/useOnError/))
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@852840146495340564> kapa.ai is still learning and improving, please let us know how it did by reacting below
flat-fuchsia•12mo ago
@kapa.ai not helpful i have correct implementation of axios but i need to get rid or able to handle console errors
Let me check the knowledge base for you. One moment! :books:
----------------------
To handle console errors in refine, you can use the
onError
method in the authProvider
. This method is triggered when an error is thrown by any data hook. You can use this method to log the error or perform any other action. Here is an example:
(Source: refine.dev docs (https://refine.dev/docs/api-reference/core/hooks/authentication/useOnError/))
In addition, you can customize the error notification that shows up when the data fetching fails using the errorNotification
prop in the useCreate
hook:
(Source: refine.dev docs (https://refine.dev/docs/api-reference/core/hooks/data/useCreate/))
Also, you can handle errors in the onMutationError
callback function in the useForm
hook:
(Source: refine.dev docs (https://refine.dev/docs/api-reference/antd/hooks/form/useForm/))
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@852840146495340564> kapa.ai is still learning and improving, please let us know how it did by reacting below
flat-fuchsia•12mo ago
@kapa.ai can you ask assitance from support team?
Let me check the knowledge base for you. One moment! :books:
----------------------
Yes, you can definitely ask for better assistance from the refine support team. Here are some ways you can reach out to them:
- Apply for the Priority support program: You can apply to the priority support program and receive assistance from the refine core team in your private channel. Apply here (https://s.refine.dev/slack)
- Join the Discord community: It is the easiest way to get help and ask questions to the community. Join here (https://discord.gg/refine)
- Join the GitHub Discussions: You can ask anything about the refine project or give feedback; the team would love to hear your thoughts! Join the discussion here (https://github.com/refinedev/refine/discussions)
(Source: refine.dev docs (https://refine.dev/docs/))
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
<@852840146495340564> kapa.ai is still learning and improving, please let us know how it did by reacting below
sensitive-blue•12mo ago
when you get network errors. like (400 Bad request). chrome always prints on console
if you want to handle thrown errors from
useCreate
, you can handle from dataProvider.create method