Unable to modify login error message
In this documentation https://refine.dev/docs/api-reference/core/components/auth-page/#login
Under authProvider we can pass error object when login fails, what's this object for? Since it doesn't seem to be modifying the error message you get from the message component if the login details are invalid.
How would I go about modifying the login error message? (I know I could create a custom login component, but if there's a way to just change the error message text, it would keep things simpler). Thank you
| refine
component from refine is an authentication page that can be used to login, register, forgot password, and update password.
11 Replies
Hi @thats_rex. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
In refine, the error object you pass when the login fails is used to customize the error message that is displayed. Refine automatically displays an error notification when the
login
method resolves the promise with success: false
. If you want to customize this error message, you can resolve the promise with an error
object that has name
and message
properties.
Here is an example of how you can do this:
In this example, the name
property is set to "Login Failed!" and the message
property is set to "The email or password that you've entered doesn't match any account." When the login fails, this custom error message will be displayed.
For more information, you can refer to the refine documentation (https://refine.dev/docs/api-reference/core/providers/auth-provider/).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@339473145541165069> kapa.ai is still learning and improving, please let us know how it did by reacting below
rival-black•12mo ago
The above solution is what I tried and it didn't seem to modify the error message.
Hey @thats_rex ,
If you are using headless, you need to add a notification provider. You can add it to your project in simple steps by following this document: https://refine.dev/docs/api-reference/core/providers/notification-provider/#creating-a-notificationprovider-from-scratch. To add an empty notification provider, you can use the command
npm run refine add notification
.
If you are not using the latest version of@refinedev/cli
, please update it using this command:npm i @refinedev/cli@latest
.
rival-black•12mo ago
Never mind, I apologize for this question without proper context. I am not using headless.
Figured out that I wasn't handling the error properly. Realized that it was giving the generic error message since I hadn't wrapped the API in try and catch (in the authProvider), in the catch block I passed the return object with custom message and name, it's working now.
Thank you for the help either way.
Every problem solved makes me happy. Have fun!! 🍻
BTW, have you applied for the beta of refine devtool? https://twitter.com/refine_dev/status/1704600640994029690
refine (YC S23) (@refine_dev)
🌟 Hey folks! We've been hearing the first whispers from the users diving into our refine devtools beta, and guess what? They are loving it! 🛠️
Your toolset could be the next to level up - we're still welcoming new adventurers. Come, be a part of this journey!
Twitter
rival-black•12mo ago
I am relatively new to Refine, as you can see. Once I get a bit more familiar, I'll be sure to check this out :) I will also need to ask my teammates if we can apply for the beta.
flat-fuchsia•8mo ago
how to change this "AxiosError" label?

flat-fuchsia•8mo ago
this is my auth-provider.ts
Can I change the "AxiosError" label on line 2 notification?
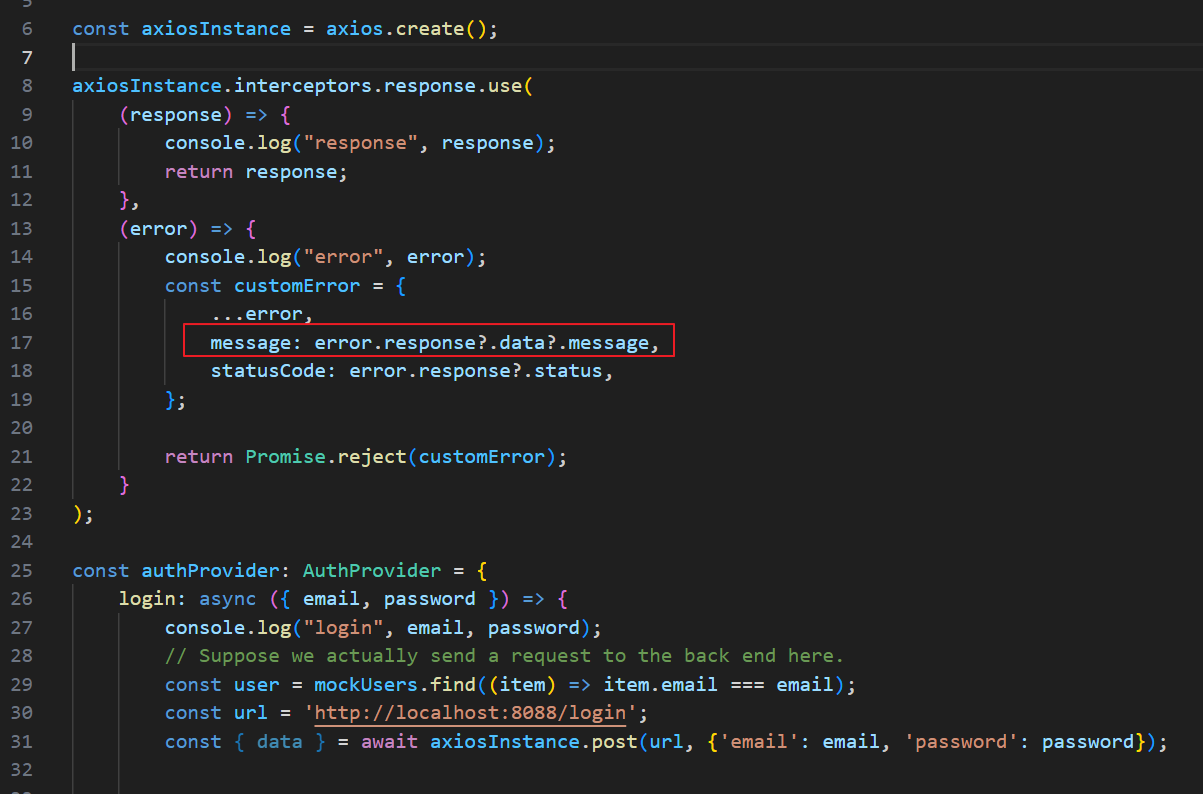
flat-fuchsia•8mo ago
I've found the solution: