How do I add a dropdown to the login page?
I know there is
formProps
in the AuthPage
component, but I'm not sure how to use it with MUI Select component. On the login page, I want to add a select component that lets the user select their role (whether they are an admin, student, or teacher)7 Replies
Hi @soto76. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
The
formProps
prop in the AuthPage
component is used to pass props to the form component. This includes changing the initialValues
and the onSubmit
function. However, the extracts provided do not contain specific information on how to use formProps
with the MUI Select component.
That being said, you can use the MUI Select component within the form in the AuthPage
component. The MUI Select component can be used for a wide range of purposes on a website, including a form component.
Here's an example of how to use the MUI Select component in a form:
In this example, the Select
component is used to create a dropdown menu where the user can select their role. The Option
component is used to create the options in the dropdown menu. The value
state is used to keep track of the selected option.
Please note that this is a general example and might need adjustments based on your specific use case. For more detailed information, you can refer to the refine documentation (https://refine.dev/docs) and blogs (https://refine.dev/blog).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@934748406096736296> kapa.ai is still learning and improving, please let us know how it did by reacting below
xenial-black•11mo ago
@kapa.ai how do I use MUI Select component within the form in the AuthPage component?
Let me check the knowledge base for you. One moment! :books:
----------------------
Based on the information provided in the extracts, the
AuthPage
component in Refine allows you to customize the content of the form using the renderContent
prop. However, the extracts do not provide a specific example of using the MUI Select component within the AuthPage
form.
Here's a general idea of how you might use the renderContent
prop to include a MUI Select component in the form:
In this example, the renderContent
prop is used to render a custom form content. The Select
component from MUI is used to create a dropdown menu where the user can select their role. The MenuItem
component is used to create the options in the dropdown menu.
Please note that this is a general example and might need adjustments based on your specific use case. For more detailed information, you can refer to the refine documentation (https://refine.dev/docs) and blogs (https://refine.dev/blog).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@934748406096736296> kapa.ai is still learning and improving, please let us know how it did by reacting below
firm-tan•10mo ago
Hello @soto76 you can
swizzle
auth page for your custom needs: https://refine.dev/docs/api-reference/mui/components/mui-auth-page/| refine
component from refine is an authentication page that can be used to login, register, forgot password, and update password.
xenial-black•10mo ago
@batuhanw thanks! this worked, but for some reason when I added another login variable "role" to the login function, I am getting the error
role does not exist in type LoginFormTypes
. But I already added "role" to the LoginFormTypes
and triple saved the file, and it still didn't work. My workaround was creating another interface that is exactly like LoginFormTypes
and then using that interface for the useLogin
hook. It works, but I feel like i'm missing something...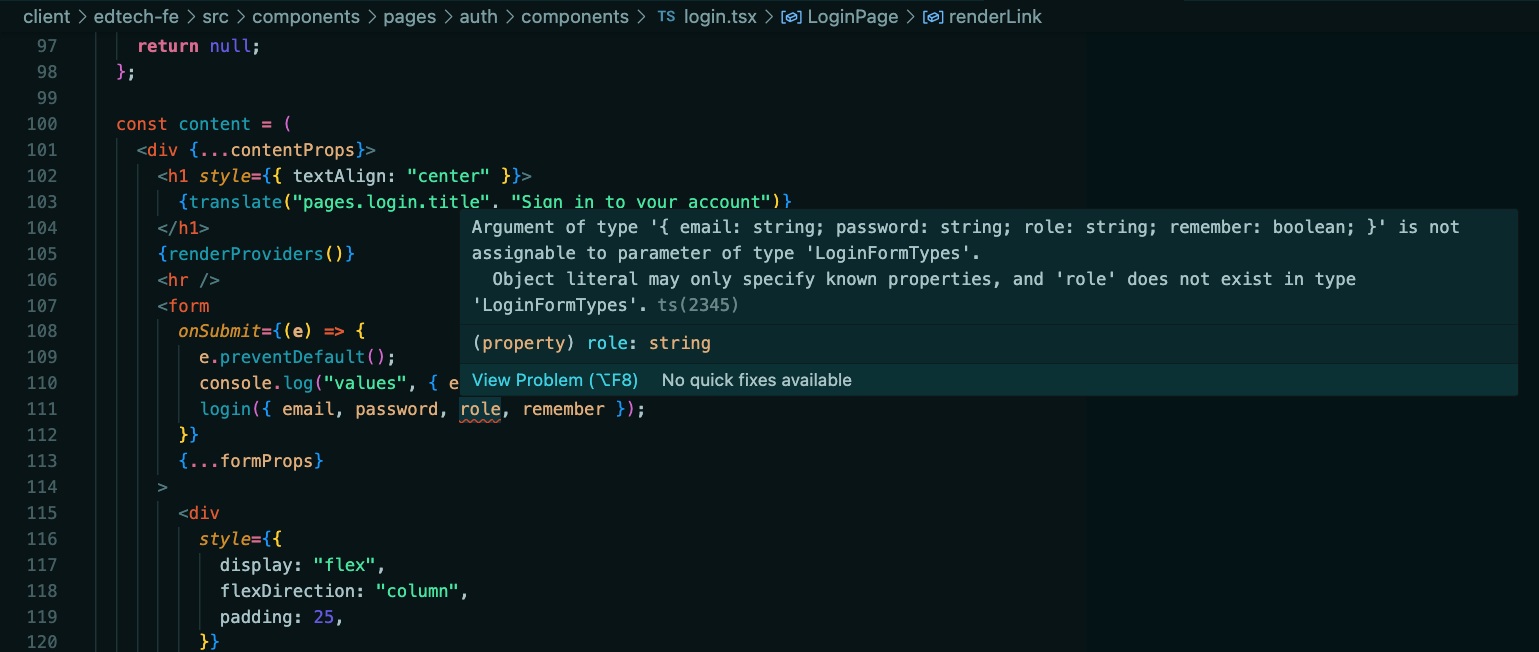
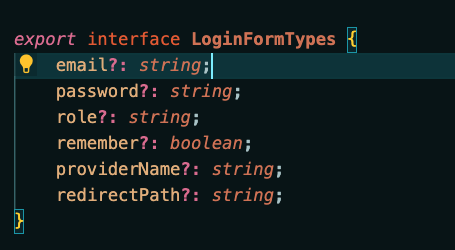