Mui textfield with react hook form register
I have a textfield with type date ,i have registers the field with react hook form register, but iam getting an error
The specified value "2023-12-02T00:00:00.000Z" does not conform to the required format, "yyyy-MM-dd".
the date came from backend , and the field sometime show date sometime not .iam using this same field with type text, password, time and date
12 Replies
Hi @MarcoNix. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
The issue you're facing is due to the format of the date returned by the backend. The date value '2023-12-02T00:00:00.000Z' is in ISO 8601 format, but the TextField of type 'date' expects the date in 'yyyy-MM-dd' format.
To resolve this, you can use a date library like
Day.js
to format the date. Day.js
is used by refine to display date format. Here's how you can modify your code:
In this example, dayjs(start_date).format('YYYY-MM-DD')
is used to format the date value to 'yyyy-MM-dd' format which is expected by the TextField of type 'date'.
Please note that this is a general example and might need to be adjusted based on your specific use case and data structure. For more information, you can refer to the refine documentation (https://refine.dev/docs/api-reference/mui/components/fields/date).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@942824607818317905> kapa.ai is still learning and improving, please let us know how it did by reacting below
ambitious-aqua•10mo ago
backend is sending 2023-12-02T00:00:00.000Z this format ,when it register in that format ,it shows the error ,can we set a default value transformer for this,without onchange
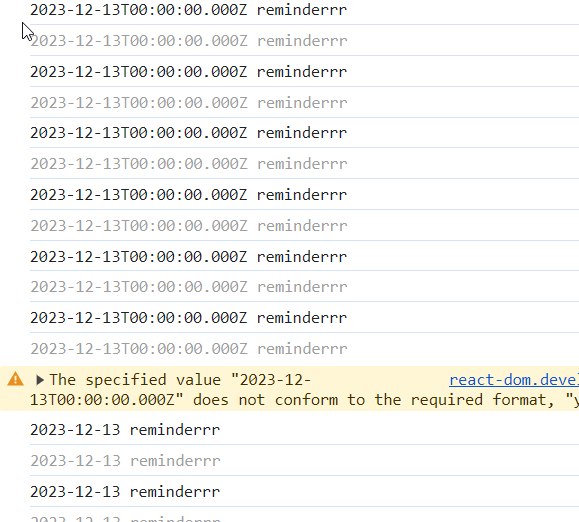
ambitious-aqua•10mo ago
@kapa.ai i want to transform the date to the before register add the value
Let me check the knowledge base for you. One moment! :books:
----------------------
If you want to transform the date before registering it to the form, you can do so in the
useEffect
hook where you set the form values. Here's how you can modify your code:
In this example, dayjs(post.date).format('YYYY-MM-DD')
is used to transform the date to 'yyyy-MM-dd' format before setting it to the form value with setValue("date", formattedDate)
.
Please note that this is a general example and might need to be adjusted based on your specific use case and data structure. For more information, you can refer to the refine documentation (https://refine.dev/docs/api-reference/mui/components/fields/date).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@942824607818317905> kapa.ai is still learning and improving, please let us know how it did by reacting below
ambitious-aqua•10mo ago
@kapa.ai is there somethin in useform to transform date initialy
Let me check the knowledge base for you. One moment! :books:
----------------------
Yes, you can transform the date initially using the
useForm
hook. However, the transformation is not done directly in the useForm
hook. Instead, you would typically use a useEffect
hook to set the initial form values after transforming the date. Here's an example:
In this example, dayjs(post.date).format('YYYY-MM-DD')
is used to transform the date to 'yyyy-MM-dd' format before setting it to the form value with setValue("date", formattedDate)
.
Please note that this is a general example and might need to be adjusted based on your specific use case and data structure. For more information, you can refer to the refine documentation (https://refine.dev/docs/api-reference/mui/components/fields/date).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@942824607818317905> kapa.ai is still learning and improving, please let us know how it did by reacting below
ambitious-aqua•10mo ago
@kapa.ai can we do transformation inside register hook options
Let me check the knowledge base for you. One moment! :books:
----------------------
The
register
function from the useForm
hook in Refine doesn't directly support transformation of values. It's primarily used to register input/select Ref (callback ref) into the custom hook.
However, you can handle the transformation before setting the value to the input field. If you're using a controlled component, you can transform the value before passing it to the value
prop of your input field.
Here's an example:
In this example, dayjs(dateOfBirth).format('YYYY-MM-DD')
is used to transform the date to 'yyyy-MM-dd' format before setting it to the input field.
Please note that this is a general example and might need to be adjusted based on your specific use case and data structure. For more information, you can refer to the refine documentation (https://refine.dev/docs/api-reference/mui/components/fields/date).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@942824607818317905> kapa.ai is still learning and improving, please let us know how it did by reacting below