useDrawerForm
I am opening the modal with the following path: inquiry/edit/88/authority-create. When I close the modal the path will be inquiry/edit/88 and the application calls the api edit:id. How do I close the modal without calling the api
13 Replies
Hi @hoaanhtuc. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
The
useDrawerForm
hook in Refine provides a close
function that you can use to manually close the drawer. This function does not automatically call any API when used. However, if you have set up your application to call an API when the drawer is closed, you might need to adjust your code to prevent this behavior.
Here's an example of how you can use the close
function:
In this example, the close
function is passed to the onClick
prop of the Cancel button. When this button is clicked, the drawer will close without making any API calls.
If you want to prevent the drawer from closing after form submission, you can handle it in your onFinish
handler. Here's an example:
In this code, the onFinishHandler
function is passed to the onFinish
prop of the Form
component. This function is called when the form is successfully validated and submitted. The close
function is not called in onFinishHandler
, so the modal will not close after form submission.
Please note that these examples are based on the information provided in the extracts and may need to be adjusted based on your specific use case.
Sources:
- useDrawerForm Return Values (https://refine.dev/docs/ui-integrations/mantine/hooks/use-drawer-form/#return-values)
- Discord support thread: save and continue (https://discord.com/channels/837692625737613362/1165992447659212912)
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@617879699363725341> kapa.ai is still learning and improving, please let us know how it did by reacting below
rival-blackOP•2y ago
@kapa.ai http://localhost:5173/inquiry/edit/88/authority-create
How can I avoid calling the detailed api when accessing http://localhost:5173/inquiry/edit/88?
Let me check the knowledge base for you. One moment! :books:
----------------------
Based on the information provided in the extracts, it seems like your application is set up to call a detailed API when accessing the URL
<http://localhost:5173/inquiry/edit/88
.> This behavior is likely defined in your routing configuration or in a useEffect hook that triggers when the URL changes.
To avoid calling the detailed API when accessing this URL, you would need to adjust your routing configuration or the useEffect hook to exclude this specific URL.
Unfortunately, the extracts do not provide specific code examples or details about how your application is set up, so I can't provide a more detailed answer. However, you can refer to the React Router documentation (https://reactrouter.com/) or the React documentation on useEffect (https://reactjs.org/docs/hooks-effect.html) for more information on how to control when API calls are made based on the URL.
If you're using Refine's useNavigation
hook to navigate between pages, you might be able to adjust the push
function to avoid making the API call. Here's an example:
In this example, the second argument to the push
function is false
, which means that the navigation will not trigger a new API call. Please note that this is a hypothetical example and may not work in your specific case.
Sources:
- useNavigation (https://refine.dev/docs/api-reference/core/hooks/navigation/usenavigation/)
- React Router (https://reactrouter.com/)
- React useEffect (https://reactjs.org/docs/hooks-effect.html)
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@617879699363725341> kapa.ai is still learning and improving, please let us know how it did by reacting below
rival-blackOP•2y ago
@kapa.ai Can you apply it with useDrawerForm?
Let me check the knowledge base for you. One moment! :books:
----------------------
The
useDrawerForm
hook in Refine does not directly control the routing or API calls in your application. It is primarily used to manage the state and behavior of forms within a drawer component.
However, you can use it in combination with other hooks or components in your application to control when and how API calls are made. For example, you could use the useNavigation
hook to navigate to a new URL without making an API call when the drawer is closed.
Here's a hypothetical example:
In this example, the handleClose
function is passed to the onClose
prop of the Drawer
component. This function is called when the drawer is closed. It first navigates to a new URL without making an API call, and then closes the drawer.
Please note that this is a hypothetical example and may not work in your specific case. The actual implementation would depend on the specifics of your application and how it is set up.
Sources:
- useDrawerForm (https://refine.dev/docs/ui-integrations/mantine/hooks/use-drawer-form/)
- useNavigation (https://refine.dev/docs/api-reference/core/hooks/navigation/usenavigation/)
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@617879699363725341> kapa.ai is still learning and improving, please let us know how it did by reacting below
rival-blackOP•2y ago
@kapa.ai I used this way, but still calling the api
Let me check the knowledge base for you. One moment! :books:
----------------------
I'm sorry to hear that you're still experiencing issues. Unfortunately, without more specific information about your application's setup and the exact code you're using, it's difficult to provide a more precise solution.
The
useDrawerForm
and useNavigation
hooks in Refine don't directly control API calls. They are used for managing the state and behavior of forms within a drawer component and for navigating between pages, respectively. If an API call is being made when you navigate to a certain URL, this behavior is likely defined somewhere else in your application.
You might want to check the following:
- Look for any useEffect
hooks that are triggered when the URL changes. These hooks might be making the API call.
- Check your routing configuration. If you're using a library like React Router, the API call might be defined in the render
or component
prop of a Route
.
- If you're using Refine's resource
function to define your resources, check the edit
function. This function is called when you navigate to the edit page of a resource, and it might be making the API call.
If you're still having trouble, I recommend reaching out to the Refine community or checking the Refine documentation (https://refine.dev/docs) for more information.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@617879699363725341> kapa.ai is still learning and improving, please let us know how it did by reacting below
metropolitan-bronze•2y ago
Hey @hoaanhtuc, looks like the bot hallucinated a bit here 😅 sorry for that.
Since the route changes and the component re-mounts/updates the hooks are triggered for a new fetch, in your case it's the
useForm
's useOne
call to get the current data of the record.
You can disable that call by using queryOptions.enabled
but this will also disable the initial call. I think it will be a better choice to use queryOptions.staleTime
and queryOptions.cacheTime
to adjust your calls to not make redundant requests for a certain period of time. 🚀
Let me know if you have any further issues 🙏rival-blackOP•2y ago
I have solved it, thank you br 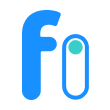
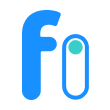