change title and icon
This is a really beginner question, but where can I change this?
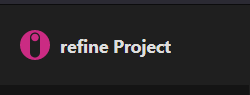
5 Replies
Hi @blackbearftw. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
You can change the title and icon in the
AuthPage
and ThemedLayout
components in Refine.
For the AuthPage
component, you can use the title
prop to change the default title. Here's an example:
You can also customize the title with the ThemedTitle
component:
For the ThemedLayout
component, you can replace the default ThemedTitle
component by passing a custom component to the Title
prop. Here's an example:
You can find more information in the Refine documentation (https://refine.dev/docs).
Please note that the specific import statements (@refinedev/antd
, @refinedev/mui
, etc.) depend on the UI library you are using with Refine.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@490961337896402944> kapa.ai is still learning and improving, please let us know how it did by reacting below
vicious-goldOP•13mo ago
@kapa.ai how can I edit the icons of the buttons, I want to use tabler icons
Let me check the knowledge base for you. One moment! :books:
----------------------
To use custom icons in your buttons, you can pass your desired icon component to the
icon
prop of the button component.
For example, if you're using Ant Design's Button
component, you can do something like this:
In the case of Refine's predefined buttons like EditButton
, DeleteButton
, etc., these components don't directly accept an icon
prop. However, you can create your own custom button components that use your desired icons and the functionalities provided by Refine.
Here's an example of how you can create a custom delete button with a custom icon:
You can then use MyDeleteButton
in your list view like this:
Please note that you'll need to replace MyCustomIcon
with your actual custom icon component from Tabler Icons.
For more information, you can refer to the Refine documentation (https://refine.dev/docs).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@490961337896402944> kapa.ai is still learning and improving, please let us know how it did by reacting below