How to add a component such as PostList or PostEdit as a child to a component
When using the supabase dataprovider starter tutorial I am trying to add the PostList component as a child to another unrelated component. The component loads the list accordingly but it is in a loading state. When checking on the network call the API call was made successfully and the dataprovider was invoked.
I am trying to do something similar to below
Something similar to below:
import React from "react";
import { PostList } from "./list";
function Hello() {
return (
<div>
<div>hello</div>
<PostList />
</div>
);
}
export default Hello;
When linking this component to my router I just get the loading spinner in my list.
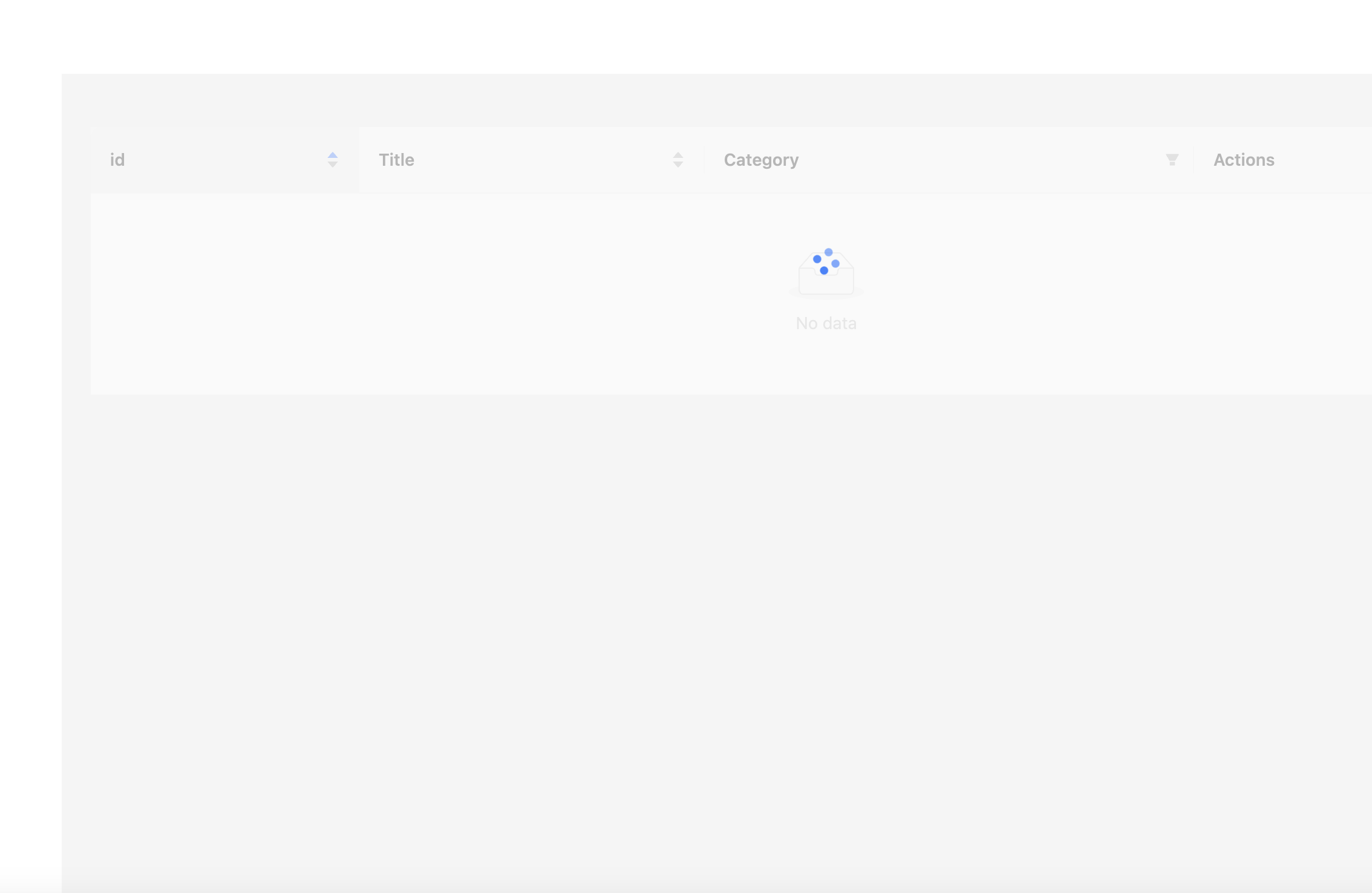
8 Replies
Hi @davlaha. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
The issue you're experiencing might be due to the fact that the
PostList
component is designed to be used within the Refine
component, which provides necessary context and hooks for data fetching. When you use PostList
outside of the Refine
component, it might not have access to the necessary context and hooks, hence the loading state.
Here's how you can use PostList
within the Refine
component:
In the code above, PostList
is used as a child of the Route
component, which is itself a child of the Refine
component. This way, PostList
has access to the necessary context and hooks provided by Refine
.
If you want to use PostList
in another component like Hello
, you should ensure that Hello
is used within the Refine
component, like so:
In this case, Hello
is used as a child of the Route
component, which is itself a child of the Refine
component. This way, PostList
(which is a child of Hello
) has access to the necessary context and hooks provided by Refine
.
For more information, you can refer to the Refine documentation (https://refine.dev/docs).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@689184849054924914> kapa.ai is still learning and improving, please let us know how it did by reacting below
ambitious-aquaOP•15mo ago
Hmmm okay so what is the recomended approach of having multiple components pulling from resources data sources in a single route
Can you give me a sample
@kapa.ai can you provide an example
Let me check the knowledge base for you. One moment! :books:
----------------------
Sure, here's an example of how you can use the
PostList
component as a child of another component within the Refine
component:
In this example, the Hello
component includes the PostList
component. The Hello
component is then used as a route in the Refine
component. This way, PostList
has access to the necessary context and hooks provided by Refine
.
Please note that the PostList
component needs to be defined properly. You can refer to the Refine documentation (https://refine.dev/docs) or the Refine blog (https://refine.dev/blog) for more information on how to define the PostList
component.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@689184849054924914> kapa.ai is still learning and improving, please let us know how it did by reacting below
ambitious-aquaOP•15mo ago
Thanks @kapa.ai I figured it out ... I was not passing the resource in correctly. Thanks
Let me check the knowledge base for you. One moment! :books:
----------------------
You're welcome! I'm glad to hear that you've figured it out. Remember, when using Refine, it's important to correctly define and pass the
resource
prop to ensure the proper functioning of your components. If you have any more questions or run into any other issues, feel free to ask. Happy coding!
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@689184849054924914> kapa.ai is still learning and improving, please let us know how it did by reacting below