How to return a specific error message from the backend server for the resource update / create?
Hi, I'm using
@nestjsx/crud
adapter with Refine. What I want to do is that I want to rewrite some of the services and provide a meaningful error message when there's some validation errors on the backend, and show it on the front-end after saving the content. Is that doable?11 Replies
Hi @Eric Hu. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
Yes, it's definitely doable. Refine supports server-side validation out-of-the-box in all
useForm
derivatives. To handle server-side validation, the data providers need to be correctly set up to return the errors in form submissions with a specific format. After this, Refine's useForm
will propagate the errors to the respective form fields.
Here's an example of how you can return a specific error message from the backend:
In this example, the errors
field is required for server-side validation. When the errors
field is set, useForm
will automatically display the error messages in the form with the corresponding fields. The message
field is the error message that you want to display.
For more information, you can check out the Refine documentation on server-side validation (https://refine.dev/docs/guides-concepts/forms#server-side-validation-).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@454649036289343500> kapa.ai is still learning and improving, please let us know how it did by reacting below
dependent-tanOP•16mo ago
Thanks, I think it should work. However, how can I use it with
@nestjsx/crud
? I tried this but it doesn't work:

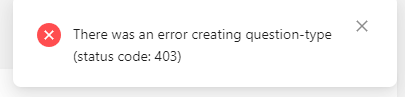
dependent-tanOP•16mo ago
@kapa.ai How to use the code you showed me above? Should I install
@refinedev/core
on my backend?Let me check the knowledge base for you. One moment! :books:
----------------------
No, you don't need to install
@refinedev/core
on your backend. The code I provided was just an example of the structure that your backend should return when a validation error occurs.
In your NestJS service, when a validation error occurs, you should throw an HttpException
with a similar structure. Here's an example:
In this example, HttpException
is a class from @nestjs/common
and HttpStatus
is an enum from the same package. The errors
object keys should match the names of your form fields. The error messages you provide here will be displayed next to the corresponding form fields in the Refine form.
Remember, the key point here is that your backend needs to return the errors in the mutation response with the expected format for Refine to handle them correctly.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@454649036289343500> kapa.ai is still learning and improving, please let us know how it did by reacting below
dependent-tanOP•16mo ago
@kapa.ai Is there any example with Nest.js?
I tried this but it doesn't work:
Let me check the knowledge base for you. One moment! :books:
----------------------
I'm sorry, but based on the information extracts provided, there isn't a specific example of handling server-side validation errors with Nest.js and Refine. However, the code you've shared seems correct in terms of throwing an
HttpException
with a specific error message.
If it's not working as expected, the issue might be somewhere else in your code or it could be related to how the error is handled on the frontend with Refine.
Refine expects errors to be in a specific format to handle them correctly. The errors
object in the response should have keys that match the names of your form fields. The error messages you provide here will be displayed next to the corresponding form fields in the Refine form.
If you're still having trouble, I would recommend checking the Refine documentation (https://refine.dev/docs) and Nest.js documentation (https://docs.nestjs.com/exception-filters) for more information on error handling.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@454649036289343500> kapa.ai is still learning and improving, please let us know how it did by reacting below
dependent-tanOP•16mo ago
It turned out that the adapter of @nestjsx/crud has got some issues with server error handling. I forked it and rewrote this part. And it works now.
optimistic-gold•16mo ago
Hello @Eric Hu happy to see you fixed the issue, would you like to create a PR for this one?