LoginInput password not a valid input
I'm trying to use both email and password to login
login: async ({ email, password }) => {
try {
// call the login mutation
// dataProvider.custom is used to make a custom request to the GraphQL API
// this will call dataProvider which will go through the fetchWrapper function
const { data } = await dataProvider.custom({
url: API_URL,
method: "post",
headers: {},
meta: {
variables: { email, password },
// pass the email to see if the user exists and if so, return the accessToken
rawQuery:
mutation Login($email: String!, $password: String!) {
login(loginInput: { email: $email, password: $password }) {
accessToken
}
}
,
},
});
// save the accessToken in localStorage
localStorage.setItem("access_token", data.login.accessToken);
return {
success: true,
redirectTo: "/",
};
} catch (e) {
const error = e as Error;
return {
success: false,
error: {
message: "message" in error ? error.message : "Login failed",
name: "name" in error ? error.name : "Invalid email or password",
},
};
}
},
but it doesn't want to accpet the password entry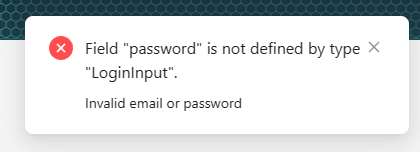
5 Replies
Hi @saaaadXD. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
Based on the provided knowledge sources, it's not clear why the
password
entry is not being accepted in your login
function. However, there are a few things you could check:
1. Ensure that the password
is being correctly passed into the login
function. You can do this by logging the password
value at the start of the function.
2. Check the GraphQL API you're calling with dataProvider.custom
. Ensure that it's expecting a password
field in the loginInput
object and that the password
field is of the correct type.
3. Make sure that the password
value meets any requirements set by the GraphQL API, such as minimum length or inclusion of certain characters.
4. If the password
is being encrypted or hashed before being sent to the API, ensure that this process is working correctly.
If you're still having trouble, you might find it helpful to look at the Refine documentation (https://refine.dev/docs), particularly the sections on authentication (https://refine.dev/docs/authentication/auth-provider/) and custom data providers (https://refine.dev/docs/data-provider/introduction/).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@504686295491280917> kapa.ai is still learning and improving, please let us know how it did by reacting below
blank-aquamarineOP•14mo ago
@kapa.ai how do I check the graphql api
@kapa.ai how do i check the graphql api
Let me check the knowledge base for you. One moment! :books:
----------------------
To check your GraphQL API, you can use a tool like GraphQL Playground (https://github.com/graphql/graphql-playground), GraphiQL (https://github.com/graphql/graphiql), or Apollo Studio (https://studio.apollographql.com/). These tools allow you to send queries and mutations to your GraphQL server and see the responses in real time.
Here's a general process you can follow:
1. Open your GraphQL tool of choice and enter the URL of your GraphQL API.
2. Write a mutation that mimics the login process. Based on your code, it might look something like this:
3. In the variables section of the tool, provide an
email
and password
to test the mutation:
4. Run the mutation and check the response. If there's an error, it should give you more information about what's going wrong.
Remember to replace the email
and password
values with ones that are valid for your application.
If you're using a custom GraphQL server, you might also want to check the server logs for any errors or warnings. If you have access to the server code, you can also check the schema and resolvers to make sure they're set up correctly.
For more information on how to use GraphQL with Refine, you can refer to the Refine documentation on GraphQL (https://refine.dev/docs/packages/documentation/data-providers/graphql/).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@504686295491280917> kapa.ai is still learning and improving, please let us know how it did by reacting below