Access data provider in child component
How can i access data provider in a child component? I want to get the api base url in my component
117 Replies
Hi @biohazard#1521. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
You can access the data provider in a child component using the
useDataProvider
and useApiUrl
hooks provided by Refine.
The useDataProvider
hook returns the dataProvider
which is passed to the <Refine>
component. It is useful when you have multiple data providers and you want to access one of them. Here is an example of how to use it:
(Source: useDataProvider (https://refine.dev/docs/data/hooks/use-data-provider))
The useApiUrl
hook returns the API URL. It uses the getApiUrl
method to get the API URL from the dataProvider
. Here is an example of how to use it:
(Source: useApiUrl (https://refine.dev/docs/data/hooks/use-api-url))
Remember, these hooks can only be used inside a component that is a child of the <Refine>
component.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai how can i access the theme object in a component?
Let me check the knowledge base for you. One moment! :books:
----------------------
You can access the theme object in a component using the
useTheme
hook provided by the UI library you are using.
For example, if you are using Chakra UI, you can use the useTheme
hook as follows:
(Source: Chakra UI useTheme (https://refine.dev/blog/chakra-ui#usetheme))
If you are using Material UI, you can use the useTheme
hook as follows:
(Source: Material UI useTheme (https://mui.com/styles/api/#usetheme-theme))
If you are using Ant Design, you can use the useContext
hook with ConfigContext
to access the theme:
(Source: Ant Design ConfigProvider (https://ant.design/components/config-provider/))
Remember, these hooks can only be used inside a component that is a child of the <ThemeProvider>
or <ChakraProvider>
or <ConfigProvider>
component.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai in my edit form i have an image field, and I want to add a button that would make an upload input appear, how could i implement this?
Let me check the knowledge base for you. One moment! :books:
----------------------
You can achieve this by using a state variable to control the visibility of the upload input. Here's a basic example of how you can implement this in your form:
In this example, clicking the "Upload Image" button sets the
showUpload
state to true
, which causes the Upload.Dragger
component to be rendered.
Please note that you need to replace "/upload"
in the action
prop with your actual upload endpoint.
For more information on how to use the Upload
component in Refine, you can refer to the Multipart Upload guide (https://refine.dev/docs/advanced-tutorials/upload/multipart-upload#create-form) and the Supabase guide (https://refine.dev/docs/data/packages/supabase#adding-an-edit-page) in the Refine documentation.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai how can i implement a tag input in refine with antd? I want to be able to add tags as well as remove them
Let me check the knowledge base for you. One moment! :books:
----------------------
You can implement a tag input in Refine with Ant Design using the
useCheckboxGroup
hook. This hook allows you to manage an Ant Design Checkbox.Group component when records in a resource need to be used as checkbox options.
Here's an example of how you can use it:
In this example, useCheckboxGroup
is used to fetch data from the tags
resource. The checkboxGroupProps
it returns are then passed to the <Checkbox.Group>
component. This allows you to add and remove tags by checking and unchecking the checkboxes.
For more information, refer to the useCheckboxGroup
documentation (https://refine.dev/docs/ui-integrations/ant-design/hooks/use-checkbox-group).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai any way to do it using <TagField/>?
Let me check the knowledge base for you. One moment! :books:
----------------------
The
<TagField />
component in Refine with Ant Design is used to display a value in a tag, but it doesn't provide the functionality to add or remove tags. It uses Ant Design's <Tag>
component under the hood.
If you want to implement a tag input where users can add or remove tags, you should use Ant Design's <Select>
component with the mode
prop set to "tags"
. This allows users to create and remove tags.
Here's an example:
In this example, the <Select>
component is in "tags" mode, which allows users to create and remove tags. You can render <Option>
components inside the <Select>
component to provide initial options.
For more information, refer to the Ant Design Select documentation (https://ant.design/components/select/).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai in my form i am trying to render a list of objects in as a Form.List which i want to have multiple inputs corresponding to different fields of the object. I have this now:
<Form.List name={'intervals'}>
{(fields, { add, remove }, { errors }) => (
<>
<Title level={5}>Stimulation Intervals</Title>
{fields.map((field, index) => {
console.log(
'FIELDS MAP - ',
field,
fields,
form.getFieldsValue(),
);
return (
<Form.Item required={false} key={field.key}>
<Form.Item
{...field}
validateTrigger={['onChange', 'onBlur']}
rules={[
{
required: true,
whitespace: true,
message:
'Please input something or delete this field.',
},
]}
noStyle
>
{/<TestInput/>/}
<Input
key="fromSeconds"
placeholder="Interval"
style={{ width: '90%' }}
autoFocus={form.isFieldsTouched()}
/>
basically the field name is intervals, which is an array of intervals which have a "from" and "to" field, but i don't understand how to map either of those values to the input. Right now i get [object Object] in the input value
Let me check the knowledge base for you. One moment! :books:
----------------------
In your case, you have an array of objects and each object has two fields: "from" and "to". You can map these fields to inputs by creating nested
Form.Item
components inside the Form.List
.
Here's an example of how you can do this:
In this example, each object in the intervals
array is represented by a Space
component that contains two Form.Item
components: one for the "from" field and one for the "to" field. The name
prop of each Form.Item
is an array that specifies the path to the field in the form data.
For more information, refer to the Ant Design Form.List documentation (https://ant.design/components/form/#components-form-demo-dynamic-form-item).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai in refine antd form, how can i check whether any changes were made to the form before submitting? I want to prevent update calls when no values were changed
Let me check the knowledge base for you. One moment! :books:
----------------------
In Refine, you can use the
warnWhenUnsavedChanges
property of the useForm
or useModalForm
hooks to show a warning when the user tries to leave the page with unsaved changes. This property is false
by default, but you can set it to true
to activate the feature.
Here's how you can use it with useForm
:
(Source: useForm warnWhenUnsavedChanges (https://refine.dev/docs/ui-integrations/ant-design/hooks/use-form#warnwhenunsavedchanges))
And here's how you can use it with useModalForm
:
(Source: useModalForm warnWhenUnsavedChanges (https://refine.dev/docs/ui-integrations/ant-design/hooks/use-modal-form#warnwhenunsavedchanges))
You can also set this value globally in the Refine
component:
(Source: Refine warnWhenUnsavedChanges (https://refine.dev/docs/core/refine-component#warnwhenunsavedchanges))
This feature requires the UnsavedChangesNotifier
component to be mounted. You can import this component from your router package.
Please note that this feature only shows a warning when the user tries to leave the page. It doesn't prevent the form from being submitted when no values were changed. If you want to prevent the form from being submitted when no values were changed, you would need to implement custom logic in your form's onFinish
handler to compare the initial and current form values.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai this is not what i want. I want to control the Edit form "save" button and check if values have changed, and if they did only then i want to make the api call
Let me check the knowledge base for you. One moment! :books:
----------------------
You can achieve this by using the
form
instance returned by the useForm
hook. The form
instance has a isFieldsTouched
method that you can use to check if any fields have been touched (i.e., changed) before submitting the form.
Here's an example of how you can do this:
In this example, the handleOnFinish
function checks if any fields have been touched using the form.isFieldsTouched
method. If no fields have been touched, it returns immediately and doesn't submit the form. If any fields have been touched, it submits the form.
Please note that this example assumes that you're using the onFinish
prop of the Form
component to handle form submissions. If you're using a different method to handle form submissions, you may need to adjust this example accordingly.
For more information, refer to the Ant Design Form documentation (https://ant.design/components/form/#components-form-demo-dynamic-form-item) and the Refine useForm documentation (https://refine.dev/docs/api-reference/antd/hooks/form/useForm).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai this works with regular inputs, but i have a case where i manually set a field value by calling form.setFieldValue(), and in that case it doesn't recognize field as touched. how to incorporate this?
Let me check the knowledge base for you. One moment! :books:
----------------------
When you manually set a field value using
form.setFieldsValue()
, Ant Design's form doesn't consider the field as "touched". A field is only considered "touched" when the user has interacted with it.
To work around this, you can manually mark the field as touched using the form.touchField()
method after setting its value. Here's an example:
In this example, fieldName
is the name of the field you're setting the value for, and value
is the value you're setting.
After calling form.touchField('fieldName')
, the form.isFieldsTouched()
method will return true
if fieldName
is the only field you've interacted with.
For more information, refer to the Ant Design Form documentation (https://ant.design/components/form/#components-form-demo-dynamic-form-item).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai form.touchField does not exist
Let me check the knowledge base for you. One moment! :books:
----------------------
I apologize for the confusion. You're correct, there's no
touchField
method in Ant Design's Form API.
To track changes when you manually set a field value with form.setFieldsValue()
, you could maintain a separate state to keep track of whether the form has been modified.
Here's an example:
In this example, setFieldValue
is a function that sets a field value and marks the form as modified by calling setIsModified(true)
. The handleOnFinish
function then checks the isModified
state in addition to form.isFieldsTouched()
to determine whether to submit the form.
Please adjust this example according to your needs.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai how can i use the form values to render them in jsx? I want to display the changed value in a custom way
Let me check the knowledge base for you. One moment! :books:
----------------------
You can use the
form
instance returned by the useForm
hook to get the current form values. The form
instance has a getFieldsValue
method that you can use to get the current form values.
Here's an example of how you can do this:
In this example, form.getFieldsValue()
is called inside a pre
element to display the current form values as JSON. The JSON.stringify
function is used to convert the form values to a JSON string, and the null
and 2
arguments are used to format the JSON string with two spaces of indentation.
Please adjust this example according to your needs.
For more information, refer to the Ant Design Form documentation (https://ant.design/components/form/#components-form-demo-dynamic-form-item) and the Refine useForm documentation (https://refine.dev/docs/api-reference/antd/hooks/form/useForm).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai in my antd form i want to add some validation. I have 2 fields, "icon" and "remoteIcon" and i want the validation to require at least one. So if none are filled, it would error on both, but if "icon" or "remoteIcon" is filled, the validation would pass and no errors on either. How can i achieve that?
Let me check the knowledge base for you. One moment! :books:
----------------------
You can achieve this by using a custom validator in the
rules
prop of the Form.Item
component. The custom validator can access all field values, so you can use it to check if at least one of the "icon" and "remoteIcon" fields is filled.
Here's an example:
In this example, the validateIcon
function is a custom validator that checks if at least one of the "icon" and "remoteIcon" fields is filled. If neither field is filled, it rejects the Promise with an error message. If at least one field is filled, it resolves the Promise, indicating that the field is valid.
Please adjust this example according to your needs.
For more information, refer to the Ant Design Form documentation (https://ant.design/components/form/#components-form-demo-dynamic-form-item) and the Refine Custom Form Validation documentation (https://refine.dev/docs/examples/form/antd/custom-form-validation).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai this goes into an infinite loop and crashes my app.
Let me check the knowledge base for you. One moment! :books:
----------------------
I apologize for the confusion. The issue is that the
validateFields
method triggers the validators, which in turn call validateFields
again, causing an infinite loop.
Instead, you can use the getFieldsValue
method to get the current form values without triggering the validators. Here's how you can do it:
In this example, the validateIcon
function is a custom validator that checks if at least one of the "icon" and "remoteIcon" fields is filled. It uses the getFieldsValue
method to get the current form values without triggering the validators. If neither field is filled, it rejects the Promise with an error message. If at least one field is filled, it resolves the Promise, indicating that the field is valid.
Please adjust this example according to your needs.
For more information, refer to the Ant Design Form documentation (https://ant.design/components/form/#components-form-demo-dynamic-form-item).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai in a antd table can i pass multiple keys to a column? For example now I have dataIndex="icon", but i want to have both "icon" and "remoteIcon" there to execute custom logic
Let me check the knowledge base for you. One moment! :books:
----------------------
Yes, you can pass an array of keys to the
dataIndex
prop of a column in an Ant Design Table. This is useful when you want to access nested data.
However, if you want to execute custom logic based on the values of both "icon" and "remoteIcon", you can use the render
prop of the column. The render
function receives the value of the column, the record of the row, and the row index as arguments, so you can access any field of the record.
Here's an example:
In this example, the render
function of the "Icon" column accesses both the "icon" and "remoteIcon" fields of the record. You can replace the comment with your custom logic and JSX.
For more information, refer to the Ant Design Table documentation (https://ant.design/components/table/#Column).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai how can i set custom width for my ThemedSiderV2 component?
Let me check the knowledge base for you. One moment! :books:
----------------------
The
ThemedSiderV2
component from Refine doesn't directly accept a width prop. However, you can customize it using CSS. You can create a custom CSS class and apply it to the ThemedSiderV2
component.
Here's an example:
And in your App.css
file:
In this example, the custom-sider
CSS class sets the width of the ThemedSiderV2
component to 300px
.
Please adjust this example according to your needs.
For more information, refer to the Refine documentation (https://refine.dev/docs).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai this doesn't work. the sider component doesn't take a className prop.
Let me check the knowledge base for you. One moment! :books:
----------------------
I apologize for the confusion. You're correct, the
ThemedSiderV2
component doesn't accept a className
prop.
The ThemedSiderV2
component in Refine is a wrapper around the Ant Design Layout.Sider
component. It doesn't directly expose a prop to set the width, but it does have a fixed
prop that makes the sidebar fixed and sets its width to 256px
.
Here's an example:
In this example, the fixed
prop is passed to the ThemedSiderV2
component, making the sidebar fixed and setting its width to 256px
.
If you need a custom width, you would need to create a custom sider component. You can use the swizzle
command to copy the default Sider
component to your project and customize it as you want.
For more information, refer to the Refine documentation (https://refine.dev/docs) and the Ant Design Layout.Sider documentation (https://ant.design/components/layout/#Layout.Sider).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------genetic-orange•3mo ago
@kapa.ai now in my Refine component resources , i want to pass a custom svg icon. I am trying to pass it like this, which works, but the color does not change when it is selected:
<Icon
size={5}
style={{ width: 20 }}
component={BreathIcon}
/>
Let me check the knowledge base for you. One moment! :books:
----------------------
The color of the SVG icon not changing when selected is likely due to the SVG's
fill
property being set to a specific color. When the fill
property is set, it overrides any color applied through CSS.
To make the SVG icon color changeable, you can remove the fill
attribute from the SVG itself and control the color through CSS. You can set the fill
property to currentColor
in your CSS, which will make the SVG take on the color of the text.
Here's an example:
In this example, the fill
property of the style
prop is set to currentColor
, which makes the SVG take on the color of the text.
Please adjust this example according to your needs.
For more information, refer to the Ant Design Icon documentation (https://ant.design/components/icon/) and the Refine documentation (https://refine.dev/docs).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai in my edit form i want to use a table as a form field, so I would use the drag sortable table from Antd, and i want to use it as a field. How can i achieve this?
Let me check the knowledge base for you. One moment! :books:
----------------------
To use a table as a form field in Ant Design, you can use the
Form.List
component. Form.List
can be used to handle dynamic form fields, where you can add, remove, and rearrange form items.
Here's an example of how you can use a table as a form field:
``jsx
import { Form, Input, Button, Table } from "antd";
import { DragOutlined } from "@ant-design/icons";
import { DndProvider, useDrag, useDrop } from "react-dnd";
import { HTML5Backend } from "react-dnd-html5-backend";
import update from "immutability-helper";
const type = "DragableBodyRow";
const DragableBodyRow = ({ index, moveRow, className, style, ...restProps }) => {
const ref = React.useRef();
const [{ isOver, dropClassName }, drop] = useDrop(() => ({
accept: type,
collect: (monitor) => {
const { index: dragIndex } = monitor.getItem() || {};
if (dragIndex === index) {
return {};
}
return {
isOver: monitor.isOver(),
dropClassName: dragIndex < index ? " drop-over-downward" : " drop-over-upward",
};
},
drop: (item) => {
moveRow(item.index, index);
},
}));
const [, drag] = useDrag(() => ({
type,
item: { index },
collect: (monitor) => ({
isDragging: monitor.isDragging(),
}),
}));
drop(drag(ref));
return (
<tr
ref={ref}
className={
${className}${isOver ? dropClassName : ""}`}
style={{ cursor: "move", ...style }}
{...restProps}
/>
);
};
const MyForm = () => {
const [form] = Form.useForm();
const [data, setData] = React.useState([
{
key: "1",
name: "John Brown",
age: 32,
address: "New York No. 1 Lake Park",
},
{
key: "2",
name: "Jim Green",
age: 42,
address: "London No. 1 Lake Park",
},
{
key: "3",
name: "Joe Black",
age: 32,
address: "Sidney No. 1 Lake Park",
},
]);
const components = {
body: {
row: DragableBodyRow,
},
};
const moveRow = (dragIndex, hoverIndex) => {
const dragRow = data[dragIndex];
setData(
update(data, {
$splice: [
[dragIndex, 1],
[hoverIndex, 0, dragRow],
],
})
);
};
const columns = [
{
title: "Name",
dataIndex: "name",
key: "name",
render: (text, record, index) => (
<Form.Item
name={["users", index, "name"]}
initialValue={text}
rules={[{ required: true, message: "Please input name!" }]}
>
<Input />
</Form.Item>
),
},
{
title: "Age",
dataIndex: "age",
key: "age",
render: (text, record, index) => (
<Form.Item
name={["users", index, "age"]}
initialValue={text}
rules={[{ required: true, message: "Please input age!" }]}
>
<Input />
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai can you update the formatting on this? it is difficult to read
Let me check the knowledge base for you. One moment! :books:
----------------------
I apologize for the formatting issue. Here's the formatted code:
``jsx
import { Form, Input, Button, Table } from "antd";
import { DragOutlined } from "@ant-design/icons";
import { DndProvider, useDrag, useDrop } from "react-dnd";
import { HTML5Backend } from "react-dnd-html5-backend";
import update from "immutability-helper";
const type = "DragableBodyRow";
const DragableBodyRow = ({ index, moveRow, className, style, ...restProps }) => {
const ref = React.useRef();
const [{ isOver, dropClassName }, drop] = useDrop(() => ({
accept: type,
collect: (monitor) => {
const { index: dragIndex } = monitor.getItem() || {};
if (dragIndex === index) {
return {};
}
return {
isOver: monitor.isOver(),
dropClassName: dragIndex < index ? " drop-over-downward" : " drop-over-upward",
};
},
drop: (item) => {
moveRow(item.index, index);
},
}));
const [, drag] = useDrag(() => ({
type,
item: { index },
collect: (monitor) => ({
isDragging: monitor.isDragging(),
}),
}));
drop(drag(ref));
return (
<tr
ref={ref}
className={
${className}${isOver ? dropClassName : ""}`}
style={{ cursor: "move", ...style }}
{...restProps}
/>
);
};
const MyForm = () => {
const [form] = Form.useForm();
const [data, setData] = React.useState([
{
key: "1",
name: "John Brown",
age: 32,
address: "New York No. 1 Lake Park",
},
{
key: "2",
name: "Jim Green",
age: 42,
address: "London No. 1 Lake Park",
},
{
key: "3",
name: "Joe Black",
age: 32,
address: "Sidney No. 1 Lake Park",
},
]);
const components = {
body: {
row: DragableBodyRow,
},
};
const moveRow = (dragIndex, hoverIndex) => {
const dragRow = data[dragIndex];
setData(
update(data, {
$splice: [
[dragIndex, 1],
[hoverIndex, 0, dragRow],
],
})
);
};
const columns = [
{
title: "Name",
dataIndex: "name",
key: "name",
render: (text, record, index) => (
<Form.Item
name={["users", index, "name"]}
initialValue={text}
rules={[{ required: true, message: "Please input name!" }]}
>
<Input />
</Form.Item>
),
},
{
title: "Age",
dataIndex: "age",
key: "age",
render: (text, record, index) => (
<Form.Item
name={["users", index, "age"]}
initialValue={text}
rules={[{ required: true, message: "Please input age!" }]}
>
<Input />
</Form.Item>
),
},
// Other columns...
];
return (
<DndProvider backend={HTML5Backend}>
<Form form={form}>
<Table
components={components}
dataSource={data}
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai your example is not finished, make a full example
Let me check the knowledge base for you. One moment! :books:
----------------------
I apologize for the incomplete example. Here's the complete example of how you can use a table as a form field with drag and drop functionality:
``jsx
import React, { useRef } from 'react';
import { Form, Input, Button, Table } from 'antd';
import { DndProvider, useDrag, useDrop } from 'react-dnd';
import { HTML5Backend } from 'react-dnd-html5-backend';
import update from 'immutability-helper';
const type = 'DragableBodyRow';
const DragableBodyRow = ({ index, moveRow, className, style, ...restProps }) => {
const ref = useRef();
const [{ isOver, dropClassName }, drop] = useDrop(() => ({
accept: type,
collect: (monitor) => {
const { index: dragIndex } = monitor.getItem() || {};
if (dragIndex === index) {
return {};
}
return {
isOver: monitor.isOver(),
dropClassName: dragIndex < index ? ' drop-over-downward' : ' drop-over-upward',
};
},
drop: (item) => {
moveRow(item.index, index);
},
}));
const [, drag] = useDrag(() => ({
type,
item: { index },
collect: (monitor) => ({
isDragging: monitor.isDragging(),
}),
}));
drop(drag(ref));
return (
<tr
ref={ref}
className={
${className}${isOver ? dropClassName : ''}`}
style={{ cursor: 'move', ...style }}
{...restProps}
/>
);
};
const MyForm = () => {
const [form] = Form.useForm();
const [data, setData] = React.useState([
{
key: '1',
name: 'John Brown',
age: 32,
address: 'New York No. 1 Lake Park',
},
{
key: '2',
name: 'Jim Green',
age: 42,
address: 'London No. 1 Lake Park',
},
{
key: '3',
name: 'Joe Black',
age: 32,
address: 'Sidney No. 1 Lake Park',
},
]);
const components = {
body: {
row: DragableBodyRow,
},
};
const moveRow = (dragIndex, hoverIndex) => {
const dragRow = data[dragIndex];
setData(
update(data, {
$splice: [
[dragIndex, 1],
[hoverIndex, 0, dragRow],
],
})
);
};
const columns = [
{
title: 'Name',
dataIndex: 'name',
key: 'name',
render: (text, record, index) => (
<Form.Item
name={['users', index, 'name']}
initialValue={text}
rules={[{ required: true, message: 'Please input name!' }]}
>
<Input />
</Form.Item>
),
},
{
title: 'Age',
dataIndex: 'age',
key: 'age',
render: (text, record, index) => (
<Form.Item
name={['users', index, 'age']}
initialValue={text}
rules={[{ required: true, message: 'Please input age!' }]}
>
<Input />
</Form.Item>
),
},
// Other columns...
];
return (
<DndProvider backend={HTML5Backend}>
<Form form={form}>
<Table
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai with a useTable in a <List> component, how can i make the filters/pagination create a step in the browser history? for example if i go to page 2, i want the back button to go back to page 1
Let me check the knowledge base for you. One moment! :books:
----------------------
To make the filters and pagination create a step in the browser history in a
useTable
within a <List>
component, you can use the syncWithLocation
option in the <Refine>
component. This option synchronizes the parameters (filters, pagination, sorting) with the current route.
Here's an example of how to enable this:
Once you pass syncWithLocation: true
to <Refine>
component's options
prop, useTable
will:
- Read the current route and update its parameters (filters, pagination, sorting) accordingly.
- Update the current route when its parameters (filters, pagination, sorting) change.
For example, if you have a products
list page with the following route:
And you want to filter products by category.id
and sort them by id
in asc
order. You can pass these parameters to useTable
hook as follows:
useTable
will automatically update the route to:
And you will see a list of products, already filtered, sorted, and paginated automatically based on the query parameters of the current route.
For more information, refer to the Refine documentation (https://refine.dev/docs/guides-concepts/routing#usetable-).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
i already have syncWithLocation set as true in my Refine component. The filters and queries will continue to work after refresh, but the history doesn't update
@kapa.ai
Let me check the knowledge base for you. One moment! :books:
----------------------
The
syncWithLocation
option in Refine synchronizes the table state (filters, sorters, and pagination) with the URL. However, it uses the replaceState
method of the History API, which modifies the current history entry instead of creating a new one. This means that the browser's back button won't revert the table state to the previous state.
If you want the table state changes to create new history entries, you would need to implement a custom solution. You could use the pushState
method of the History API to create a new history entry whenever the table state changes. However, this would require a deep understanding of both Refine and the History API, and it might not be straightforward to implement.
For more information, refer to the Refine documentation (https://refine.dev/docs/guides-concepts/routing#usetable-) and the History API documentation (https://developer.mozilla.org/en-US/docs/Web/API/History_API).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai in refine i am using a delete button with undoable, which seems to work fine but the undo notification that pops up never disappears even after the timer runs out. My button is used like this:
<DeleteButton
size="small"
recordItemId={
${id}
}
hideText
mutationMode="undoable"
successNotification={{
message: 'Sucessfully deleted a Breathing Session',
type: 'success',
}}
/>Let me check the knowledge base for you. One moment! :books:
----------------------
The issue you're experiencing might be related to a bug in Refine where the undoable mutation is called multiple times. This issue has been reported and a possible fix has been suggested in this Github issue (https://github.com/refinedev/refine/issues/2556).
However, without more information, it's hard to say exactly what's causing the issue in your case. It could be related to how the
DeleteButton
is used or it could be a bug in Refine itself.
If you're using a custom notification provider, make sure that you're handling the undoable
mutation mode correctly. When the mutationMode
is undoable
, Refine applies the mutation locally and immediately redirects and updates the UI as if the mutation is successful. It then waits for a customizable timeout before applying the mutation. During the timeout, you can cancel the mutation and revert the UI.
Here's an example of how to create a custom undoable notification component:
You can find more details in the Refine documentation (https://refine.dev/docs).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai i am using
useNotificationProvider
from refineLet me check the knowledge base for you. One moment! :books:
----------------------
If you're using
useNotificationProvider
from Refine and the undo notification doesn't disappear after the timer runs out, it might be due to a configuration issue or a bug in Refine.
The useNotificationProvider
hook is used to show and hide notifications in Refine. When you use the mutationMode
prop with the value undoable
in the DeleteButton
, it should show an undo notification and then automatically hide it after a certain timeout.
Here's an example of how you should use the DeleteButton
:
If you've set it up correctly and it's still not working, it might be a bug in Refine. In that case, I recommend creating an issue in the Refine GitHub repository (https://github.com/pankod/refine/issues) to get help from the maintainers.
For more information, refer to the Refine documentation (https://refine.dev/docs).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai after time runs out i end up with the notification in the screenshot
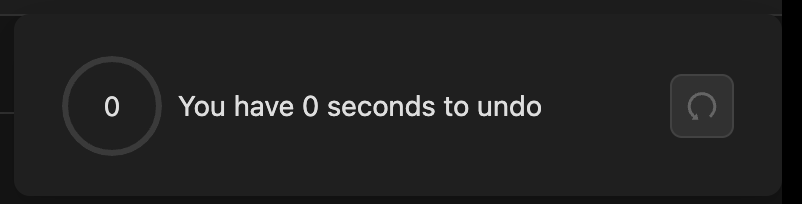
Let me check the knowledge base for you. One moment! :books:
----------------------
I'm sorry, but as an AI, I'm unable to view screenshots or images. However, if you're experiencing issues with the undo notification not disappearing after the timer runs out, it might be a bug or a configuration issue.
If you believe it's a bug, I recommend creating an issue in the Refine GitHub repository (https://github.com/pankod/refine/issues) with a detailed description of the problem and the screenshot you mentioned. The maintainers of the library should be able to help you.
If it's a configuration issue, please ensure that you've correctly set up the
DeleteButton
with the mutationMode
prop set to undoable
. Here's an example:
For more information, refer to the Refine documentation (https://refine.dev/docs).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai so in the end i see "You have 0 seconds to undo" and it never goes away
Let me check the knowledge base for you. One moment! :books:
----------------------
The issue you're experiencing might be related to a bug in Refine where the undoable mutation is called many times. This issue has been reported and a possible fix has been suggested in this Github issue (https://github.com/refinedev/refine/issues/2556).
However, without more information, it's hard to say exactly what's causing the issue in your case. It could be related to how the
DeleteButton
is used or it could be a bug in Refine itself.
If you're using a custom notification provider, make sure that you're handling the undoable
mutation mode correctly. When the mutationMode
is undoable
, Refine applies the mutation locally and immediately redirects and updates the UI as if the mutation is successful. It then waits for a customizable timeout before applying the mutation. During the timeout, you can cancel the mutation and revert the UI.
Here's an example of how to create a custom undoable notification component:
You can find more details in the Refine documentation (https://refine.dev/docs).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai no i don't use a custom notification provider
Let me check the knowledge base for you. One moment! :books:
----------------------
If you're using the default notification provider from Refine and the undo notification doesn't disappear after the timer runs out, it might be a bug in Refine.
In this case, I recommend creating an issue in the Refine GitHub repository (https://github.com/pankod/refine/issues) with a detailed description of the problem. The maintainers of the library should be able to help you.
Please include the following details in your issue:
- A brief description of the issue.
- Steps to reproduce the issue.
- Expected behavior (e.g., the undo notification should disappear after the timer runs out).
- Actual behavior (e.g., the undo notification doesn't disappear and shows "You have 0 seconds to undo").
- Any error messages you're seeing in the console.
- Your Refine version.
- Any other relevant information (e.g., browser version, operating system).
This will help the maintainers understand and reproduce the issue, and hopefully provide a fix or a workaround.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai ok so how can i implement the custom provider?
Let me check the knowledge base for you. One moment! :books:
----------------------
To implement a custom notification provider in Refine, you can follow these steps:
1. Create a new folder in your
src
directory and name it providers
. Inside this folder, create a new file and name it NotificationProvider.tsx
.
2. In NotificationProvider.tsx
, import the necessary dependencies and define your custom notification provider. Here's an example using react-toastify
:
3. Now, you need to use this custom notification provider in your application. You can do this by passing it as a prop to the Refine
component in your App.tsx
file:
This will replace the default notification provider with your custom one. Now, whenever a notification is triggered in your application, it will be handled by your custom notification provider.
For more information, refer to the Refine documentation (https://refine.dev/docs/guides-concepts/notifications#custom-notification-provider) and the Refine blog post (https://refine.dev/blog/react-toastify#create-a-notification-provider-with-react-toastify) on creating a custom notification provider.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai with antd i use <Upload.Dragger> component to handle uploads. Now i want to also preview files when i'm in my edit form. So after you load the page, I want to pass some initial preview files that weren't uploaded in current session. is there a way to do that?
Let me check the knowledge base for you. One moment! :books:
----------------------
Yes, you can preview files in the
<Upload.Dragger>
component by using the fileList
prop. The fileList
prop accepts an array of file objects. Each file object can have properties like uid
, name
, status
, and url
. The url
property is used to provide the URL of the file for preview.
Here's an example of how you can use the fileList
prop to provide initial preview files:
In this example, useState
is used to manage the state of the fileList
. The initial state of the fileList
includes one file with the status
set to 'done'
, which means the file is already uploaded. The url
property is set to the URL of the file, which is used for preview.
You can update the fileList
state when new files are uploaded or existing files are removed.
For more information, refer to the Ant Design documentation (https://ant.design/components/upload/#API).
In the context of Refine, you can use the initialValues
prop of the <Edit>
component to provide initial values for the form fields, including the file field. Here's an example from the Refine documentation (https://refine.dev/docs/data/packages/appwrite#edit-page):
In this example, the initialValues
prop is used to provide an initial value for the images
field. The initial value includes one file with the status
set to 'done'
, which means the file is already uploaded. The url
property is set to the URL of the file, which is used for preview.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai what is the default fileList? I still want to keep showing when a file is being downloaded, i just want to initially prefill with some files
Let me check the knowledge base for you. One moment! :books:
----------------------
The
fileList
prop in the <Upload.Dragger>
component is an array of file objects. Each file object represents a file and can have properties like uid
, name
, status
, and url
.
Here's an example of a file object:
The status
property can have the following values:
- 'uploading'
: The file is being uploaded.
- 'done'
: The file has been uploaded.
- 'error'
: An error occurred while uploading the file.
- 'removed'
: The file has been removed.
By default, the fileList
is an empty array, which means no files are being uploaded or have been uploaded.
If you want to prefill the fileList
with some files and still show when a file is being uploaded, you can manage the fileList
state in your component. Here's an example:
In this example, useState
is used to manage the state of the fileList
. The initial state of the fileList
includes one file with the status
set to 'done'
, which means the file is already uploaded. The url
property is set to the URL of the file, which is used for preview. The handleChange
function is used to update the fileList
state when new files are uploaded or existing files are removed.
For more information, refer to the Ant Design documentation (https://ant.design/components/upload/#API).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai with antd refine, is it possible to show a reference field in a list table? i have some blog posts and they have a category field, can i reference it as a link to the category item in the table?
Let me check the knowledge base for you. One moment! :books:
----------------------
Yes, it is possible to show a reference field in a list table with Ant Design in Refine. You can use the
render
prop of the <Table.Column>
component to customize how the field is displayed. In the render
function, you can return a <Link>
component that links to the category item.
Here's an example:
In this example, the render
function of the "Category" column returns a <Link>
component that links to the category item. The to
prop of the <Link>
component is set to the URL of the category item.
Please note that you need to replace /categories/${value}
with the actual path to your category item.
For more information, refer to the Refine documentation (https://refine.dev/docs/ui-integrations/ant-design/components/fields/url-field) and the Ant Design documentation (https://ant.design/components/table/).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai how could i display multiple images in a column? Maybe like a gallery? I have a
pictures
field which is an array of image urlsLet me check the knowledge base for you. One moment! :books:
----------------------
Yes, you can display multiple images in a column by using the
render
prop of the <Table.Column>
component. In the render
function, you can map over the pictures
array and return an <img>
element for each image URL.
Here's an example:
In this example, the render
function of the "Pictures" column maps over the pictures
array and returns an <img>
element for each image URL. The src
prop of the <img>
element is set to the image URL.
Please note that you need to replace width: 50, height: 50
with the actual width and height you want for the images.
For more information, refer to the Refine documentation (https://refine.dev/docs/ui-integrations/ant-design/components/fields/url-field) and the Ant Design documentation (https://ant.design/components/table/).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai i am trying to implement an import functionality, but i want to customize what happens to the data that was imported. how can i do this?
Let me check the knowledge base for you. One moment! :books:
----------------------
In Refine, you can customize the process of handling imported data using the
mapData
option in the useImport
hook. This is particularly useful when your data includes relational data and references to other data, or when your backend API requires a specific data format.
Here's an example of how you can use the mapData
option:
In this example, the mapData
function takes an item from the imported data and returns a new object that conforms to the API requirements. The categoryId
and userId
fields are mapped to category.id
and user.id
, respectively.
For more information, refer to the Refine documentation (https://refine.dev/docs/ui-integrations/ant-design/hooks/use-import#handling-relational-data).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai what i mean is that I already map the data like this. However it automatically tries to call the
create
method of my rest provider. How can i override that action?Let me check the knowledge base for you. One moment! :books:
----------------------
In Refine, the
useImport
hook automatically calls the create
or createMany
method of your data provider for each row in the imported file. If you want to override this behavior, you can create a custom hook that wraps the useImport
hook and provides your own implementation for handling the imported data.
Here's an example of how you can create a custom hook:
In this example, the useCustomImport
hook wraps the useImport
hook. The onImported
option is used to provide a callback that is called after the data is imported and mapped. In the callback, you can handle the imported data as needed. For example, you can call your own mutation.
Please note that this is a basic example and you might need to adjust it to fit your needs.
For more information, refer to the Refine documentation (https://refine.dev/docs/core/hooks/utilities/use-import).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai
onImported
does not exist. How can i prevent the api call from happening?Let me check the knowledge base for you. One moment! :books:
----------------------
I apologize for the confusion. You're correct, there's no
onImported
option in the useImport
hook.
The useImport
hook in Refine automatically calls the create
or createMany
method of your data provider for each row in the imported file. Currently, there's no built-in way to override this behavior directly within the useImport
hook.
However, you can create a custom import button and handle the file upload manually. Here's an example:
In this example, the CustomImportButton
component includes a hidden file input and a button. When the button is clicked, it triggers a click on the file input, which opens the file picker. When a file is selected, the handleFileChange
function is called. This function reads the file, parses the data, maps the data as needed, and then calls a mutation to handle the imported data.
Please note that this is a basic example and you might need to adjust it to fit your needs. For example, you might need to adjust the file reading and data parsing code depending on the format of your imported file.
For more information, refer to the Refine documentation (https://refine.dev/docs/core/hooks/utilities/use-import) and the React documentation (https://reactjs.org/docs/uncontrolled-components.html#the-file-input-tag).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai i want to read a csv file
Let me check the knowledge base for you. One moment! :books:
----------------------
You can read a CSV file in a React application using the FileReader API. Here's an example of how you can do this:
In this example, a file input is used to select the CSV file. When a file is selected, the
handleOnChange
function is called, which sets the selected file in the state. When the form is submitted, the handleOnSubmit
function is called, which reads the file as text and logs the output to the console.
Please note that this is a basic example and you might need to adjust it to fit your needs. For example, you might want to parse the CSV data into a more usable format.
For more information, refer to the Refine documentation (https://refine.dev/blog/how-to-import-csv#example).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai i am using a
parent
in my resources, to put multiple sidebar links under the same parent. How can i set parent icon?Let me check the knowledge base for you. One moment! :books:
----------------------
To set an icon for a parent resource in Refine, you can use the
meta
property in the resource definition. The meta
property allows you to store additional information about the resource, including the icon.
Here's an example of how you can set an icon for a parent resource:
In this example, the meta
property of the "CMS" resource includes an icon
property, which is set to the FileOutlined
icon from Ant Design. The "posts" and "categories" resources are nested under the "CMS" parent resource using the meta.parent
property.
For more information, refer to the Refine documentation (https://refine.dev/docs/advanced-tutorials/multi-level-menu#usage) and the Ant Design Icons documentation (https://ant.design/components/icon/).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------genetic-orange•3mo ago
@kapa.ai in antd table i want to add a total count to a table,, but i don't need the pagination buttons, how to do this?
Let me check the knowledge base for you. One moment! :books:
----------------------
To add a total count to an Ant Design table without displaying the pagination buttons, you can set the
pagination
prop to false
and use the footer
prop to display the total count.
Here's an example:
In this example:
- The pagination
prop is set to false
to disable the pagination buttons.
- The footer
prop is used to display the total count of the data. The footer
prop accepts a function that returns a React node, which in this case is a string showing the total count.
For more information, refer to the Ant Design Table documentation (https://ant.design/components/table/#Table).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•3mo ago
@kapa.ai in antd table i want to show the total count, but i don't want the pagination, can i still use the pagination prop or should i do custom?
Let me check the knowledge base for you. One moment! :books:
----------------------
To show the total count in an Ant Design table without displaying the pagination buttons, you can still use the
pagination
prop but customize it to hide the pagination controls. You can achieve this by setting the pagination
prop to an object with pageSize
set to a large number and hideOnSinglePage
set to true
.
Here's an example:
In this example:
- The pagination
prop is set to an object with pageSize
set to the length of the dataSource
to ensure all data is displayed on a single page.
- The hideOnSinglePage
property is set to true
to hide the pagination controls when there's only one page.
- The footer
prop is used to display the total count of the data.
For more information, refer to the Ant Design Table documentation (https://ant.design/components/table/#Table).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below
genetic-orange•2mo ago
@kapa.ai how can i scroll antd table programatically when a new item gets added?
Let me check the knowledge base for you. One moment! :books:
----------------------
To scroll an Ant Design table programmatically when a new item gets added, you can use the
scrollTo
method of the table's container. Here's a step-by-step guide on how to achieve this:
1. Get a reference to the table container: Use the ref
attribute to get a reference to the table container.
2. Scroll to the bottom: Use the scrollTo
method to scroll to the bottom of the table when a new item is added.
Here's an example:
In this example:
- The tableRef
is used to get a reference to the table container.
- The addItem
function adds a new item to the dataSource
and then scrolls to the bottom of the table using the scrollTo
method.
- The setTimeout
function is used to ensure that the scroll action happens after the new item is rendered.
For more information, refer to the Ant Design Table documentation (https://ant.design/components/table/).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@680514070167683118> kapa.ai is still learning and improving, please let us know how it did by reacting below