how to get route component to react to sidebar expansion?
Depending on the components on the page, the route, including the header, takes the full width of the page and the sidebar shows up correctly, but has a width of 0. I'm confused and annoyed.
See how the sidebar is in front of the route and header in this case... it's annoying. It doesn't do that for other pages with some standard mui content. If I remove the table on this page, it works too.
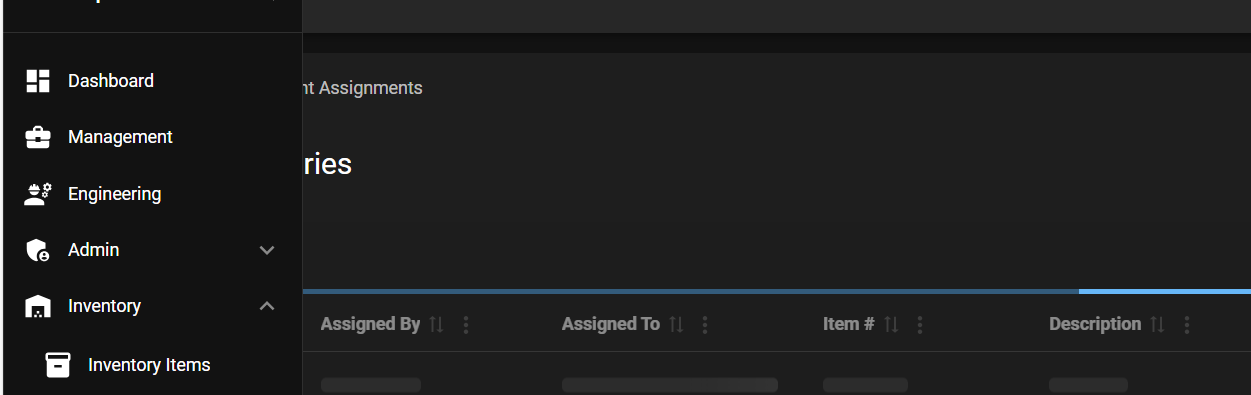
3 Replies
Hi @nerdykeystr0ke23. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
To address the issue of the sidebar not expanding correctly and overlapping with the route and header, you can use the
useThemedLayoutContext
hook provided by refine. This hook allows you to control the collapsed state of the Sider
component, ensuring that it behaves as expected when toggled.
Here's an example of how you can use the useThemedLayoutContext
hook to manage the sidebar's state:
In this example, the useThemedLayoutContext
hook is used to manage the state of the Sider
component. The DashboardPage
component includes buttons to toggle the sidebar's collapsed state and the mobile sidebar's open state. This ensures that the sidebar behaves correctly and does not overlap with the route and header.
For more details, you can refer to the collapse/uncollapse Sider
component with useThemedLayoutContext
hook (https://refine.dev/docs/ui-integrations/ant-design/components/themed-layout/#collapseuncollapse-sider-component-with-usethemedlayoutcontext-hook) documentation.
If you need further customization or if the issue persists, please provide more details about your specific setup and components.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@506691400570503191> kapa.ai is still learning and improving, please let us know how it did by reacting below
relaxed-coralOP•11mo ago
I found a solution. Not exactly sure why it works and i think it's something that's not a bug but might need to be considered a bug for ease of use of the software.
Wrap the ThemedSider with a div with style display block. It works for all of my pages, not sure if thats a full fledged solution
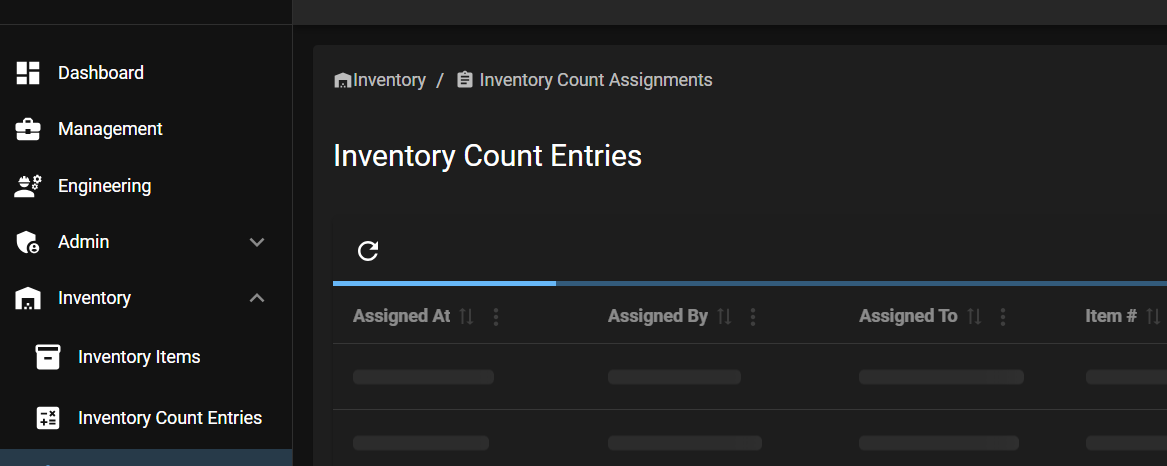