Fetch menu items
I'm struggling to create menu items dynamically via our API. Is there any specific way to handle this case?
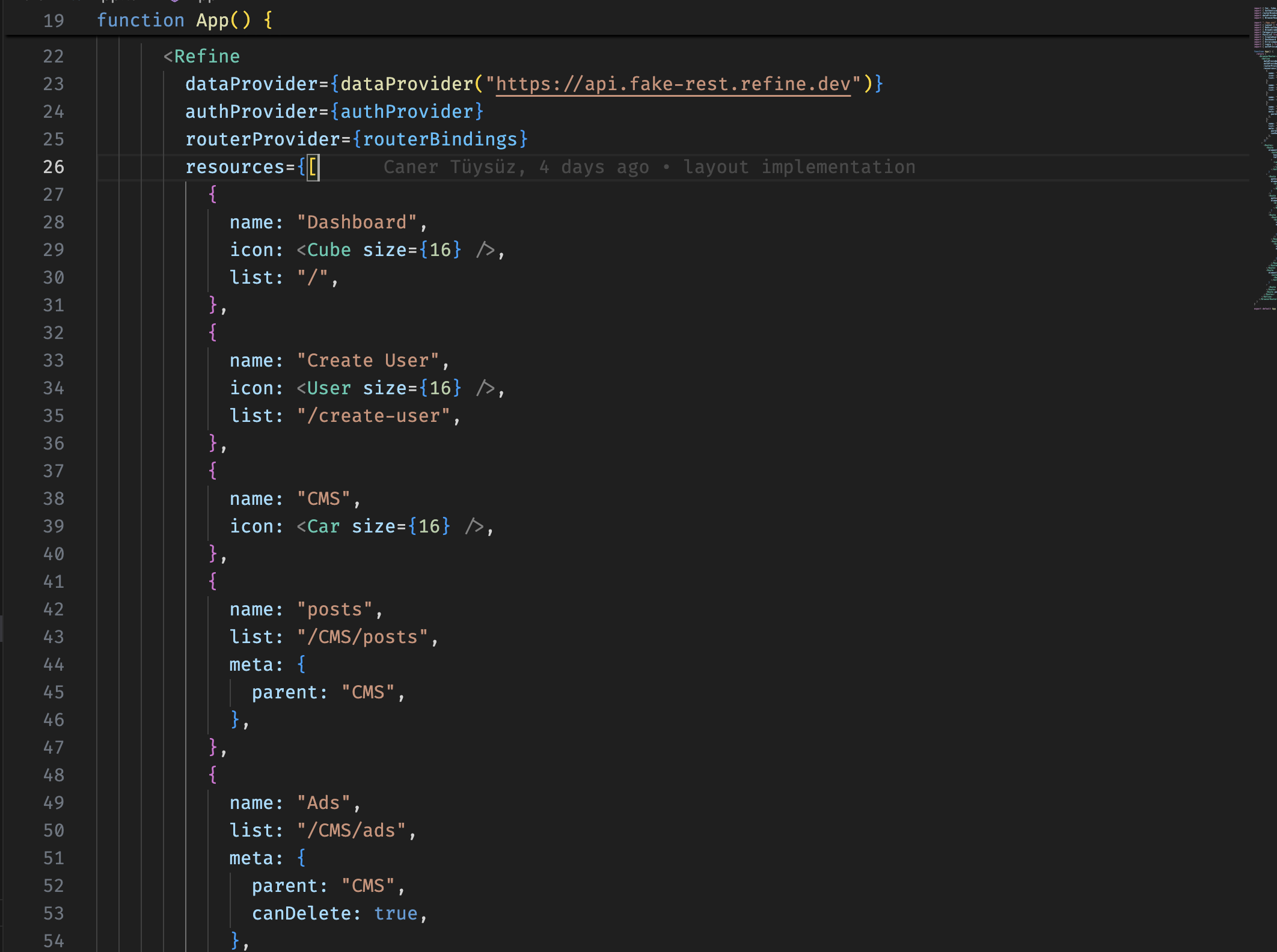
2 Replies
Hi @ctsz. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
To dynamically create menu items via your API in a Refine application, you can use the
useMenu
hook. This hook allows you to fetch and render menu items based on the resources defined in your <Refine>
component. Here’s a step-by-step guide to help you achieve this:
1. Set Up Your Resources: Ensure that your resources are defined in the <Refine>
component. These resources will be used to generate the menu items.
2. Use the useMenu
Hook: This hook will fetch the menu items based on the resources. You can then map these items to create your custom menu.
3. Fetch Data from API: If you need to fetch additional data from your API to create a dynamic secondary menu, you can do so within your component and then integrate it with the useMenu
hook.
Here’s an example of how you can create a custom menu using the useMenu
hook:
In this example:
- The useMenu
hook is used to get the initial menu items based on the resources.
- An additional API call is made to fetch dynamic menu items.
- Both static and dynamic menu items are rendered together.
For more details, you can refer to the [useMenu documentation](https://
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1266918101761658942> kapa.ai is still learning and improving, please let us know how it did by reacting below