Create Company
The image shows how my Create page looks, but it should not look like that
The Code: import React from 'react'
import { CompanyList } from './list'
import { Form, Input, Modal } from 'antd'
import { Create, useModalForm } from '@refinedev/antd'
import { useGo } from '@refinedev/core'
import { CREATE_COMPANY_MUTATION } from '@/graphql/mutations'
const create = () => {
const go = useGo();
const goToListPage = () => {
go({
to: { resource: 'companies', action: 'list' },
options: { keepQuery: true },
type: 'replace',
});
};
const { formProps, modalProps } = useModalForm({
action: 'create',
resource: 'companies',
redirect: false,
mutationMode: 'pessimistic',
onMutationSuccess: goToListPage,
meta: {
gqlMutation: CREATE_COMPANY_MUTATION,
},
});
return( <CompanyList> <Modal {...modalProps} mask = {true} onCancel = {goToListPage} title = "Erstelle ein neues Unternehmen" width = {512} > <Form {...formProps} layout = "vertical"> <Form.Item label = "Comany Name" name = "name" rules = {[{required:true}]} > <Input placeholder = "Please enter a Company Name" /> </Form.Item> </Form>
</Modal> </CompanyList> ) } export default Create
return( <CompanyList> <Modal {...modalProps} mask = {true} onCancel = {goToListPage} title = "Erstelle ein neues Unternehmen" width = {512} > <Form {...formProps} layout = "vertical"> <Form.Item label = "Comany Name" name = "name" rules = {[{required:true}]} > <Input placeholder = "Please enter a Company Name" /> </Form.Item> </Form>
</Modal> </CompanyList> ) } export default Create
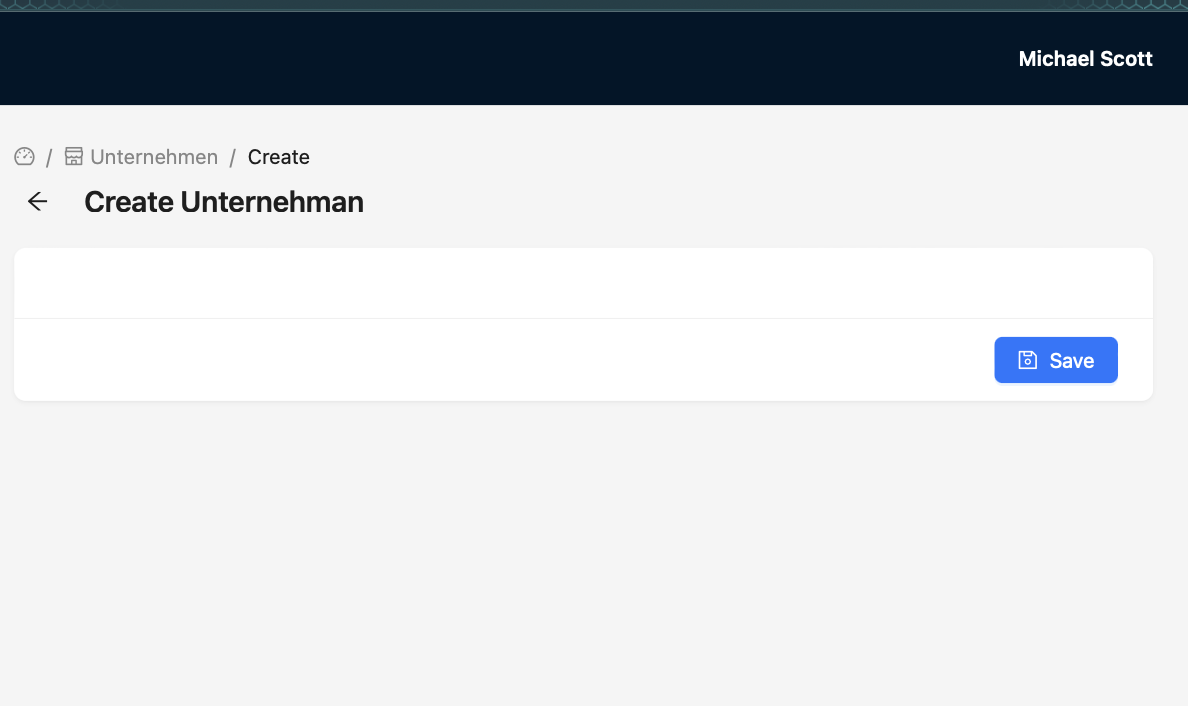
11 Replies
Hi @Yasin1012. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
It looks like you are trying to create a "Create Company" page using Refine and Ant Design. Based on the provided code and the knowledge sources, I can see that you might be missing some fields and configurations that are typically included in a "Create Company" form.
Here is an example of a more comprehensive "Create Company" form based on the knowledge sources:
``typescript
import React from 'react';
import { CompanyList } from './list';
import { Form, Input, Modal, Upload, Grid } from 'antd';
import { Create, useModalForm } from '@refinedev/antd';
import { useGo, useApiUrl } from '@refinedev/core';
import { CREATE_COMPANY_MUTATION } from '@/graphql/mutations';
import { useStrapiUpload, getValueProps, mediaUploadMapper } from '@refinedev/strapi-v4';
import { TOKEN_KEY } from '../../constants';
const CreateCompany: React.FC = () => {
const go = useGo();
const API_URL = useApiUrl();
const breakpoint = Grid.useBreakpoint();
const { ...uploadProps } = useStrapiUpload({ maxCount: 1 });
const goToListPage = () => {
go({
to: { resource: 'companies', action: 'list' },
options: { keepQuery: true },
type: 'replace',
});
};
const { formProps, modalProps } = useModalForm({
action: 'create',
resource: 'companies',
redirect: false,
mutationMode: 'pessimistic',
onMutationSuccess: goToListPage,
meta: {
gqlMutation: CREATE_COMPANY_MUTATION,
},
});
return (
<CompanyList>
<Modal
{...modalProps}
mask={true}
onCancel={goToListPage}
title="Erstelle ein neues Unternehmen"
width={breakpoint.sm ? "600px" : "80%"}
>
<Form
{...formProps}
layout="vertical"
onFinish={(values) => {
return formProps.onFinish?.({
...mediaUploadMapper(values),
});
}}
>
<Form.Item
label="Company Name"
name="name"
rules={[{ required: true }]}
>
<Input placeholder="Please enter a Company Name" />
</Form.Item>
<Form.Item label="Company Address" name="address">
<Input />
</Form.Item>
<Form.Item label="Company Country" name="country">
<Input />
</Form.Item>
<Form.Item label="Company City" name="city">
<Input />
</Form.Item>
<Form.Item
label="Email"
name="email"
rules={[{ required: true, type: "email" }]}
>
<Input />
</Form.Item>
<Form.Item label="Website" name="website">
<Input />
</Form.Item>
<Form.Item label="Company Logo">
<Form.Item
name={"logo"}
valuePropName="fileList"
getValueProps={(data) => getValueProps(data, API_URL)}
noStyle
rules={[{ required: true }]}
>
<Upload.Dragger
name="files"
action={
${API_URL}/upload}
headers={{
Authorization:
Bearer ${localStorage.getItem(TOKEN_KEY)}`,
}}
listType="picture"
multiple
{...uploadProps}
>
<p className="ant-upload-text">Drag & drop a file in this area</p>
</
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@866038216879374378> kapa.ai is still learning and improving, please let us know how it did by reacting below
unwilling-turquoiseOP•9mo ago
@kapa.ai give me complete code
Let me check the knowledge base for you. One moment! :books:
----------------------
Based on the knowledge sources and your provided code, here is a complete example of a "Create Company" page using Refine and Ant Design. This example includes additional fields and configurations to ensure a comprehensive form.
``typescript
import React from 'react';
import { CompanyList } from './list';
import { Form, Input, Modal, Upload, Grid } from 'antd';
import { Create, useModalForm } from '@refinedev/antd';
import { useGo, useApiUrl } from '@refinedev/core';
import { CREATE_COMPANY_MUTATION } from '@/graphql/mutations';
import { useStrapiUpload, getValueProps, mediaUploadMapper } from '@refinedev/strapi-v4';
import { TOKEN_KEY } from '../../constants';
const CreateCompany: React.FC = () => {
const go = useGo();
const API_URL = useApiUrl();
const breakpoint = Grid.useBreakpoint();
const { ...uploadProps } = useStrapiUpload({ maxCount: 1 });
const goToListPage = () => {
go({
to: { resource: 'companies', action: 'list' },
options: { keepQuery: true },
type: 'replace',
});
};
const { formProps, modalProps } = useModalForm({
action: 'create',
resource: 'companies',
redirect: false,
mutationMode: 'pessimistic',
onMutationSuccess: goToListPage,
meta: {
gqlMutation: CREATE_COMPANY_MUTATION,
},
});
return (
<CompanyList>
<Modal
{...modalProps}
mask={true}
onCancel={goToListPage}
title="Erstelle ein neues Unternehmen"
width={breakpoint.sm ? "600px" : "80%"}
>
<Form
{...formProps}
layout="vertical"
onFinish={(values) => {
return formProps.onFinish?.({
...mediaUploadMapper(values),
});
}}
>
<Form.Item
label="Company Name"
name="name"
rules={[{ required: true }]}
>
<Input placeholder="Please enter a Company Name" />
</Form.Item>
<Form.Item label="Company Address" name="address">
<Input />
</Form.Item>
<Form.Item label="Company Country" name="country">
<Input />
</Form.Item>
<Form.Item label="Company City" name="city">
<Input />
</Form.Item>
<Form.Item
label="Email"
name="email"
rules={[{ required: true, type: "email" }]}
>
<Input />
</Form.Item>
<Form.Item label="Website" name="website">
<Input />
</Form.Item>
<Form.Item label="Company Logo">
<Form.Item
name={"logo"}
valuePropName="fileList"
getValueProps={(data) => getValueProps(data, API_URL)}
noStyle
rules={[{ required: true }]}
>
<Upload.Dragger
name="files"
action={
${API_URL}/upload}
headers={{
Authorization:
Bearer ${localStorage.getItem(TOKEN_KEY)}`,
}}
listType="picture"
multiple
{...uploadProps}
>
<p className="ant-upload-text">Drag & drop a file in this area</p>
</Upload.Dragger>
</Form.Item>
</Form.Item>
</Form>
</Modal>
</CompanyList>
);
};
export
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@866038216879374378> kapa.ai is still learning and improving, please let us know how it did by reacting below
unwilling-turquoiseOP•9mo ago
@kapa.ai that is not my problem i am shown a complety diffrent page where could the mistake be
Let me check the knowledge base for you. One moment! :books:
----------------------
It seems like the issue might be related to how the
CreateCompany
component is being rendered or how the modal is being triggered. Let's go through a few potential issues and solutions:
1. Ensure Correct Component Export and Import:
Make sure that the CreateCompany
component is correctly exported and imported in your application.
2. Check Routing Configuration:
Ensure that the routing configuration in your application correctly points to the CreateCompany
component.
3. Verify Modal Trigger:
Ensure that the modal is being correctly triggered. If you are using a button or link to open the modal, make sure it is correctly set up.
4. Check for Errors in Console:
Open the browser's developer console and check for any errors that might give you more information about what is going wrong.
If none of these solutions resolve the issue, please provide more details or screenshots of the problem you are encountering
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@866038216879374378> kapa.ai is still learning and improving, please let us know how it did by reacting below
unwilling-turquoiseOP•9mo ago
@kapa.ai How can I Open the browser's developer console
Let me check the knowledge base for you. One moment! :books:
----------------------
To open the browser's developer console, follow the steps below based on the browser you are using:
Google Chrome
1. Keyboard Shortcut:
- Press
Ctrl + Shift + I
(Windows/Linux) or Cmd + Option + I
(Mac).
- Alternatively, you can press F12
.
2. Using the Menu:
- Click on the three vertical dots in the upper-right corner of the browser window.
- Navigate to More tools
> Developer tools
.
Mozilla Firefox
1. Keyboard Shortcut:
- Press Ctrl + Shift + I
(Windows/Linux) or Cmd + Option + I
(Mac).
- Alternatively, you can press F12
.
2. Using the Menu:
- Click on the three horizontal lines in the upper-right corner of the browser window.
- Navigate to Web Developer
> Toggle Tools
.
Microsoft Edge
1. Keyboard Shortcut:
- Press Ctrl + Shift + I
(Windows/Linux) or Cmd + Option + I
(Mac).
- Alternatively, you can press F12
.
2. Using the Menu:
- Click on the three horizontal dots in the upper-right corner of the browser window.
- Navigate to More tools
> Developer tools
.
Safari
1. Enable the Developer Menu:
- Go to Safari
> Preferences
> Advanced
.
- Check the box that says Show Develop menu in menu bar
.
2. Open the Console:
- Click on Develop
in the menu bar.
- Select Show JavaScript Console
.
Using the Console
Once the developer tools are open, you can navigate to the Console
tab to view any errors or logs that might help you debug your application.
For more detailed instructions, you can refer to the official documentation of each browser:
- Chrome DevTools (https://developer.chrome.com/docs/devtools/)
- Firefox Developer Tools (https://firefox-source-docs.mozilla.org/devtools-user/)
- Microsoft Edge DevTools (https://docs.microsoft.com/en-us/microsoft-edge/devtools-guide-chromium/)
- Safari Web Inspector Guide (https://developer.apple.com/safari/tools/)
By using the developer console, you can inspect elements, view console logs, and debug JavaScript errors, which can be very helpful in identifying issues with your application.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@866038216879374378> kapa.ai is still learning and improving, please let us know how it did by reacting below