Saving
On strapi4 we have the createdAt field. How to use the DatePicker to save a different date in this field instead of the current date
92 Replies
Hi @Sly. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
To use a DatePicker to save a different date in the
createdAt
field when using Strapi v4 with refine, you can follow these steps:
1. Use the <DatePicker />
component from Ant Design in your form.
2. Set up the form field to use the DatePicker and map its value correctly.
Here's an example of how you can implement this:
This code snippet uses the getValueProps
prop to properly format the date value for the DatePicker DatePicker example (https://github.com/refinedev/refine/issues/1813#issuecomment-1183671046).
When submitting the form, the selected date will be sent to Strapi v4 as the createdAt
value, overriding the default current date.
Remember to import the necessary components and libraries:
Note that you may need to configure your Strapi v4 backend to allow overriding the createdAt
field, as by default it might be set automatically.
Strapi v4 Integration (https://github.com/refinedev/refine/issues/1274#issuecomment-1049723659) is supported in refine, so make sure you're using the correct data provider for Strapi v4.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai how to display more than 10 records using useList
Let me check the knowledge base for you again. One moment! :books:
----------------------
To display more than 10 records using the
useList
hook in refine, you can adjust the pageSize
parameter in the pagination
option. Here's how you can do it:
In this example, I've set pageSize
to 20, which means it will fetch and display up to 20 records. You can adjust this number based on your requirements.
The useList
hook is an extended version of TanStack Query's useQuery
that supports pagination, filtering, and sorting useList documentation (https://refine.dev/docs/data/hooks/use-list/).
Here's a more complete example of how you might use this in a component:
Remember that the actual number of records returned may depend on your backend implementation. The useList
hook passes the pagination
property to the getList
method from the dataProvider
, so make sure your data provider and backend support fetching the desired number of records Pagination documentation (https://refine.dev/docs/data/hooks/use-list/#pagination).
If you need to display even more records or implement infinite scrolling, you might want to consider using the useInfiniteList
hook instead useInfiniteList documentation (https://refine.dev/docs/data/hooks/use-infinite-list/#example).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai How to use EditButton to open edit page of another resorce
Let me check the knowledge base for you again. One moment! :books:
----------------------
To use the
EditButton
to open the edit page of another resource in refine, you can utilize the resource
prop along with the recordItemId
prop. Here's how you can do it:
In this example, clicking the button will redirect to the edit page of the "categories" resource with the record ID of "2" Mantine UI EditButton (https://refine.dev/docs/ui-integrations/mantine/components/buttons/edit-button/#resource).
The resource
prop allows you to specify which resource's edit page you want to navigate to. By default, the EditButton
uses the inferred resource from the current route, but you can override this by explicitly setting the resource
prop Ant Design EditButton (https://refine.dev/docs/ui-integrations/ant-design/components/buttons/edit-button/#resource).
Here's a more complete example of how you might use this in a component:
This setup will create an edit button that, when clicked, will navigate to the edit page of the "categories" resource for the record with ID "2" Material UI EditButton (https://refine.dev/docs/ui-integrations/material-ui/components/buttons/edit-button/#resource).
If you need to pass additional parameters to the navigation, you can use the meta
prop:
This can be useful if your edit route requires additional parameters Chakra UI EditButton (https://refine.dev/docs/ui-integrations/chakra-ui/components/buttons/edit-button/#meta).
Remember that the actual behavior of the EditButton
depends on the edit
method of the useNavigation
(https://refine.dev/docs/routing/hooks/use-navigation/) hook, which is used under the hood by the EditButton
component.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai The edit page is in modal. I want to open the edit page of another resource in my current page
Let me check the knowledge base for you again. One moment! :books:
----------------------
To open the edit page of another resource in a modal on your current page, you can use the
useModalForm
hook along with the EditButton
component in refine. Here's how you can implement this:
1. First, set up the useModalForm
hook for the resource you want to edit:
2. Then, use the EditButton
component with a custom onClick
handler to show the modal:
3. Finally, render the modal with the edit form:
Here's a more complete example of how this might look in a component:
This approach allows you to open the edit page of another resource (in this case, "categories") in a modal on your current page (which might be listing "products") useModalForm usage (https://refine.dev/docs/ui-integrations/ant-design/hooks/use-modal-form/#usage).
The useModalForm
hook handles the modal visibility and form submission, while the EditButton
is used to trigger the modal opening. The resource
prop in useModalForm
ensures that the correct resource is being edited.
Remember to adjust the interfaces (IProduct
, ICategory
) and form fields according to your specific resources and data structure.
Edit Button documentation (https://refine.dev/docs/ui-integrations/ant-design/components/buttons/edit-button/#properties)
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
How to keep the show page in the background and open the EditButton of the same resource
How to keep the show page in the background and open modal using the EditButton of the same resource
@kapa.ai How to keep the show page in the background and open modal using the EditButton of the same resource
Let me check the knowledge base for you again. One moment! :books:
----------------------
To keep the show page in the background and open a modal using the EditButton of the same resource, you can use the
useModalForm
hook along with the EditButton
component in refine. Here's how you can implement this:
1. First, set up the useModalForm
hook in your show page component:
2. Then, use the EditButton
component with a custom onClick
handler to show the modal:
3. Finally, render the modal with the edit form:
Here's a more complete example of how this might look in a show page component:
This approach allows you to keep the show page in the background while opening an edit modal for the same resource when the EditButton is clicked useModalForm usage (https://refine.dev/docs/ui-integrations/ant-design/hooks/use-modal-form/#usage).
The useModalForm
hook handles the modal visibility and form submission, while the EditButton
is used to trigger the modal opening. The show
function from useModalForm
is called with the record id when the EditButton is clicked, which opens the modal with the edit form for that specific record.
Remember to adjust the form fields according to your specific resource and data structure.
Edit Button documentation (https://refine.dev/docs/ui-integrations/ant-design/components/buttons/edit-button/#properties)
Show component documentation (https://refine.dev/docs/ui-integrations/ant-design/components/basic-views/show/#properties)
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
I put the EditButton in the view page
@kapa.ai can i use onclick to open the props Name of the page? Like this: onClick={() = <Collaboration />}
Let me check the knowledge base for you again. One moment! :books:
----------------------
I apologize, but I don't have enough information in the provided knowledge sources to directly answer your question about using
onClick
to open a component named <Collaboration />
. The specific syntax you're asking about is not covered in the refine documentation I have access to.
However, I can provide some general guidance based on refine's typical patterns:
1. In refine, navigation between pages or opening of modals is usually handled through hooks like useNavigation
or useModalForm
, rather than directly rendering components in onClick
handlers useModalForm usage (https://refine.dev/docs/ui-integrations/ant-design/hooks/use-modal-form/#usage).
2. If you want to open a new page or component, you might use the useNavigation
hook. For example:
3. If you want to open a modal, you would typically use the useModalForm
hook as demonstrated in previous examples useModalForm usage (https://refine.dev/docs/ui-integrations/ant-design/hooks/use-modal-form/#usage).
4. Directly rendering a component inside an onClick
handler (like onClick={() => <Collaboration />}
) is not a typical pattern in React or refine, and likely wouldn't work as expected.
If you need to implement a specific behavior with the Collaboration
component, I'd recommend reviewing refine's documentation on routing and navigation, or considering whether a modal might be more appropriate for your use case. If you need more specific guidance, please provide more context about what you're trying to achieve with the Collaboration
component.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai it's to open the edit page
Let me check the knowledge base for you again. One moment! :books:
----------------------
I understand you want to open the edit page. In refine, there are specific ways to handle navigation to edit pages. Let's look at how you can achieve this using refine's built-in components and hooks.
To open the edit page for a resource, you can use the
EditButton
component or the edit
function from the useNavigation
hook. Here are two approaches you can use:
1. Using the EditButton
component:
This will create a button that, when clicked, will navigate to the edit page of the "posts" resource for the specific record Mantine UI EditButton (https://refine.dev/docs/ui-integrations/mantine/components/buttons/edit-button/#resource).
2. Using the useNavigation
hook:
This approach gives you more flexibility in terms of the UI, as you can use any component to trigger the navigation useNavigation hook (https://refine.dev/docs/routing/hooks/use-navigation/#edit).
Both of these methods will navigate to the edit page of the specified resource. The exact URL will depend on your routing configuration, but it typically follows the pattern /posts/edit/:id
.
Remember, you need to have the edit page component defined and properly routed in your application for these navigation methods to work correctly.
If you're trying to open the edit page in a modal while keeping the current page in the background, you can use the useModalForm
hook as described in the previous answer.
Is there a specific aspect of opening the edit page you're having trouble with?
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai inLoading in use one is always appear "false"
Let me check the knowledge base for you again. One moment! :books:
----------------------
I understand you're having an issue with the
isLoading
state in the useOne
hook always appearing as "false". This behavior is actually expected in certain scenarios. Let me explain why this might be happening and how you can work with it.
According to the refine documentation:
useOne documentation (https://refine.dev/docs/data/hooks/use-show/#queryresult):
The isLoading
state is false initially, before the query mounts. It becomes true when the query is mounted (not necessarily when the fetch occurs, as it might be disabled). Once isLoading
is true, it never goes back to false. This is different from isFetching
.
If you need to show a loading state while data is being fetched, you should use the isFetching
property instead. Here's an example of how you might use it:
If you're specifically using the useShow
hook (which is an extended version of useOne
), the same principle applies:
Remember, isLoading
is more about the overall state of the query, while isFetching
indicates whether the query is currently fetching data, including background refetches.
If you're still experiencing issues after using isFetching
, it might be worth checking your data provider implementation or network requests to ensure data is being fetched as expected.
Github Issue Discussion (https://github.com/refinedev/refine/issues/5793)
Let me know if you need any further clarification or have any other questions!
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
How to Use Skeleton globally instead of Spin
@kapa.ai How to Use Skeleton globally instead of Spin
Let me check the knowledge base for you again. One moment! :books:
----------------------
To use Skeleton globally instead of Spin in refine, you can customize the default loading component. Here's how you can achieve this:
1. First, create a custom Skeleton component:
2. Then, use the
LoadingComponent
prop in the <Refine>
component to set your custom Skeleton as the global loading component:
By setting the LoadingComponent
prop, you're telling refine to use your CustomSkeleton component wherever it would normally use the default Spin component Refine component documentation (https://refine.dev/docs/core/refine-component/#loadingcomponent).
This approach will apply the Skeleton loading indicator globally across your refine application. However, keep in mind that this will only affect the loading states managed by refine itself. For component-specific loading states, you might still need to replace Spin with Skeleton manually.
For example, in a List component, you might do:
Remember to import the Skeleton component from the appropriate package based on your UI framework (e.g., "@refinedev/antd" for Ant Design) Ant Design Skeleton (https://refine.dev/docs/ui-integrations/ant-design/components/basic-views/show/#properties).
By following these steps, you can effectively use Skeleton globally instead of Spin in your refine application.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai go to login page when reopen Browser
Let me check the knowledge base for you again. One moment! :books:
----------------------
To handle the scenario where you want to redirect users to the login page when they reopen the browser in a refine application, you need to implement this behavior in your
authProvider
. Specifically, you'll need to focus on the check
method of the authProvider
.
Here's how you can implement this:
1. In your authProvider
, modify the check
method to verify if the user's session is still valid:
In this example, the check
method looks for a token in localStorage. If it finds one, it verifies if the token is still valid (you'll need to implement the verifyToken
function based on your authentication system). If the token is invalid or doesn't exist, it returns an object that tells refine to redirect to the login page Auth Provider documentation (https://refine.dev/docs/guides-concepts/usage-with-existing-projects/#check-method).
2. Make sure you've set up your routes correctly, wrapping authenticated routes with the <Authenticated>
component:
This setup ensures that when a user reopens the browser and tries to access an authenticated route, the check
method in your authProvider
will be called. If it returns authenticated: false
, the user will be redirected to the login page Routing documentation (https://refine.dev/docs/routing/integrations/react-router/#how-to-handle-optional-authentication-redirects-and-layouts-with-authentication).
Remember to adjust the token storage and verification logic according to your specific authentication system. The key is to implement a robust check
method in your authProvider
that accurately determines if the user's session is still valid when the browser is reopened.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai I'm using strapi4
Let me check the knowledge base for you again. One moment! :books:
----------------------
I understand you're using Strapi v4 with refine. When working with Strapi v4 and refine, there are some specific configurations and methods you can use to handle authentication and session management. Let's adapt the previous answer to work with Strapi v4.
For Strapi v4 integration with refine, you can use the
AuthHelper
from @refinedev/strapi-v4
. Here's how you can modify your authProvider
to work with Strapi v4:
This setup uses the AuthHelper
from @refinedev/strapi-v4
to handle login and token management Strapi v4 Integration (https://refine.dev/blog/refine-react-admin-invoice-generator/#usage).
To ensure that users are redirected to the login page when reopening the browser, the check
method verifies if a valid token exists in localStorage. If not, it returns authenticated: false
, which will trigger a redirect to the login page.
Remember to configure your Refine app to use this authProvider
:
This setup will ensure that when a user reopens the browser, the check
method in your authProvider
will be called. If no valid token is found, the user will be redirected to the login page Refine with Strapi v4 (https://refine.dev/blog/refine-react-admin-invoice-generator/#usage).
Remember to replace "Your_Strapi_Url"
with your actual Strapi v4 API URL.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai when I close the browser without login out and reopen the browser it passes the login page
Let me check the knowledge base for you again. One moment! :books:
----------------------
Based on your description, it seems that the authentication state is persisting even after closing and reopening the browser. This behavior is likely due to how the
check
method in your authProvider
is implemented when using Strapi v4 with refine.
To address this issue and ensure that users are redirected to the login page when reopening the browser, you can modify the check
method in your authProvider
. Here's an improved version:
In this improved version, we're not only checking for the presence of a token but also verifying its validity with Strapi using the strapiAuthHelper.me()
method Strapi v4 Integration (https://refine.dev/blog/refine-react-admin-invoice-generator/#usage). This ensures that:
1. If no token is found, the user is redirected to the login page.
2. If a token is found, its validity is checked with Strapi.
3. If the token is invalid or expired, it's removed from localStorage, and the user is redirected to the login page.
4. Only if the token is valid will the user remain authenticated.
This approach should resolve the issue of bypassing the login page when reopening the browser. It adds an extra layer of security by verifying the token's validity with your Strapi backend.
Remember to implement this authProvider
in your Refine app as shown in the previous response:
This setup ensures that the authentication state is properly checked each time the app is loaded, including when the browser is reopened Refine with Strapi v4 (https://refine.dev/blog/refine-react-admin-invoice-generator/#usage).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai how to console.log inside this
Let me check the knowledge base for you again. One moment! :books:
----------------------
To add console.log statements inside the
check
method of your authProvider
when using Strapi v4 with refine, you can modify the code as follows:
This modified version includes several console.log
statements that will help you track the flow of the check
method and see the values of important variables at each step Strapi v4 Integration (https://refine.dev/blog/refine-react-admin-invoice-generator/#usage).
Remember to open your browser's developer tools (usually by pressing F12) and look at the Console tab to see these log messages. This will help you understand what's happening during the authentication check process and can be useful for debugging any issues you're experiencing.
Keep in mind that it's generally a good practice to remove or disable these console.log
statements in production code, as they can potentially expose sensitive information and affect performance.
Auth Provider documentation (https://refine.dev/docs/authentication/auth-provider/#check)
</assistant_answer>
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai give me another simple way to use session
Let me check the knowledge base for you again. One moment! :books:
----------------------
To provide a simpler way to use session management in refine with Strapi v4, we can utilize the browser's built-in sessionStorage instead of localStorage. This approach will automatically clear the session when the browser is closed, addressing your previous concern about persisting login state across browser sessions. Here's how you can implement this:
1. First, modify your
authProvider
to use sessionStorage:
This simplified approach uses sessionStorage
instead of localStorage
Auth Provider documentation (https://refine.dev/docs/authentication/auth-provider/#check). The main differences are:
1. We use sessionStorage.setItem()
and sessionStorage.getItem()
instead of their localStorage
counterparts.
2. We don't need to explicitly verify the token on each check, as the session will be automatically cleared when the browser is closed.
This method provides a simpler way to manage sessions and ensures that users will need to log in again when they reopen the browser, addressing your previous concern.
Remember to use this authProvider
in your Refine app setup:
This setup provides a simple and effective way to manage user sessions in your refine application with Strapi v4 Refine with Strapi v4 (https://refine.dev/blog/refine-react-admin-invoice-generator/#usage).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai how render all itens of the menu once they are all fecthed
Let me check the knowledge base for you again. One moment! :books:
----------------------
To render all items of the menu once they are all fetched in refine, you can use the
useMenu
hook. This hook provides the menu items based on the resources defined in your <Refine>
component. Here's how you can implement this:
1. First, create a custom Menu
component:
In this example, we use the useMenu
hook to get the menuItems
and isLoading
state useMenu documentation (https://refine.dev/docs/core/hooks/utilities/use-menu/#usage). The isLoading
state ensures that we don't render the menu until all items are fetched.
2. Use this Menu
component in your layout:
3. Make sure your resources are properly defined in the <Refine>
component:
This approach ensures that all menu items are rendered once they are fetched. The useMenu
hook handles the fetching of menu items based on your defined resources, and the isLoading
state allows you to show a loading indicator if needed Creating a Menu (https://refine.dev/docs/core/hooks/utilities/use-menu/#creating-a-menu).
Remember, if you need to customize the menu further or handle multi-level menus, the useMenu
hook provides additional functionality for these cases Multi Level Menus (https://refine.dev/docs/core/hooks/utilities/use-menu/#multi-level-menus).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai I'm using the default refine menu
Let me check the knowledge base for you again. One moment! :books:
----------------------
If you're using the default refine menu, you don't need to create a custom menu component. The default menu in refine is designed to automatically render all the menu items based on your defined resources. However, if you're experiencing issues with menu items not appearing immediately, there are a few things you can check:
1. Make sure your resources are properly defined in the
<Refine>
component:
2. If you're using the Ant Design UI package, the default <Sider>
component should handle the menu rendering automatically Ant Design Sider (https://refine.dev/docs/ui-integrations/ant-design/components/themedLayout/sider/#usage).
3. To ensure all menu items are visible, you can adjust the hideOnMissingParameter
option in the <ThemedSiderV2>
component:
This will show all menu items, even if they require parameters Themed Sider V2 (https://refine.dev/docs/ui-integrations/ant-design/components/themedLayout/sider/#hideonmissingparameter).
4. If you're still having issues with menu items not appearing, you can try to force a re-render of the menu by using the useMenu
hook in a custom component:
Then use this CustomSider
in your layout.
Remember, the default refine menu should handle the rendering of all resource items automatically. If you're still experiencing issues after trying these suggestions, it might be worth checking your resource definitions or any custom configurations that might be affecting the menu rendering.
useMenu documentation (https://refine.dev/docs/core/hooks/utilities/use-menu/#usage)
Let me know if you need any further clarification or if you're still experiencing issues with the menu rendering!
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai when I'm using access control the menus are listed slowly, I want to be listed all at once
Let me check the knowledge base for you again. One moment! :books:
----------------------
When using access control with refine, the menu items are typically checked for permissions individually, which can lead to slower rendering if you have many menu items. To improve this and list all menu items at once, you can optimize your access control implementation. Here's an approach you can take:
1. Modify your
accessControlProvider
to fetch all permissions at once:
2. Use the usePermissions
hook to prefetch all permissions:
3. Modify the Sider component to use the prefetched permissions:
This approach fetches all permissions at once and uses them to filter the menu items, which should result in faster rendering of the menu Access Control Provider documentation (https://refine.dev/docs/api-reference/core/providers/accessControl-provider/#overview).
Remember to replace fetchAllPermissions()
with your actual implementation to fetch permissions from your backend.
By implementing these changes, you should see all menu items listed at once, as the permissions are fetched and applied in a single operation rather than checking each item individually.
usePermissions documentation (https://refine.dev/docs/api-reference/core/hooks/accessControl/usePermissions/)
If you're still experiencing slow rendering after implementing these optimizations, you might want to consider caching the permissions on the client side or optimizing your backend's permission fetching process.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai what about use tl time?
Let me check the knowledge base for you again. One moment! :books:
----------------------
I apologize, but I don't have specific information about "tl time" in the context of refine or its menu rendering. The term "tl time" is not a standard concept in refine's documentation or typical React development practices.
However, if you're referring to optimizing the time it takes to load and render the menu items, there are a few approaches you can consider:
1. Use memoization: You can use React's
useMemo
hook to memoize the menu items, which can help prevent unnecessary re-renders:
2. Implement lazy loading: If your application has many resources, you could implement lazy loading of menu items:
3. Optimize your accessControlProvider
: Ensure that your access control checks are as efficient as possible. Consider caching permissions or fetching them in bulk as suggested in the previous answer.
useMenu documentation (https://refine.dev/docs/core/hooks/utilities/use-menu/#usage)
If you're experiencing specific performance issues with menu rendering, it might be helpful to profile your application to identify bottlenecks. React DevTools can be useful for this purpose.
Remember, the exact optimization strategy will depend on your specific use case and the structure of your application. If you have a more specific concern or if "tl time" refers to something else in your context, please provide more details so I can give you a more accurate answer.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai List all resources at once when they are all fecthed
Let me check the knowledge base for you again. One moment! :books:
----------------------
To list all resources at once when they are all fetched in refine, you can use the
useMenu
hook along with some state management to ensure all items are loaded before rendering. Here's an approach you can take:
1. Create a custom component to handle the menu rendering:
2. Use this custom component in your layout:
This approach ensures that the menu is only rendered once all items are fetched. The useMenu
hook provides the menuItems
and isLoading
state, which we use to determine when all items are ready useMenu documentation (https://refine.dev/docs/core/hooks/utilities/use-menu/#usage).
If you're using access control and want to ensure all permissions are checked before rendering the menu, you can combine this with the usePermissions
hook:
This approach ensures that both the menu items and permissions are loaded before rendering the menu, and it filters the menu items based on the user's permissions Access Control Provider documentation (https://refine.dev/docs/api-reference/core/providers/accessControl-provider/#overview).
By implementing these changes, you should see all permitted menu items listed at once, only after they have all been fetched and processed.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai How to lazy load in the file index.tsx
Let me check the knowledge base for you again. One moment! :books:
----------------------
To implement lazy loading in the
index.tsx
file of your refine application, you can use React's lazy
and Suspense
components. This approach allows you to split your code into smaller chunks and load components only when they are needed, which can significantly improve your application's initial load time.
Here's how you can implement lazy loading in your index.tsx
file:
1. First, import the necessary components from React:
2. Then, use lazy
to import your components:
3. Wrap your routes with Suspense
and provide a fallback UI:
This setup will lazy load your components, splitting them into separate chunks that are loaded only when the corresponding route is accessed React Lazy Loading (https://refine.dev/blog/react-lazy-loading/#what-is-reactlazy).
Remember to adjust the import paths according to your project structure. Also, make sure that your bundler (like webpack) is configured to support code splitting.
If you're using a custom layout, you might want to apply lazy loading there as well:
This approach allows you to lazy load entire sections of your application, further improving performance Route-based code-splitting (https://refine.dev/blog/react-lazy-loading/#route-based-code-splitting).
By implementing lazy loading in your index.tsx
file, you can significantly reduce the initial bundle size and improve the load time of your refine application.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai I'm using the default index.tsx file like the image attached
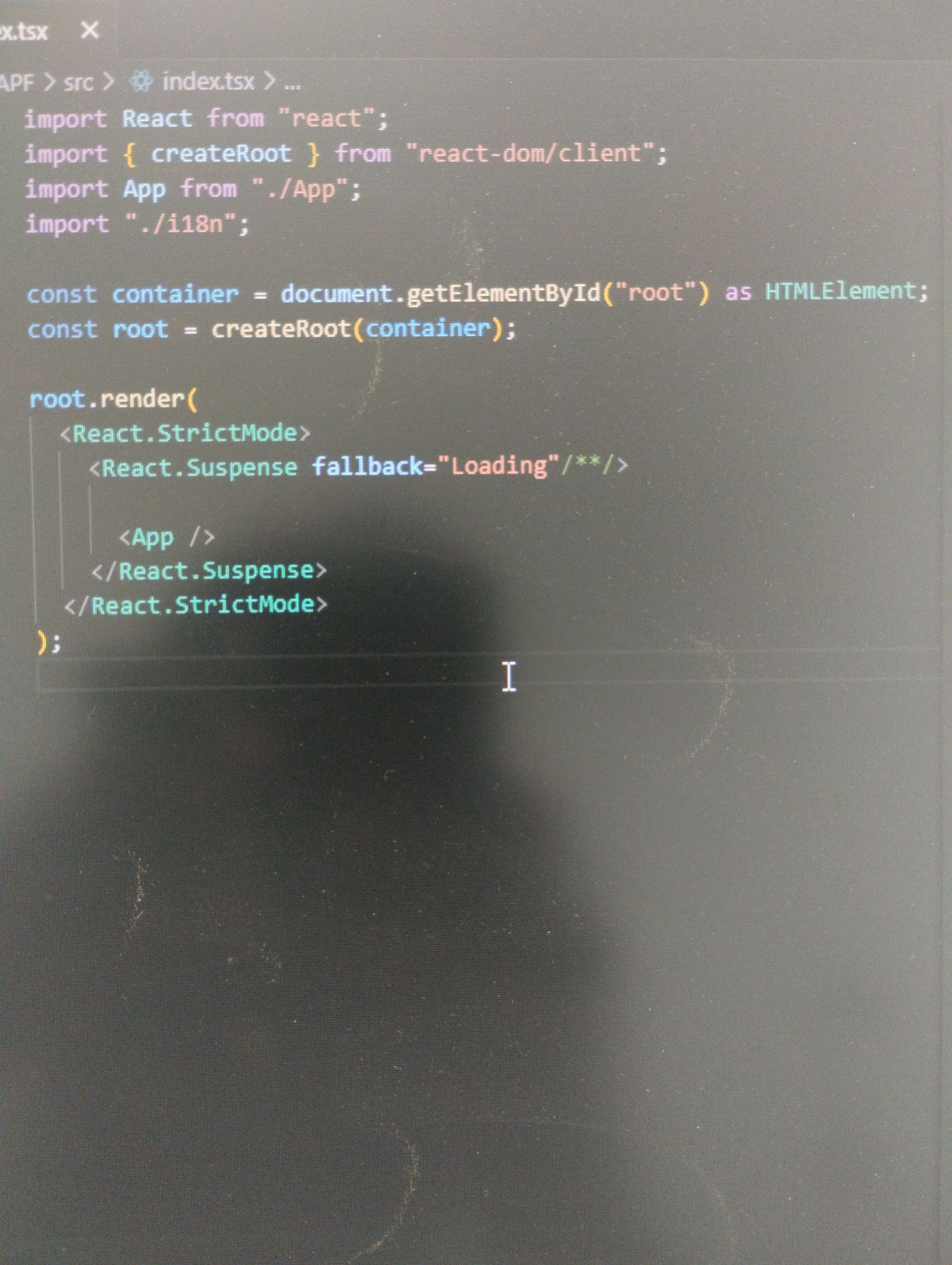
Let me check the knowledge base for you again. One moment! :books:
----------------------
I apologize, but I don't see any image attached to your question. Without seeing the specific content of your default
index.tsx
file, I can't provide a precise answer tailored to your exact setup. However, I can give you general guidance on how to implement lazy loading in a typical refine index.tsx
file.
Assuming you're using a standard refine setup, here's how you might modify your index.tsx
file to implement lazy loading:
1. First, import the necessary components:
2. Then, use lazy
to import your components:
3. Modify your App
component to use Suspense
:
This setup implements lazy loading for your route components, which can help improve your application's initial load time React Lazy Loading (https://refine.dev/blog/react-lazy-loading/#route-based-code-splitting).
If your index.tsx
file is significantly different from this example, or if you have specific requirements or constraints, please provide more details about your current setup. With more information, I can give you a more tailored solution for implementing lazy loading in your refine application.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai
Let me check the knowledge base for you again. One moment! :books:
Sorry @Sly, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?
vicious-goldOP•2mo ago
@kapa.ai how to delay for 5 seconds to render the <App />
Let me check the knowledge base for you again. One moment! :books:
----------------------
To delay rendering the
<App />
component for 5 seconds in refine, you can use React's useEffect
hook along with useState
to create a simple delay mechanism. Here's how you can implement this in your index.tsx
file:
This approach wraps your <App />
component in a DelayedApp
component that uses a 5-second timer before rendering the actual App
component. Here's what's happening:
1. We create a DelayedApp
component that manages the delay.
2. We use useState
to track whether the app is ready to render.
3. We use useEffect
to set up a timer that will set isReady
to true
after 5 seconds.
4. While isReady
is false
, we render a loading indicator.
5. Once isReady
becomes true
, we render the <App />
component.
This implementation ensures that your entire <App />
component and its children will only start rendering after the 5-second delay React 18 Upgrade Guide (https://refine.dev/blog/react-18-upgrade-guide/).
Remember that artificially delaying your app's render like this might not be ideal for user experience in most cases. It's generally better to show content as soon as possible and use loading indicators only for specific parts of your app that are actually loading data or resources.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai how to show loading indicators in the index.tsx
Let me check the knowledge base for you again. One moment! :books:
----------------------
To show loading indicators in the
index.tsx
file of your refine application, you can use React's Suspense
component along with a custom loading component. Here's how you can implement this:
1. First, create a custom loading component:
2. Then, in your index.tsx
file, wrap your <App />
component with Suspense
and use the LoadingComponent
as the fallback:
This setup will show the LoadingComponent
while your main App
component and its dependencies are being loaded React Lazy Loading (https://refine.dev/blog/react-lazy-loading/#suspense).
If you're using a UI library like Ant Design, you can use their built-in loading components for a more polished look:
This approach provides a centered spinning loader while your application is loading Ant Design Spin (https://refine.dev/docs/ui-integrations/ant-design/components/basic-views/show/#isloading).
Remember, this loading indicator will only be shown while the initial JavaScript bundle is being loaded and parsed. For loading states within your application (like data fetching), you'll need to handle those separately within your components or use refine's built-in loading state management.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai Delay spin for 10seconds
Let me check the knowledge base for you again. One moment! :books:
----------------------
To delay the spin loading indicator for 10 seconds in your
index.tsx
file, we can combine the delayed rendering approach with the loading indicator. Here's how you can modify your index.tsx
file to achieve this:
In this implementation:
1. We create a DelayedLoadingComponent
that uses useState
and useEffect
to manage the delay.
2. The component initially renders nothing (return null
) for the first 10 seconds.
3. After 10 seconds, it renders the Spin
component from Ant Design.
4. We use this DelayedLoadingComponent
as the fallback for Suspense
.
This approach will show nothing for the first 10 seconds, and then display the spinning loader if your app is still loading after that time.
Remember that artificially delaying your loading indicator like this might not provide the best user experience in most cases. It's generally better to show loading indicators as soon as possible to give users immediate feedback that something is happening. However, if you have a specific reason for this 10-second delay, this implementation will achieve that goal.
Ant Design Spin (https://refine.dev/docs/ui-integrations/ant-design/components/basic-views/show/#isloading)
React Suspense (https://refine.dev/blog/react-lazy-loading/#suspense)
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai continue spinning untill the App is fully rendered
Let me check the knowledge base for you again. One moment! :books:
----------------------
To continue spinning until the App is fully rendered, we can modify our approach to use a combination of React's
Suspense
, a custom hook to track when the App is fully loaded, and the Ant Design Spin
component. Here's how you can implement this in your index.tsx
file:
1. First, let's create a custom hook to track when the App is fully rendered:
2. Now, let's modify the App
component to use this hook:
3. Finally, let's update the index.tsx
file to continue spinning until the App is fully rendered:
This approach ensures that:
1. The spinning loader is shown immediately when the app starts loading.
2. The App
component doesn't render its content until it's fully loaded (thanks to the useAppLoaded
hook).
3. The spinning loader continues to show until the App
component signals that it's fully loaded.
This implementation provides a smooth loading experience, showing the spinner until your entire App is ready to be displayed. It combines the Suspense
component for handling asynchronous loading with a custom hook to ensure the App doesn't render prematurely React Suspense (https://refine.dev/blog/react-suspense-guide/#what-is-react-suspense).
Remember that this approach assumes that your App
component and its children are the main source of loading time. If you have specific data fetching or other asynchronous operations within your App, you might need to handle those separately or incorporate them into your useAppLoaded
hook.
Ant Design Spin (https://refine.dev/docs/ui-integrations/ant-design/components/basic-views/show/#isloading)
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai how to console.log on the App file to check if the file is tendered or not
Let me check the knowledge base for you again. One moment! :books:
----------------------
To console.log in the App file to check if it's rendered or not, you can add a
useEffect
hook in your App component. This will allow you to log a message when the component mounts, indicating that it has been rendered. Here's how you can modify your App file:
This approach will log the message "App component has rendered" to the console as soon as the App component mounts, which indicates that it has been rendered React 18 Upgrade Guide (https://refine.dev/blog/react-18-upgrade-guide/).
If you want to log more detailed information or check for specific conditions, you can expand on this basic implementation. For example:
This more comprehensive example will log messages at different stages of the component lifecycle:
1. When the component starts rendering
2. On each re-render (showing the current state of isFullyLoaded
)
3. When the component is considered fully loaded
4. When the component is unmounting
Remember to open your browser's developer tools (usually by pressing F12) and look at the Console tab to see these log messages React Memo Guide (https://refine.dev/blog/react-memo-guide/#investigation).
Keep in mind that excessive logging can impact performance, so it's best to remove or comment out these console.log statements in production code.
</assistant_answer>
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai how to filter with useOne
Let me check the knowledge base for you again. One moment! :books:
----------------------
To filter data with the
useOne
hook in refine, you need to understand that useOne
is primarily used to fetch a single record based on its ID. It doesn't have built-in filtering capabilities like useList
does. However, you can achieve filtering-like behavior by using the meta
property of useOne
. Here's how you can approach this:
1. Use the meta
property to pass additional parameters to your data provider:
2. In your data provider, you'll need to handle these additional parameters in the getOne
method:
This approach allows you to send additional filtering parameters to your API when fetching a single record useOne documentation (https://refine.dev/docs/data/hooks/use-one/#meta).
Remember that the actual implementation of filtering will depend on your backend API. You'll need to ensure that your API can handle these additional parameters and apply the filtering on the server side.
Also, keep in mind that useOne
is designed to fetch a single record by its ID. If you need more complex filtering or want to fetch multiple records based on certain criteria, you might want to consider using useList
or useMany
hooks instead, which are better suited for those use cases Data Hooks documentation (https://refine.dev/docs/guides-concepts/data-fetching/#data-hooks).
If you need more specific guidance, please provide more details about your exact use case and what kind of filtering you're trying to achieve.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai In my resource profeDatas I have id, userId, group and so on. Can I use useOne to retrieve the data but not using the id, use userId
Let me check the knowledge base for you again. One moment! :books:
----------------------
While
useOne
is primarily designed to fetch a single record using its id
, you can adapt it to use a different field like userId
for retrieval. However, this requires some customization in your data provider. Here's how you can approach this:
1. Use the meta
property in useOne
to pass the userId
:
2. Modify your data provider's getOne
method to handle this custom scenario:
This approach allows you to use useOne
with userId
instead of id
for the profeDatas
resource useOne documentation (https://refine.dev/docs/data/hooks/use-one/#meta).
Remember to adjust the API call in the data provider according to your backend's specific requirements. The key is to use the meta
object to pass additional information to your data provider.
Keep in mind that this is a custom solution and might not be ideal for all scenarios. If you frequently need to fetch records based on fields other than id
, you might want to consider creating a custom hook or using useList
with appropriate filters.
Data Provider documentation (https://refine.dev/docs/data/data-provider/#getone-)
If you need further assistance or have more specific requirements, please provide more details about your use case.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai useList or useTable to show only one record
Let me check the knowledge base for you again. One moment! :books:
----------------------
While both
useList
and useTable
are designed to fetch and display multiple records, you can adapt them to show only one record. However, for fetching a single record, useOne
is generally more appropriate. Let's look at how you can use each of these hooks to display a single record:
1. Using useOne
(recommended for single record):
This is the most straightforward and efficient way to fetch and display a single record useOne documentation (https://refine.dev/docs/data/hooks/use-one/).
2. Using useList
:
This approach uses pagination and filtering to fetch only one record useList documentation (https://refine.dev/docs/data/hooks/use-list/#pagination).
3. Using useTable
:
This is similar to useList
but provides additional functionality for tables useTable documentation (https://refine.dev/docs/data/hooks/use-table/#filtering).
While you can use useList
or useTable
to fetch a single record, it's generally more efficient and semantically correct to use useOne
for this purpose. useOne
is specifically designed to fetch a single record and will typically result in a more optimized API call.
If you have a specific reason for needing to use useList
or useTable
for a single record, the above examples show how you can achieve that. However, unless you have a particular requirement that necessitates using these hooks, useOne
is the recommended approach for fetching and displaying a single record.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
@kapa.ai How to filter by the latest date created
Let me check the knowledge base for you again. One moment! :books:
----------------------
To filter by the latest date created in refine, you can use the
useList
or useTable
hook with appropriate sorting and pagination options. Here's how you can achieve this:
1. Using useList
:
2. Using useTable
:
In both cases, we're doing the following:
1. Setting the sorters
option to sort by the createdAt
field in descending order (desc
).
2. Using pagination to fetch only the first page with one item.
This approach will fetch the most recently created record based on the createdAt
field useList documentation (https://refine.dev/docs/data/hooks/use-list/#sorting).
Remember that the actual field name (createdAt
in this example) should match the field name in your data model. If your creation date field has a different name, adjust the field
value in the sorters
array accordingly.
Also, ensure that your backend API supports sorting by this field. The exact implementation may vary depending on your data provider and backend setup.
If you need to display multiple records sorted by creation date, you can adjust the pageSize
in the pagination options to fetch more records while maintaining the sorting order.
useTable documentation (https://refine.dev/docs/data/hooks/use-table/#sorting)
</assistant_answer>
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1172098337760096258> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
vicious-goldOP•2mo ago
Filter to retrieve the latest date created