Enable Google Auth using Supabase, Refine, nextjs and material
The guides I am seeing say to make changes to src/App.tsx, but this does not exist in my Refine installation.
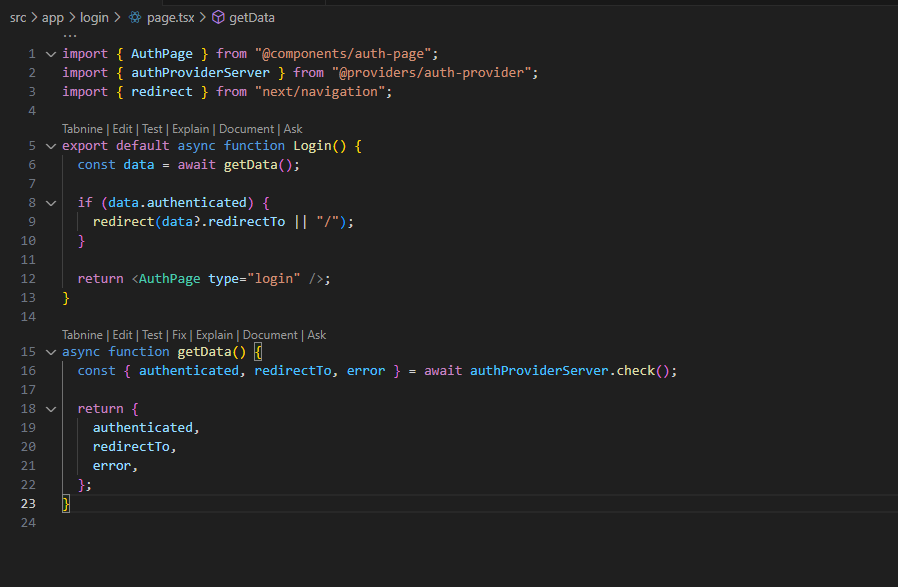
15 Replies
Hi @OhSoGamer. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
To enable Google Auth using Supabase with Refine, Next.js, and Material-UI, you'll need to follow a few steps. However, you're correct that the guides mentioning
src/App.tsx
may not be applicable to your specific Refine installation, especially if you're using Next.js.
For a Next.js-based Refine application, the setup is slightly different. Here's a general approach you can follow:
1. First, enable Google Auth on Supabase:
Enable Google Auth on Supabase (https://refine.dev/docs/data/packages/supabase/#enable-google-auth-on-supabase)
2. In your Next.js application, you'll need to set up the authentication provider. This is typically done in a file like pages/_app.tsx
or a custom App
component.
3. You'll need to create an auth provider that uses Supabase. This might be in a file like src/authProvider.ts
or similar.
4. In your main application file (which could be pages/_app.tsx
for Next.js), you'll need to set up the Refine component with the auth provider and other necessary configurations.
Here's a general idea of what your setup might look like:
5. For the login page, you can create a custom page (e.g., pages/login.tsx
) that uses the AuthPage
component from Refine:
Remember, the exact implementation might vary depending on your specific setup and versions of Refine and Next.js you're using.
For more detailed guidance, you might want to check out the Refine documentation on authentication (https://refine.dev/docs/authentication/auth-provider/) and Supabase authentication with Refine (https://refine.dev/blog/refine-pixels-3/#supabase-authentication-with-refine).
If you're still having trouble, you might want to check if there's a specific example or tutorial for Next.js with Refine and Supabase, as the setup can be slightly different from the standard React application.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@272101189942902795> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
extended-salmonOP•6mo ago
@kapa.ai when using the below, and hitting 'sign in with google', I get 'missing email or phone AuthApiError'
``
Let me check the knowledge base for you again. One moment! :books:
----------------------
I apologize, but I don't have enough context or code to fully understand the issue you're experiencing. You mentioned "using the below," but there's no code or configuration provided in your question.
However, based on the error message you're receiving ("missing email or phone AuthApiError"), it seems like there might be an issue with the Google authentication setup or the way the user data is being handled.
To properly diagnose and help with this issue, I would need more information, such as:
1. The relevant code snippets where you're setting up the Google authentication.
2. The configuration of your Supabase project, especially the Google OAuth settings.
3. The version of Refine, Supabase, and Next.js you're using.
Without this information, I can only provide general advice:
1. Make sure you've correctly set up Google OAuth in your Supabase project as described in the Supabase documentation (https://refine.dev/docs/data/packages/supabase/#enable-google-auth-on-supabase).
2. Check that your Google OAuth credentials (Client ID and Client Secret) are correctly configured in Supabase.
3. Ensure that you're requesting the necessary scopes from Google, including the user's email.
4. Review your
authProvider
implementation to make sure it's correctly handling the Google sign-in process.
If you can provide more details about your setup and the relevant code, I'd be happy to help you troubleshoot this issue further.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@272101189942902795> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
extended-salmonOP•6mo ago
@kapa.ai
src/app/login/page.tsx
import { AuthPage } from "@components/auth-page";
import { authProviderServer } from "@providers/auth-provider";
import { redirect } from "next/navigation";
export default async function Login() {
const data = await getData();
if (data.authenticated) {
redirect(data?.redirectTo || "/");
}
return <AuthPage type="login" providers={[
{
name: "google",
label: "Sign in with Google",
},
]} />;
}
async function getData() {
const { authenticated, redirectTo, error } = await authProviderServer.check();
return {
authenticated,
redirectTo,
error,
};
}
Let me check the knowledge base for you again. One moment! :books:
----------------------
Thank you for providing the code snippet. It appears you're using Refine with Next.js and trying to implement Google authentication. Let's analyze the code and provide some insights.
The code you've shared is for a login page in a Next.js application using Refine. Here's a breakdown of what's happening:
1. The
Login
component is an async function, which is a feature of Next.js 13+ for server components.
2. It uses an AuthPage
component from Refine, which is imported from a custom path @components/auth-page
. This suggests you might have a custom implementation of the AuthPage.
3. The authProviderServer
is imported from @providers/auth-provider
, which is likely your custom auth provider implementation for server-side operations.
4. The component checks if the user is already authenticated using the getData
function, which calls authProviderServer.check()
.
5. If the user is authenticated, it redirects them to the specified redirect URL or the home page.
6. If not authenticated, it renders the AuthPage
component with Google as a provider option.
This setup looks correct in general, but there are a few points to consider:
1. Make sure your AuthPage
component is correctly implemented to handle the Google sign-in process. The Refine documentation provides an example of how this should be set up: Social Logins (https://refine.dev/docs/data/packages/supabase/#social-logins)
2. Ensure that your authProviderServer
is correctly implemented. It should handle the server-side authentication checks and processes. The Refine documentation provides a detailed example of how an auth provider should be structured: Auth Provider Interface (https://refine.dev/docs/guides-concepts/authentication/auth-provider-interface/)
3. If you're still encountering the "missing email or phone AuthApiError", the issue might be in how the Google authentication is being processed after the user clicks the "Sign in with Google" button. This could be happening in your AuthPage
component or in the client-side part of your auth provider.
To resolve the "missing email or phone AuthApiError", you might need to:
1. Check your Supabase project settings to ensure Google OAuth is correctly configured.
2. Verify that your Google OAuth credentials are correct and that you're requesting the necessary scopes (including email).
3. Review the client-side implementation of your auth provider, especially the login
method, to ensure it's correctly handling the Google sign-in process.
If you're still facing issues, you might want to check the Refine Supabase auth provider example (https://refine.dev/blog/refine-pixels-2/#refine-s-authprovider-prop) for a complete implementation of the auth provider with Supabase.
Remember, the exact implementation might vary depending on your specific setup and versions of Refine, Next.js, and Supabase you're using. If you continue to face issues, you might need to provide more details about your setup or check the Refine and Supabase documentation for any recent changes or updates to the authentication process.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@272101189942902795> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
extended-salmonOP•6mo ago
If anyone can help this is what shows up when I hit sign in with google...
Supabase and google cloud are configured correctly
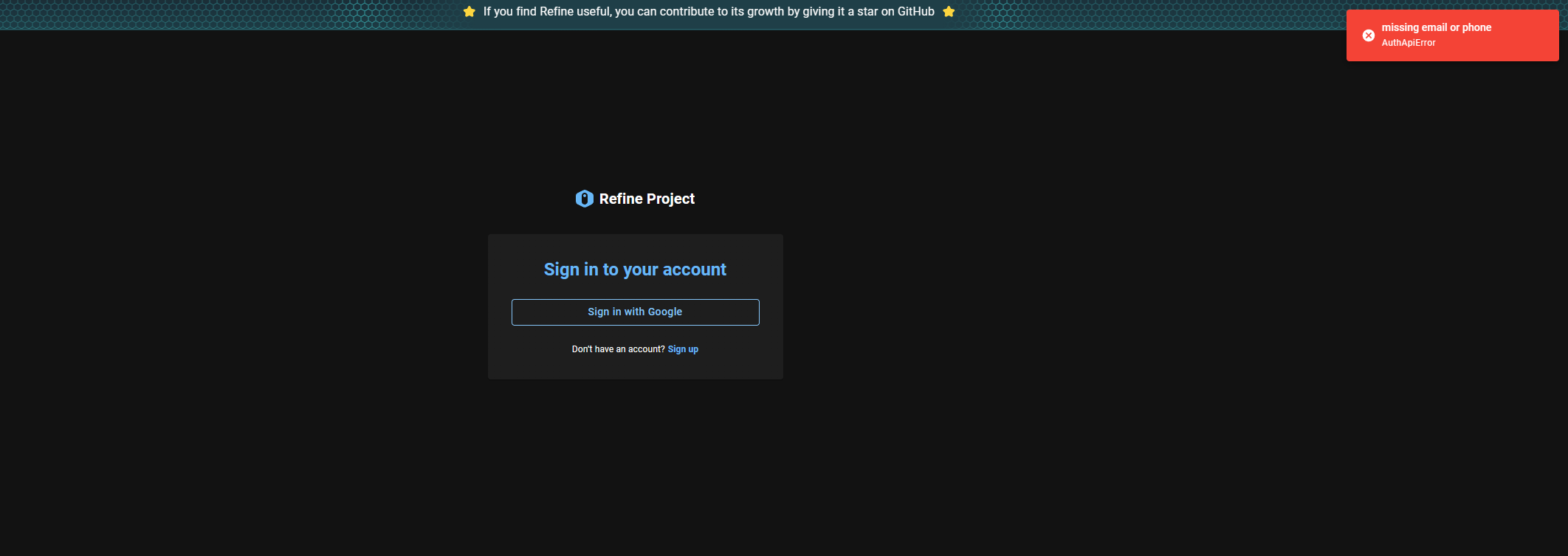
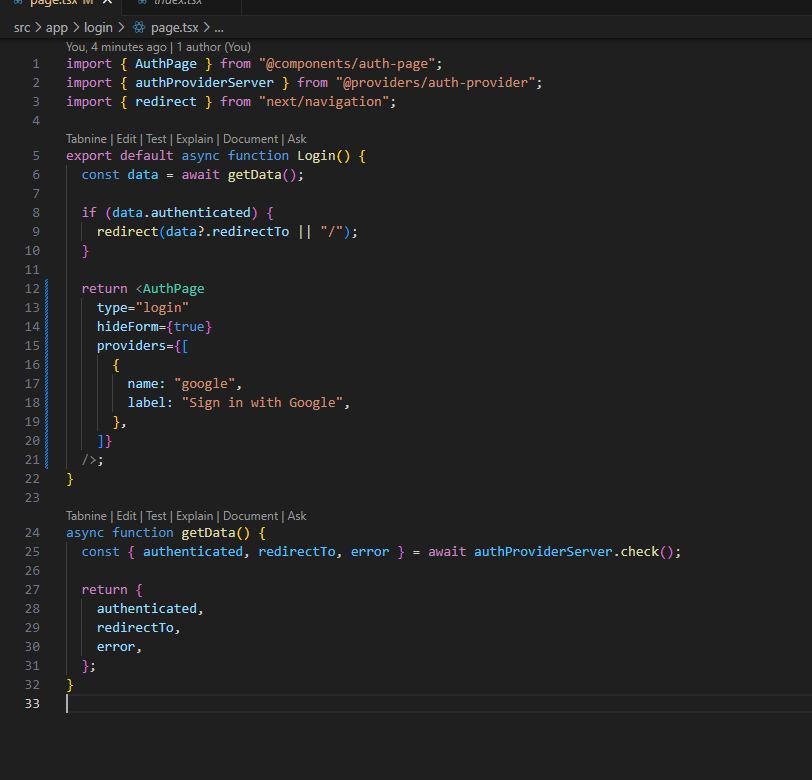
extended-salmonOP•6mo ago
@kapa.ai The google login popup doesn't appear, it is like the page is trying to login using an email
Let me check the knowledge base for you again. One moment! :books:
----------------------
Based on the information you've provided and the previous context, it seems that the Google login functionality is not being triggered correctly. Instead, it appears the page is defaulting to an email-based login. Let's troubleshoot this issue:
1. First, make sure that your
AuthPage
component is correctly set up to handle Google login. The providers
prop you're passing should trigger the Google OAuth flow. Here's an example of how it should look:
2. Check your authProvider
implementation. The login
method should handle different provider types, including Google. Here's a simplified example of how it might look:
3. Ensure that your Supabase project is correctly configured for Google OAuth. You can review the setup process in the Supabase documentation on enabling Google Auth (https://refine.dev/docs/data/packages/supabase/#enable-google-auth-on-supabase).
4. If you're using a custom AuthPage
component, make sure it's correctly implementing the Google login flow. The standard Refine AuthPage
component should handle this automatically when provided with the correct providers
prop.
5. Check your browser console for any errors that might provide more information about why the Google login isn't being triggered.
6. Verify that you're not accidentally overriding the Google login functionality somewhere in your custom implementation.
If you've confirmed all of the above and are still facing issues, you might want to review the Refine authentication documentation (https://refine.dev/docs/authentication/auth-provider/) for any recent changes or updates to the authentication process.
Remember, the exact implementation can vary depending on your specific setup and the versions of Refine, Next.js, and Supabase you're using. If you continue to face issues, you might need to provide more details about your authProvider
implementation and any custom AuthPage
component you might be using.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@272101189942902795> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
ratty-blush•3w ago
@kapa.ai How to register a user using this google oauth
Let me check the knowledge base for you again. One moment! :books:
----------------------
Based on the information provided in the knowledge sources, I'll explain how to register a user using Google OAuth with Refine and Supabase.
To implement Google OAuth for user registration in Refine, you need to:
1. Configure Google OAuth in Supabase:
First, enable Google Auth in your Supabase project by going to Authentication → Settings → Auth providers, and configure your Google credentials as mentioned in the Supabase documentation.
2. Set up the AuthPage component:
In your login page, use the
AuthPage
component with Google provider configuration:
3. Implement the authProvider:
The key part is implementing the login
method in your authProvider
to handle OAuth providers:
It's important to note that when using Google OAuth with Supabase, the authentication flow works differently than traditional registration. When a user signs in with Google for the first time, Supabase automatically creates a new user account if one doesn't exist. This means you don't need a separate registration flow for Google OAuth users.
Based on the issue you're experiencing where the Google login popup doesn't appear, there might be a problem with how the OAuth flow is being triggered. According to a GitHub issue, there can be issues with the asynchronous nature of the OAuth process. The popup should be triggered by the signInWithOAuth
method, and it should wait for user confirmation before proceeding.
Make sure your implementation correctly awaits the OAuth process and doesn't proceed prematurely. If you're still experiencing issues, you might want to check the updated Google Auth example that Refine provides, which has been updated to use the new Google Identity Service.
Remember that for proper OAuth implementation, you need to add both your deployed application URL and your local development URL to the list of allowed origins in your Google OAuth app settings.
Would you like more specific details on any part of this implementation?
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@347285614531444756> Kapa.ai is still learning and improving, please let me know how I did by reacting below.