edit useModalForm
is there a way to create an edit modalform and keep the list view on the back?
24 Replies
Hi @SetiZ. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
Yes, it is possible to create an edit modal form while keeping the list view in the background using
useModalForm
in refine. This approach allows you to edit a record without navigating away from the list view.
Here's how you can implement this:
1. Use the useModalForm
hook to manage the modal state and form logic.
2. Create a modal component that contains the edit form.
3. Add an edit button to your list view that opens the modal when clicked.
Here's an example of how you can implement this:
In this example:
1. We use useModalForm
to manage the modal and form state.
2. The Modal
component from Mantine is used to create the modal.
3. The edit form is placed inside the Modal
.
4. The List
component remains in the background.
5. An EditButton
is added to each record in the list, which opens the modal when clicked.
This approach allows you to edit records in a modal while keeping the list view visible in the background. The modal will open when the edit button is clicked, and the list view will remain unchanged in the background.
useModalForm Usage (https://refine.dev/docs/ui-integrations/mantine/hooks/use-modal-form/#usage)
Remember to adjust the code according to your specific needs and data structure.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@267689263246606336> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
xenial-blackOP•4mo ago
I wanted it in ant design
@kapa.ai I wanted it in ant design
Let me check the knowledge base for you again. One moment! :books:
----------------------
I apologize for the confusion. You're right, and I'll provide you with an example using Ant Design instead. Here's how you can create an edit modal form while keeping the list view in the background using
useModalForm
with Ant Design in refine:
In this example:
1. We use useModalForm
from @refinedev/antd
to manage the modal and form state.
2. The Modal
component from Ant Design is used to create the modal.
3. The edit form is placed inside the Modal
.
4. The List
component remains in the background, containing a Table
with the list of posts.
5. An EditButton
is added to each record in the table, which opens the modal when clicked.
This approach allows you to edit records in a modal while keeping the list view visible in the background. The modal will open when the edit button is clicked, and the list view will remain unchanged in the background.
useModalForm Usage with Ant Design (https://refine.dev/docs/ui-integrations/ant-design/hooks/use-modal-form/#usage)
Remember to adjust the code according to your specific needs and data structure.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@267689263246606336> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
xenial-blackOP•4mo ago
@kapa.ai should I create a /edt/id file ?
Let me check the knowledge base for you again. One moment! :books:
----------------------
Based on the information provided in the knowledge sources, you don't need to create a separate
/edit/id
file when using useModalForm
for editing records in a modal while keeping the list view in the background.
The useModalForm
hook is designed to handle the editing functionality within the same component as your list view. This approach allows you to manage both the list and the edit form in a single file, typically your list component.
Here's why you don't need a separate /edit/id
file:
1. Modal-based editing: The edit form is displayed in a modal, which is part of the same component as your list view useModalForm Usage (https://refine.dev/docs/ui-integrations/ant-design/hooks/use-modal-form/#usage).
2. In-place editing: The useModalForm
hook manages the state and logic for editing within the component where it's used, eliminating the need for a separate edit page useModalForm Usage (https://refine.dev/docs/ui-integrations/ant-design/hooks/use-modal-form/#usage).
3. Resource configuration: When setting up your resources in the <Refine>
component, you typically define the edit
property only if you're using a separate edit page. In this case, you wouldn't need to specify it Refine Usage (https://refine.dev/docs/ui-integrations/ant-design/introduction/#usage).
By using useModalForm
, you're implementing an inline editing approach that doesn't require navigation to a separate edit page. This keeps your application structure simpler and provides a more seamless user experience.
If you decide later that you want a separate edit page in addition to or instead of the modal form, then you would create an /edit/[id]
file and configure the edit
property in your resource definition.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@267689263246606336> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
xenial-blackOP•4mo ago
@kapa.ai can we have a modalform with /edit/id ? or only in new page?
Let me check the knowledge base for you again. One moment! :books:
----------------------
Yes, it is possible to have a modal form while still using the
/edit/id
route in refine. This approach combines the benefits of modal editing with the traditional routing structure. Here's how you can implement this:
1. Create an /edit/[id]
file for your edit page.
2. Use useModalForm
in this edit page component.
3. The modal will appear when navigating to the /edit/id
route, while still allowing you to keep the list view in the background.
Here's an example of how you can implement this using Ant Design:
In your list component, you would use the <EditButton>
component to navigate to this edit page:
This approach allows you to:
1. Use the /edit/id
route structure.
2. Display the edit form in a modal.
3. Keep the list view visible in the background when editing.
The useModalForm
hook automatically handles opening the modal when the component mounts, which happens when navigating to the /edit/id
route useModalForm Usage (https://refine.dev/docs/ui-integrations/ant-design/hooks/use-modal-form/#usage).
Remember to configure your resource in the <Refine>
component to use this edit page:
This setup gives you the flexibility of using both modal forms and traditional routing, allowing for a more versatile user interface.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@267689263246606336> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
xenial-blackOP•4mo ago
@kapa.ai but it's hiding the listview on the back, how can I make it still show?
Let me check the knowledge base for you again. One moment! :books:
----------------------
I apologize for the confusion in my previous response. You're right that the approach I described would hide the list view when navigating to the
/edit/id
route. To keep the list view visible in the background while still using the /edit/id
route, we need to take a different approach. Here's how you can achieve this:
1. Keep your list view as the main component.
2. Use the useModalForm
hook in the list component.
3. Use the useParams
hook from react-router-dom to check if there's an id
in the URL.
4. If there's an id
, show the modal automatically.
Here's an example of how you can implement this using Ant Design:
In this setup:
1. The list view remains as the main component, always visible.
2. We use useParams
to check if there's an id
in the URL.
3. If an id
is present, we automatically show the modal using the useEffect
hook.
4. When closing the modal, we navigate back to the list view URL.
You'll need to update your routes to make this work:
This approach allows you to:
1. Keep the list view always visible.
2. Use the /edit/id
route to open the edit modal.
3. Maintain a clean URL structure.
Remember to adjust the EditButton
in your table to use the correct route:
This solution combines the benefits of modal editing, URL-based navigation, and keeping the list view visible, providing a seamless user experience while maintaining a structured routing system useModalForm Usage (https://refine.dev/docs/ui-integrations/ant-design/hooks/use-modal-form/#usage).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@267689263246606336> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
xenial-blackOP•4mo ago
@kapa.ai rollback a bit. I need to do it with nextjs, /edit/id with modalform
Let me check the knowledge base for you again. One moment! :books:
----------------------
I apologize for the confusion earlier. Let's focus on implementing a modal form for editing in Next.js using the
/edit/[id]
route structure. Here's how you can achieve this with refine and Next.js:
1. Create an /edit/[id].tsx
file in your pages/posts
directory (assuming you're working with a "posts" resource).
2. In this file, use the useModalForm
hook to handle the modal and form logic.
3. Use Next.js's useRouter
to handle navigation.
Here's an example of how you can implement this:
In your pages/posts/index.tsx
file (list view), you can use the <EditButton>
component to navigate to the edit page:
This setup will:
1. Use the /posts/edit/[id]
route structure.
2. Display the edit form in a modal when navigating to this route.
3. Allow you to return to the list view by closing the modal.
Remember to configure your resource in the <Refine>
component:
This approach combines the benefits of Next.js routing with refine's useModalForm
hook, allowing you to have a modal edit form while maintaining clean URL structures useModalForm Usage (https://refine.dev/docs/ui-integrations/ant-design/hooks/use-modal-form/#usage).
Note that this method will navigate to the edit page, which means the list view won't be visible in the background. If you want to keep the list view visible, you'd need to implement a more complex solution involving layout components and state management.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@267689263246606336> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
xenial-blackOP•4mo ago
@kapa.ai it's still hiding elements on the back, I don't know what i am doing wrong
Let me check the knowledge base for you again. One moment! :books:
----------------------
I apologize for the confusion. You're right that the previous approaches I suggested are still hiding elements in the background, which is not what you want. Let's take a step back and address your specific need for a Next.js application with a modal form that keeps the list view visible in the background.
To achieve this, we need to modify our approach. Instead of creating a separate
/edit/[id]
page, we'll implement the modal form directly in the list view. This way, the list remains visible when editing a record. Here's how you can do this:
1. Keep your list view in pages/posts/index.tsx
.
2. Implement the modal form within this component.
3. Use Next.js routing to handle the URL changes.
Here's an example of how you can implement this:
In this setup:
1. The list view remains as the main component, always visible.
2. We use useModalForm
to handle the edit form logic.
3. We use Next.js's useRouter
to handle URL changes.
4. When an edit button is clicked, we update the URL with a query parameter (?id=...
) instead of navigating to a new page.
5. We use an useEffect
hook to show the modal when an id
is present in the URL.
6. When closing the modal, we remove the id
from the URL.
This approach allows you to:
1. Keep the list view always visible.
2. Use URL parameters to represent the edit state.
3. Maintain a clean URL structure.
4. Use the modal form for editing.
Remember to adjust your <Refine>
component configuration accordingly:
This solution should keep your list view visible in the background while editing a record in a modal form, addressing the issue you were facing useModalForm Usage (https://refine.dev/docs/ui-integrations/ant-design/hooks/use-modal-form/#usage).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@267689263246606336> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
conscious-sapphire•3mo ago
Hello @SetiZ, it's kinda complex task but Refine has example for that and actually our examples based on this: https://github.com/remix-run/react-router/tree/main/examples/modal-route-with-outlet
app-crm-minimal example:
List route: https://github.com/refinedev/refine/blob/master/examples/app-crm-minimal/src/App.tsx#L69
Renderin
<Outlet />
inside List component https://github.com/refinedev/refine/blob/master/examples/app-crm-minimal/src/routes/companies/list/index.tsx#L155
Route component as a modal https://github.com/refinedev/refine/blob/master/examples/app-crm-minimal/src/routes/companies/create/index.tsx#L7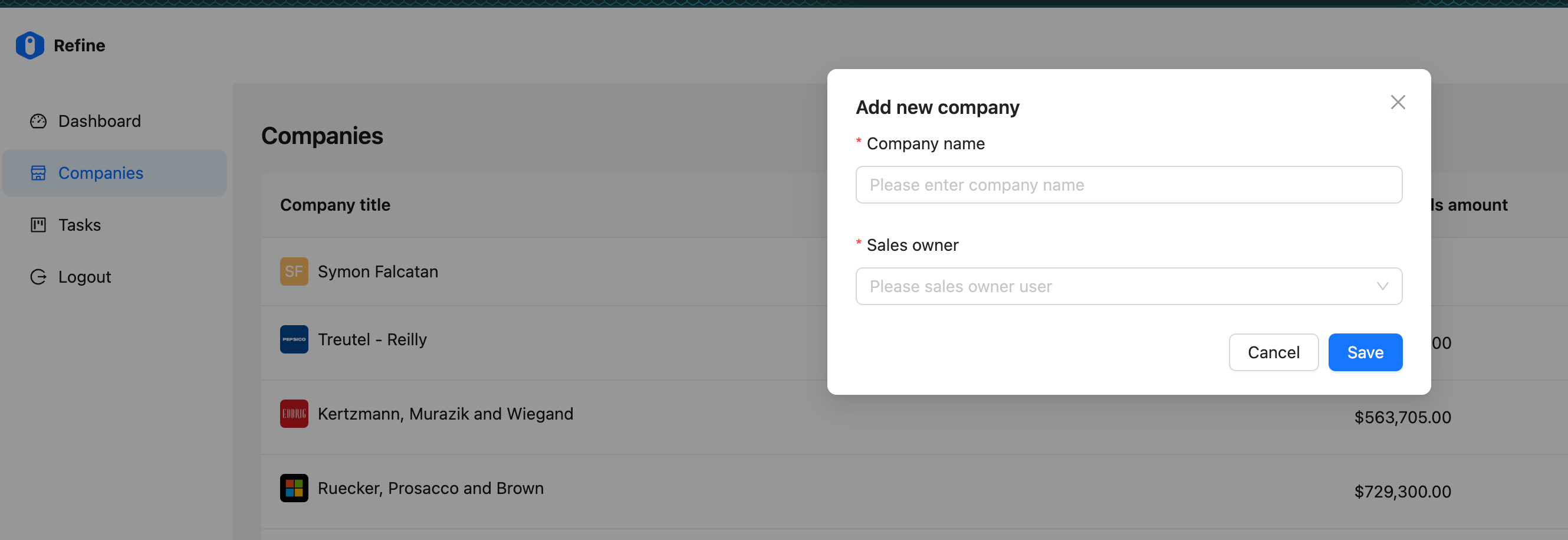
xenial-blackOP•3mo ago
Hello thank you for the answer
i was checking that app-crm-minimal and wanted to have similar behavior, I guess my problem here might reside on the fact that i am using nextjs
conscious-sapphire•3mo ago
I didn't tried to do that with nextjs and unfortunately my hands are full at the moment and I will not be able to implement this, but it will be in my mind, if I can find time and find a solution, I will publish it as an example in the repo
xenial-blackOP•3mo ago
no worries, i'll look into it!! thank you anway, i will recheck your exemples