modal
help me create a modal that presents itself in the centre of the page that takes up roughly 1/3 of the screen with sampel text , needs to have some type of elevation and background blur
10 Replies
Hi @flankieflew. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
To create a modal with the specifications you've described, we can use the Material UI Modal component along with some custom styling. Here's an example of how you can implement this:
This code creates a modal that meets your requirements:
1. It's centered on the page using
position: "absolute"
and transform: "translate(-50%, -50%)"
.
2. It takes up roughly 1/3 of the screen width with width: "33%"
.
3. It has elevation (box shadow) with boxShadow: 24
.
4. It has a background blur effect with backdropFilter: "blur(5px)"
.
5. It contains sample text in the form of a header and description.
You can adjust the styling as needed to fine-tune the appearance.
To use this modal, you would typically place it in your React component and trigger it with the handleOpen
function, which you can attach to a button or any other interactive element.
Source: How to use Material UI Modal (https://refine.dev/blog/material-ui-modal/)
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@343691288442306561> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
adverse-sapphireOP•5mo ago
@kapa.ai modify that so i can dynamically use it from another component
Let me check the knowledge base for you again. One moment! :books:
----------------------
Certainly! To make the modal component more reusable and allow it to be dynamically used from another component, we can modify it to accept props for controlling its state and content. Here's how you can refactor the previous example:
Now, you can use this
DynamicModal
component from another component like this:
In this refactored version:
1. The DynamicModal
component accepts props for open
, onClose
, title
, and content
.
2. The state management (open
state and handleOpen
/handleClose
functions) is moved to the parent component.
3. The parent component can now control when the modal opens and closes, and what content it displays.
This approach allows you to reuse the modal component across your application, dynamically setting its content and controlling its visibility from any other component.
Source: How to use Material UI Modal (https://refine.dev/blog/material-ui-modal/)
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@343691288442306561> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
like-gold•5mo ago
Hello @flankieflew ,
kapa.ai is now available for free at https://refine.dev/docs!
To improve our community interaction on the Refine Discord server and keep our focus on Q&A sharing, we invite you to our website to use kapa.ai. 🚀
adverse-sapphireOP•5mo ago
hi @Alican Erdurmaz
i get upon clicking
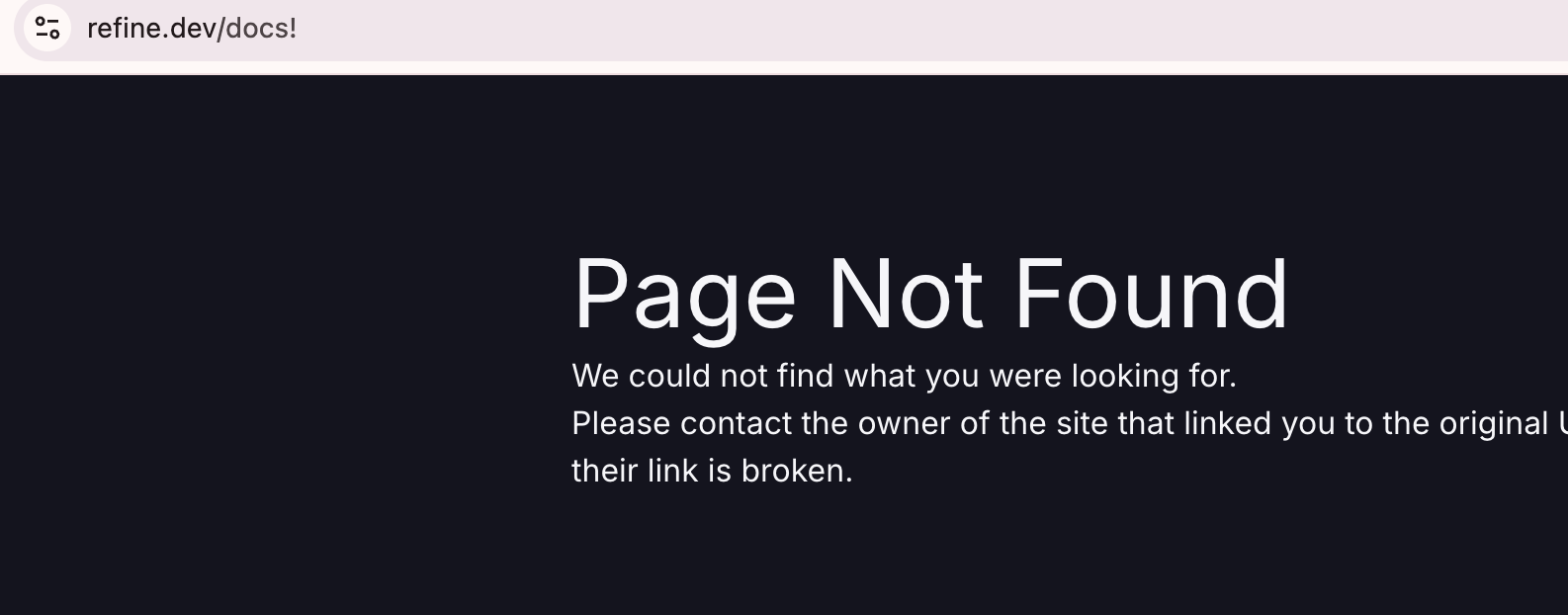
like-gold•5mo ago
sorry, wrong link. shouldn't have question mark 🫠 https://refine.dev/docs/
The AI button is at the bottom-right corner of the page.
Overview | Refine
What is Refine?
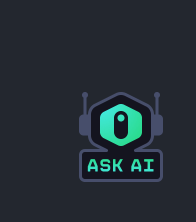
adverse-sapphireOP•5mo ago
adverse-sapphireOP•5mo ago
thanks! that worked!